In python please get_count(aList, item) (1.5 points) Returns the number of times item appears in aList. You cannot make any assumptions about the contents of the list. You are not allowed to use any count methods from the Python library. Input list aList A list of elements of any type many item An object of any type Output int Recurrence of item in aList >>> get_count([1,4,3.5,'1',3.5, 9, 1, 4, 2], 1) 2 >>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 3.5) 2 >>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 9) 1 >>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 'a') 0 replace(numList, old, new) (1.5 points) Returns a copy of numList with all the occurrences of old replaced by new. The process should not mutate the original list (alter the memory block referenced by numList). You can assume numList is a list of numbers and that old and new are either int or float. You are not allowed to use any replace methods from the Python library. Input list numList A list of numbers int/float old A number int/float new A number Output list Copy of numList with all the occurrences of old replaced by new >>> input_list = [1, 7, 5.6, 3, 2, 4, 1, 9] >>> replace(input_list, 1, 99.9) [99.9, 7, 5.6, 3, 2, 4, 99.9, 9] >>> input_list [1, 7, 5.6, 3, 2, 4, 1, 9] >>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 5.6, 777) [1, 7, 777, 3, 2, 4, 1, 9] >>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 8, 99) [1, 7, 5.6, 3, 2, 4, 1, 9] cut(aList) (3 points) Takes a list of integers and returns a list identical to aList, but when a negative number appears, the function deletes the negative number and the next x-1 elements, where x is the absolute value of the negative number. The function should not mutate aList. Input list aList A list that contains only integers Output list A list identical to aList where we delete a number of elements from that sequence >>> cut([7, 4, -2, 1, 9]) # Found -2: Delete -2 and 1 [7, 4, 9] >>> cut([-4, -7, -2, 1, 9]) # Found -4: Delete -4, -7, -2 and 1 [9] neighbor(n) (4 points) Takes a positive integer and returns an integer where neighboring digits of the same value are removed. Converting n to other types such as str is not allowed. As described in Homework 1, floor division (//) and modulo (%) operators are useful here. You are not allowed to use the math module. Input int n A positive integer Output int Integer with neighboring duplicates removed >>> neighbor(2222466666678) 24678 >>> neighbor(2222466666625) # Note that the last 2 is not removed 24625
In python please get_count(aList, item) (1.5 points) Returns the number of times item appears in aList. You cannot make any assumptions about the contents of the list. You are not allowed to use any count methods from the Python library. Input list aList A list of elements of any type many item An object of any type Output int Recurrence of item in aList >>> get_count([1,4,3.5,'1',3.5, 9, 1, 4, 2], 1) 2 >>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 3.5) 2 >>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 9) 1 >>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 'a') 0 replace(numList, old, new) (1.5 points) Returns a copy of numList with all the occurrences of old replaced by new. The process should not mutate the original list (alter the memory block referenced by numList). You can assume numList is a list of numbers and that old and new are either int or float. You are not allowed to use any replace methods from the Python library. Input list numList A list of numbers int/float old A number int/float new A number Output list Copy of numList with all the occurrences of old replaced by new >>> input_list = [1, 7, 5.6, 3, 2, 4, 1, 9] >>> replace(input_list, 1, 99.9) [99.9, 7, 5.6, 3, 2, 4, 99.9, 9] >>> input_list [1, 7, 5.6, 3, 2, 4, 1, 9] >>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 5.6, 777) [1, 7, 777, 3, 2, 4, 1, 9] >>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 8, 99) [1, 7, 5.6, 3, 2, 4, 1, 9] cut(aList) (3 points) Takes a list of integers and returns a list identical to aList, but when a negative number appears, the function deletes the negative number and the next x-1 elements, where x is the absolute value of the negative number. The function should not mutate aList. Input list aList A list that contains only integers Output list A list identical to aList where we delete a number of elements from that sequence >>> cut([7, 4, -2, 1, 9]) # Found -2: Delete -2 and 1 [7, 4, 9] >>> cut([-4, -7, -2, 1, 9]) # Found -4: Delete -4, -7, -2 and 1 [9] neighbor(n) (4 points) Takes a positive integer and returns an integer where neighboring digits of the same value are removed. Converting n to other types such as str is not allowed. As described in Homework 1, floor division (//) and modulo (%) operators are useful here. You are not allowed to use the math module. Input int n A positive integer Output int Integer with neighboring duplicates removed >>> neighbor(2222466666678) 24678 >>> neighbor(2222466666625) # Note that the last 2 is not removed 24625
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 33SA
Related questions
Question
In python please
get_count(aList, item) (1.5 points)
Returns the number of times item appears in aList. You cannot make any assumptions about the
contents of the list. You are not allowed to use any count methods from the Python library.
