IN JAVA When people enter their telephone numbers into a form they use many different formats. Some examples are: (444) 999-1234 4449991234 444.999.1234 In the PhoneNumbers class below, complete the cleanNumber method. It takes a string and extracts the numeric digits from the string. If there are exactly 10 numeric digits, the number is reformatted: (###) ###-#### and returned. If there are any other number of digits, the string "Error" is returned. Hint: Use the Character.isDigit method to check whether a character is a digit. public class PhoneNumbers { /** Cleans a phone number. @param phoneNumber a phone number that should contain ten digits and possibly other characters @return the phone number in the form (###) ###-#### or the string "Error" if phoneNumber does not have ten digits */ public String cleanNumber(String phoneNumber) { // your work here ... for (int i = 0; i < ...; i++) { char ch = phoneNumber.charAt(i); if (Character.isDigit(ch)) { ... } } ... } }
IN JAVA
When people enter their telephone numbers into a form they use many different formats. Some examples are: (444) 999-1234 4449991234 444.999.1234 In the PhoneNumbers class below, complete the cleanNumber method. It takes a string and extracts the numeric digits from the string. If there are exactly 10 numeric digits, the number is reformatted: (###) ###-#### and returned. If there are any other number of digits, the string "Error" is returned. Hint: Use the Character.isDigit method to check whether a character is a digit.
public class PhoneNumbers
{
/**
Cleans a phone number.
@param phoneNumber a phone number that should contain ten digits and possibly other characters
@return the phone number in the form (###) ###-#### or the string "Error" if phoneNumber
does not have ten digits
*/
public String cleanNumber(String phoneNumber)
{
// your work here
...
for (int i = 0; i < ...; i++)
{
char ch = phoneNumber.charAt(i);
if (Character.isDigit(ch))
{
...
}
}
...
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

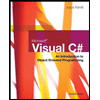
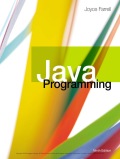
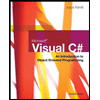
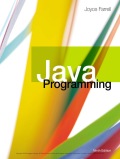