in java you are required to build a mini PetCare application for the pet owners to keep track of their pets growth in a virtual world. Follow the given steps 1. Build a class Pet having the following data members a. Name (String) b. Gender (char) c. Type (String) d. Age (int) e. Weight (float) f. healthCondition (int) Imagine this class Pet represents your pet as a virtual object. Provide constructors with arguments for name, gender, type, age and weight. Initialize healthCondition to 5 (which means healthy). Provide getter for each of these but setter for only weight. Provide a function eat(String food). This function represents the action of eating that would increase the weight of the pet according to following rules Food Increase In Weight Red Meat 0.14 kg Chicken 0.12 kg Pet Food 0.17 kg Supplement 0.1 kg Fish 0.09 kg The eat function returns the new Weight of the pet object. Provide another function fallSick() that reduces the healthCondition of the pet 1 but it cannot go below 0. Provide a function bool isDead() this function returns true if the healthCondition of a pet is 0 and false otherwise. Add another function recover(). This function increases the healthCondition by 1 and returns true. But if the healthCondition had been 0 this function will not do anything and return false. 0 value in healthCondition means the pet is dead. You should check this in the eat function also. If a pet is dead, the eat function wont increase weight. Add another function grow(int days). This function adds the days to the age of the pet and returns true. However, if the age of the pet reaches 100 or above then the pet would die. This will be done by setting the healthCondition to 0. If the pet is dead, the grow function will return false. Provide the toString method which returns a formatted string showing the details of this pet. 2. Build a class Person with the following data members/instance variables a. Name b. Pet [] pets; This class represents a person who owns one or more pets. The pets array is an aggregation of the Pet object in Person Class. You will provide a constructor taking the name of the person as argument and the number of pets he/she has. Initialize the name and instantiate the array to the size provided in the constructor. Provide a method addPet(Pet p) which adds this pet object p to the array. You might want to add more instance variables to this class to help you in manipulating the array (Seek help from banking app). Provide a method feedPet(String petName, String food). This method searches the pet with the given name in the array and then call its eat method with the given food. Provide a method getPet(String name) which searches for the pet with given name in the array and then returns it. If it is not found, then null should be returned 3. Write main method to test each part of your code.
in java you are required to build a mini PetCare application for the pet owners to keep track of their
pets growth in a virtual world. Follow the given steps
1. Build a class Pet having the following data members
a. Name (String)
b. Gender (char)
c. Type (String)
d. Age (int)
e. Weight (float)
f. healthCondition (int)
Imagine this class Pet represents your pet as a virtual
object. Provide constructors with arguments for name,
gender, type, age and weight. Initialize healthCondition to 5
(which means healthy).
Provide getter for each of these but setter for only weight.
Provide a function eat(String food). This function represents the action of eating that
would increase the weight of the pet according to following rules
Food Increase In Weight
Red Meat 0.14 kg
Chicken 0.12 kg
Pet Food 0.17 kg
Supplement 0.1 kg
Fish 0.09 kg
The eat function returns the new Weight of the pet object.
Provide another function fallSick() that reduces the healthCondition of the pet 1 but it cannot go
below 0.
Provide a function bool isDead() this function returns true if the healthCondition of a pet is 0
and false otherwise.
Add another function recover(). This function increases the healthCondition by 1 and returns
true. But if the healthCondition had been 0 this function will not do anything and return false. 0
value in healthCondition means the pet is dead. You should check this in the eat function also. If
a pet is dead, the eat function wont increase weight.
Add another function grow(int days). This function adds the days to the age of the pet and
returns true. However, if the age of the pet reaches 100 or above then the pet would die. This
will be done by setting the healthCondition to 0. If the pet is dead, the grow function will return
false.
Provide the toString method which returns a formatted string showing the details of this pet.
2. Build a class Person with the following data members/instance variables
a. Name
b. Pet [] pets;
This class represents a person who owns one or more pets. The pets array is an aggregation of the Pet
object in Person Class.
You will provide a constructor taking the name of the person as argument and the number of
pets he/she has. Initialize the name and instantiate the array to the size provided in the
constructor.
Provide a method addPet(Pet p) which adds this pet object p to the array. You might want to
add more instance variables to this class to help you in manipulating the array (Seek help from
banking app).
Provide a method feedPet(String petName, String food). This method searches the pet with the
given name in the array and then call its eat method with the given food.
Provide a method getPet(String name) which searches for the pet with given name in the array
and then returns it. If it is not found, then null should be returned
3. Write main method to test each part of your code.

Step by step
Solved in 4 steps

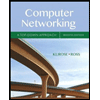
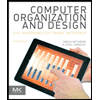
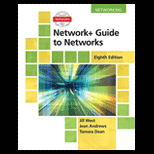
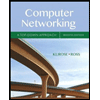
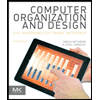
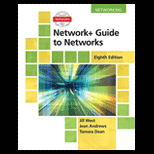
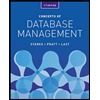
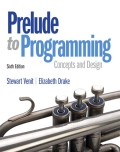
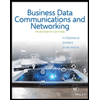