JAVA Language Caesar Shift Question: Modify the Caesar class so that it will allow various sized shifts to be used, instead of just a shift of size 3. (Hint: Use an instance variable in the Caesar class to represent the shift, add a constructor to set it, and change the encode method to use it.) import java.util.*; public class TestCipher { public static void main(String[] args) { int shift = 7; Caesar caesar = new Caesar(); String text = "hello world"; String encryptTxt = caesar.encrypt(text); System.out.println(text + " encrypted with shift " + shift + " is " + encryptTxt); } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new StringBuffer(""); // Use a StringBuffer StringTokenizer words = new StringTokenizer(s); // Break s into its words while (words.hasMoreTokens()) { // For each word in s result.append(encode(words.nextToken()) + " "); // Encode it } return result.toString(); // Return the result } // encrypt() public String decrypt(String s) { StringBuffer result = new StringBuffer(""); // Use a StringBuffer StringTokenizer words = new StringTokenizer(s); // Break s into words while (words.hasMoreTokens()) { // For each word in s result.append(decode(words.nextToken()) + " "); // Decode it } return result.toString(); // Return the decryption } // decrypt() public abstract String encode(String word); // Abstract methods public abstract String decode(String word); } // Cipher class Caesar extends Cipher { public String encode(String word) { StringBuffer result = new StringBuffer(); // Initialize a string buffer for (int k = 0; k < word.length(); k++) { // For each character in word char ch = word.charAt(k); // Get the character ch = (char)('a' + (ch -'a'+ 3) % 26); // Perform caesar shift result.append(ch); // Append it to new string } return result.toString(); // Return the result as a string } // encode() public String decode(String word) { StringBuffer result = new StringBuffer(); // Initialize a string buffer for (int k = 0; k < word.length(); k++) { // For each character in word char ch = word.charAt(k); // Get the character ch = (char)('a' + (ch - 'a' + 23) % 26); // Perform reverse shift result.append(ch); // Append it to new string } return result.toString(); // Return the result as a string } // decode() } // Caesar
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
JAVA Language
Caesar Shift
Question:
import java.util.*;
public class TestCipher {
public static void main(String[] args) {
int shift = 7;
Caesar caesar = new Caesar();
String text = "hello world";
String encryptTxt = caesar.encrypt(text);
System.out.println(text + " encrypted with shift " + shift + " is " + encryptTxt);
}
}
abstract class Cipher {
public String encrypt(String s) {
StringBuffer result = new StringBuffer(""); // Use a StringBuffer
StringTokenizer words = new StringTokenizer(s); // Break s into its words
while (words.hasMoreTokens()) { // For each word in s
result.append(encode(words.nextToken()) + " "); // Encode it
}
return result.toString(); // Return the result
} // encrypt()
public String decrypt(String s) {
StringBuffer result = new StringBuffer(""); // Use a StringBuffer
StringTokenizer words = new StringTokenizer(s); // Break s into words
while (words.hasMoreTokens()) { // For each word in s
result.append(decode(words.nextToken()) + " "); // Decode it
}
return result.toString(); // Return the decryption
} // decrypt()
public abstract String encode(String word); // Abstract methods
public abstract String decode(String word);
} // Cipher
class Caesar extends Cipher {
public String encode(String word) {
StringBuffer result = new StringBuffer(); // Initialize a string buffer
for (int k = 0; k < word.length(); k++) { // For each character in word
char ch = word.charAt(k); // Get the character
ch = (char)('a' + (ch -'a'+ 3) % 26); // Perform caesar shift
result.append(ch); // Append it to new string
}
return result.toString(); // Return the result as a string
} // encode()
public String decode(String word) {
StringBuffer result = new StringBuffer(); // Initialize a string buffer
for (int k = 0; k < word.length(); k++) { // For each character in word
char ch = word.charAt(k); // Get the character
ch = (char)('a' + (ch - 'a' + 23) % 26); // Perform reverse shift
result.append(ch); // Append it to new string
}
return result.toString(); // Return the result as a string
} // decode()
} // Caesar

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

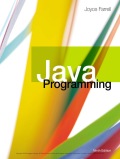
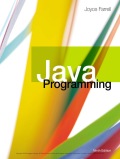