In Python 3 I have the working code below it just needs 5 functions. NO import pickle! Write a program that keeps names and email addresses in a dictionary as key-value pairs. You Must create and use at least 5 meaningful functions. A function to display a menu A function to look up a person’s email address A function to add a new name and email address A function to change an email address A function to delete a name and email address. This is an working code but it needs 5 functions. #Dictionary Dict = {} while(True): #Menu print("\nMenu") print("----------------------------------------") print("1. Look up an email address") print("2. Add a new name and email address") print("3. Change an existing email address") print("4. Delete a name and email address") print("5. Quit the program") print() #prompt for choice choice = int(input("Enter your choice: ")) #if choice is 1 if choice == 1: #prompt for name name = input("Enter a name: ") #if name in dictionary if name in Dict: #prints name and email print("Name: " + name) print("Email: " + Dict[name]) #if name not in dictionary else: print("The specified name was not found.") #if choice is 2 elif choice == 2: #prompt for name name = input("Enter name: ") #if name in dictionary if name in Dict: print("The specified name was exist") #if name not in dictionary else: #prompt for email email = input("Enter email address: ") #adding item into dictionary Dict[name] = email print("Name and address have been added.") #if choice is 3 elif choice == 3: #prompt for name name = input("Enter a name: ") #if name in dictionary if name in Dict: #prompt for email email = input("Enter the new address: ") #updating item in dictionary Dict[name] = email print("Information updated.") #if name not in dictionary else: print("The specified name was not found.") #if choice is 4 elif choice == 4: #prompt for name name = input("Enter a name: ") #if name in dictionary if name in Dict: #deleting item from dictionary del Dict[name] print("Information deleted.") #if name not in dictionary else: print("The specified name was not found.") #if choice is 5 elif choice == 5: print("Information saved.") #exiting program break #if choice is wrong else: print("Wrong Choice! Try Again...") This is the Required Output example: Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Sam The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Jack Name: Jack Email: Jack@yahoo.com Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 3 Enter name: John Enter the new address: John@wayne.edu Information updated Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: John Name: John Email: John@wayne.edu Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 4 Enter name: Sam The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 4 Enter name: Jack Information deleted Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Jack The specified name was not found Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 5 Information saved >>> Upvote for correct fix. Thank you.
In Python 3
I have the working code below it just needs 5 functions.
NO import pickle!
Write a
You Must create and use at least 5 meaningful functions.
- A function to display a menu
- A function to look up a person’s email address
- A function to add a new name and email address
- A function to change an email address
- A function to delete a name and email address.
This is an working code but it needs 5 functions.
#Dictionary
Dict = {}
while(True):
#Menu
print("\nMenu")
print("----------------------------------------")
print("1. Look up an email address")
print("2. Add a new name and email address")
print("3. Change an existing email address")
print("4. Delete a name and email address")
print("5. Quit the program")
print()
#prompt for choice
choice = int(input("Enter your choice: "))
#if choice is 1
if choice == 1:
#prompt for name
name = input("Enter a name: ")
#if name in dictionary
if name in Dict:
#prints name and email
print("Name: " + name)
print("Email: " + Dict[name])
#if name not in dictionary
else:
print("The specified name was not found.")
#if choice is 2
elif choice == 2:
#prompt for name
name = input("Enter name: ")
#if name in dictionary
if name in Dict:
print("The specified name was exist")
#if name not in dictionary
else:
#prompt for email
email = input("Enter email address: ")
#adding item into dictionary
Dict[name] = email
print("Name and address have been added.")
#if choice is 3
elif choice == 3:
#prompt for name
name = input("Enter a name: ")
#if name in dictionary
if name in Dict:
#prompt for email
email = input("Enter the new address: ")
#updating item in dictionary
Dict[name] = email
print("Information updated.")
#if name not in dictionary
else:
print("The specified name was not found.")
#if choice is 4
elif choice == 4:
#prompt for name
name = input("Enter a name: ")
#if name in dictionary
if name in Dict:
#deleting item from dictionary
del Dict[name]
print("Information deleted.")
#if name not in dictionary
else:
print("The specified name was not found.")
#if choice is 5
elif choice == 5:
print("Information saved.")
#exiting program
break
#if choice is wrong
else:
print("Wrong Choice! Try Again...")
This is the Required Output example:
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Sam The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Jack Name: Jack Email: Jack@yahoo.com
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 3 Enter name: John Enter the new address: John@wayne.edu Information updated
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: John Name: John Email: John@wayne.edu
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 4 Enter name: Sam The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 4 Enter name: Jack Information deleted
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 1 Enter a name: Jack The specified name was not found
Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program
Enter your choice: 5 Information saved >>> |
Upvote for correct fix. Thank you.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

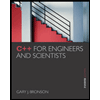
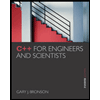