Input
list aList A list of elements of any type
many item An object of any type
Output
int Recurrence of item in aList
>>> get_count([1,4,3.5,'1',3.5, 9, 1, 4, 2], 1)
2
>>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 3.5)
2
>>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 9)
1
>>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 'a')
0
replace(numList, old, new) (1.5 points)
Returns a copy of numList with all the occurrences of old replaced by new. The process should not
mutate the original list (alter the memory block referenced by numList). You can assume numList
is a list of numbers and that old and new are either int or float. You are not allowed to use any
replace methods from the Python library.
Input
list numList A list of numbers
int/float old A number
int/float new A number
Output
list Copy of numList with all the occurrences of old replaced by new
>>> input_list = [1, 7, 5.6, 3, 2, 4, 1, 9]
>>> replace(input_list, 1, 99.9)
[99.9, 7, 5.6, 3, 2, 4, 99.9, 9]
>>> input_list
[1, 7, 5.6, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 5.6, 777)
[1, 7, 777, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 8, 99)
[1, 7, 5.6, 3, 2, 4, 1, 9]
Returns the number of times item appears in aList. You cannot make any assumptions about the
contents of the list. You are not allowed to use any count methods from the Python library.
Input
list aList A list of elements of any type
many item An object of any type
Output
int Recurrence of item in aList
>>> get_count([1,4,3.5,'1',3.5, 9, 1, 4, 2], 1)
2
>>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 3.5)
2
>>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 9)
1
>>> get_count([1,4,3.5,'1',3.5, 9, 4, 2], 'a')
0
replace(numList, old, new) (1.5 points)
Returns a copy of numList with all the occurrences of old replaced by new. The process should not
mutate the original list (alter the memory block referenced by numList). You can assume numList
is a list of numbers and that old and new are either int or float. You are not allowed to use any
replace methods from the Python library.
Input
list numList A list of numbers
int/float old A number
int/float new A number
Output
list Copy of numList with all the occurrences of old replaced by new
>>> input_list = [1, 7, 5.6, 3, 2, 4, 1, 9]
>>> replace(input_list, 1, 99.9)
[99.9, 7, 5.6, 3, 2, 4, 99.9, 9]
>>> input_list
[1, 7, 5.6, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 5.6, 777)
[1, 7, 777, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 8, 99)
[1, 7, 5.6, 3, 2, 4, 1, 9]
cut(aList) (3 points)
Takes a list of integers and returns a list identical to aList, but when a negative number appears,
the function deletes the negative number and the next x-1 elements, where x is the absolute value
of the negative number. The function should not mutate aList.
Input
list aList A list that contains only integers
Output
list A list identical to aList where we delete a number of elements from that sequence
>>> cut([7, 4, -2, 1, 9]) # Found -2: Delete -2 and 1
[7, 4, 9]
>>> cut([-4, -7, -2, 1, 9]) # Found -4: Delete -4, -7, -2 and 1
[9]
neighbor(n) (4 points)
Takes a positive integer and returns an integer where neighboring digits of the same value are
removed. Converting n to other types such as str is not allowed. As described in Homework 1,
floor division (//) and modulo (%) operators are useful here. You are not allowed to use the math
module.
