In Java intellej Correct this code and add the following 1.Correct: (The error shows a problem under () next to "firstBeachShoot.printPhotoDetails") 2.Add the following to the second package (screenshot): 1.for or for...each or while loops. 2.Arrays 3.default, no-args and parameterized constructors. Here's the first package : import java.util.Scanner; public class Image { int numberOfPhotos; // photos on roll double fStop; // light let it 1.4,2.0,2.8 ... 16.0 int iso; // sensativity to light 100,200, 600 int filterNumber; // 1-6 String subjectMatter; String color; // black and white or color String location; boolean isblurry; public String looksBlurry(boolean key) { if (key == true) { return "Photo is Blurry"; } else { return "Photo is Clear"; } } public void printPhotoDetails(String s1) { Scanner br = new Scanner(System.in); String subjectMatter = s1; System.out.println("Data of Nature photos:"); System.out.println("Enter number of photos:"); numberOfPhotos = br.nextInt(); int i = 1; while (true) { System.out.println("Enter Filter number of photos" + i + ":"); filterNumber = br.nextInt(); System.out.println("Enter colour of photo" + i + ":"); String color = br.next(); System.out.println("Enter focal length of photo" + i + ":"); fStop = br.nextInt(); System.out.println("Enter location of photo" + i + ":"); String location = br.next(); System.out.println("Enter iso of photo" + i + ":"); String iso = br.next(); System.out.println("----------------------------------"); System.out.println("Printing details of current photo:"); System.out.println("----------------------------------"); System.out.println("This is a/an " + subjectMatter + "roll:" + " " + color + "using filter: " + filterNumber + "."); System.out.println("It has " + numberOfPhotos + "photos with " + fStop + "fStop."); System.out.println("This photo was taken near the" + location + "with film at " + iso + "ISO" + "."); if (isblurry == true) { System.out.println("You can post it."); continue; } else { System.out.println("You should post it."); } break; } } public static void main(String[] args) { int ch; Image object = new Image(); Scanner brake = new Scanner(System.in); System.out.println("Photo subject"); System.out.println("1. Nature photos\n2.Flower photos"); try { System.out.println("Enter choice:"); ch = brake.nextInt(); switch (ch) { case 1: String a = "BeachShoot"; object.printPhotoDetails(a); break; case 2: String b = "City Shoot"; object.printPhotoDetails(b); break; } } catch (NumberFormatException ex) { System.out.println("catch block executed..."); } brake.close(); } }
In Java intellej
Correct this code and add the following
1.Correct:
(The error shows a problem under () next to "firstBeachShoot.printPhotoDetails")
2.Add the following to the second package (screenshot):
1.for or for...each or while loops.
2.Arrays
3.default, no-args and parameterized constructors.
Here's the first package :
import java.util.Scanner;
public class Image {
int numberOfPhotos; // photos on roll
double fStop; // light let it 1.4,2.0,2.8 ... 16.0
int iso; // sensativity to light 100,200, 600
int filterNumber; // 1-6
String subjectMatter;
String color; // black and white or color
String location;
boolean isblurry;
public String looksBlurry(boolean key) {
if (key == true) {
return "Photo is Blurry";
} else {
return "Photo is Clear";
}
}
public void printPhotoDetails(String s1) {
Scanner br = new Scanner(System.in);
String subjectMatter = s1;
System.out.println("Data of Nature photos:");
System.out.println("Enter number of photos:");
numberOfPhotos = br.nextInt();
int i = 1;
while (true) {
System.out.println("Enter Filter number of photos" + i + ":");
filterNumber = br.nextInt();
System.out.println("Enter colour of photo" + i + ":");
String color = br.next();
System.out.println("Enter focal length of photo" + i + ":");
fStop = br.nextInt();
System.out.println("Enter location of photo" + i + ":");
String location = br.next();
System.out.println("Enter iso of photo" + i + ":");
String iso = br.next();
System.out.println("----------------------------------");
System.out.println("Printing details of current photo:");
System.out.println("----------------------------------");
System.out.println("This is a/an " + subjectMatter + "roll:" + " " + color + "using filter: " + filterNumber + ".");
System.out.println("It has " + numberOfPhotos + "photos with " + fStop + "fStop.");
System.out.println("This photo was taken near the" + location + "with film at " + iso + "ISO" + ".");
if (isblurry == true) {
System.out.println("You can post it.");
continue;
} else {
System.out.println("You should post it.");
}
break;
}
}
public static void main(String[] args) {
int ch;
Image object = new Image();
Scanner brake = new Scanner(System.in);
System.out.println("Photo subject");
System.out.println("1. Nature photos\n2.Flower photos");
try {
System.out.println("Enter choice:");
ch = brake.nextInt();
switch (ch) {
case 1:
String a = "BeachShoot";
object.printPhotoDetails(a);
break;
case 2:
String b = "City Shoot";
object.printPhotoDetails(b);
break;
}
} catch (NumberFormatException ex) {
System.out.println("catch block executed...");
}
brake.close();
}
}
![public static void main(String[] args) {
Image firstBeachShoot = new Image();
firstBeachShoot.number0fPhotos = 36;
firstBeachShoot.fStop
2.8;
firstBeachShoot.iso = 400;
firstBeachShoot.location = "beach";
firstBeachShoot.isblurry
false;
firstBeachShoot.color = "black and white";
firstBeachShoot.subjectMatter = "ocean";
firstBeachShoot.filterNumber =
6;
firstBeachShoot.printPhotoDetails();
System.out.println();
Image firstCityShoot = new Image();
firstCityShoot.number0fPhotos = 35;
firstCityShoot.fStop
= 1.2;
firstCityShoot.iso = 100;
firstCityShoot.location = "city";
firstCityShoot.isblurry =
false;
firstCityShoot.color = "in color";
firstCityShoot.subjectMatter = "skyscraper";
: 5;
firstCityShoot.printPhotoDetails();
firstCityShoot.filterNumber
%3D](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e13e78d-a8e8-451b-8b31-688accdac576%2Fe084a3ce-8b0f-41af-b00d-8d67e11d18c2%2Fyuhs99_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

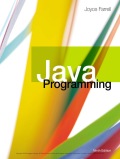
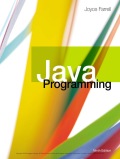