Input
int n A positive integer
Output
int Integer with neighboring duplicates removed
>>> neighbor(2222466666678)
24678
>>> neighbor(2222466666625) # Note that the last 2 is not removed
24625
Takes a list of integers and returns a list identical to aList, but when a negative number appears,
the function deletes the negative number and the next x-1 elements, where x is the absolute value
of the negative number. The function should not mutate aList.
Input
list aList A list that contains only integers
Output
list A list identical to aList where we delete a number of elements from that sequence
>>> cut([7, 4, -2, 1, 9]) # Found -2: Delete -2 and 1
[7, 4, 9]
>>> cut([-4, -7, -2, 1, 9]) # Found -4: Delete -4, -7, -2 and 1
[9]
neighbor(n) (4 points)
Takes a positive integer and returns an integer where neighboring digits of the same value are
removed. Converting n to other types such as str is not allowed. As described in Homework 1,
floor division (//) and modulo (%) operators are useful here. You are not allowed to use the math
module.
Input
int n A positive integer
Output
int Integer with neighboring duplicates removed
>>> neighbor(2222466666678)
24678
>>> neighbor(2222466666625) # Note that the last 2 is not removed
24625
![(3 points)
Takes a list of integers and returns a list identical to aList, but when a negative number appears,
cut(aList)
the function deletes the negative number and the next x-1 elements, where x is the absolute value
of the negative number. The function should not mutate aList.
Input
aList A list that contains only integers
list
Output
list
A list identical to aList where we delete a number of elements from that sequence
>>> cut([7, 4, -2, 1, 9])
Found -2: Delete -2 and 1
[7, 4, 9]
>>> cut([-4, -7, -2, 1, 9]) # Found -4: Delete -4, -7, -2 and 1
[9]
(4 points)
Takes a positive integer and returns an integer where neighboring digits of the same value are
removed. Converting n to other types such as str is not allowed. As described in Homework 1,
floor division (//) and modulo (%) operators are useful here. You are not allowed to use the math
neighbor(n)
module.
Input
int
A positive integer
Output
int Integer with neighboring duplicates removed
>>> neighbor(2222466666678)
24678
>>> neighbor(2222466666625) # Note that the last 2 is not removed
24625](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F76de5ad5-a780-4d7f-a00a-85f0792f2226%2Fdc35b58b-ba6d-4bd3-afcc-2dee98ee91b0%2F4vjpgij_processed.jpeg&w=3840&q=75)
Transcribed Image Text:(3 points)
Takes a list of integers and returns a list identical to aList, but when a negative number appears,
cut(aList)
the function deletes the negative number and the next x-1 elements, where x is the absolute value
of the negative number. The function should not mutate aList.
Input
aList A list that contains only integers
list
Output
list
A list identical to aList where we delete a number of elements from that sequence
>>> cut([7, 4, -2, 1, 9])
Found -2: Delete -2 and 1
[7, 4, 9]
>>> cut([-4, -7, -2, 1, 9]) # Found -4: Delete -4, -7, -2 and 1
[9]
(4 points)
Takes a positive integer and returns an integer where neighboring digits of the same value are
removed. Converting n to other types such as str is not allowed. As described in Homework 1,
floor division (//) and modulo (%) operators are useful here. You are not allowed to use the math
neighbor(n)
module.
Input
int
A positive integer
Output
int Integer with neighboring duplicates removed
>>> neighbor(2222466666678)
24678
>>> neighbor(2222466666625) # Note that the last 2 is not removed
24625
![(1.5 points)
get_count(aList, item)
Returns the number of times item appears in aList. You cannot make any assumptions about the
contents of the list. You are not allowed to use any count methods from the Python library.
Input
A list of elements of any type
An object of any type
list
aList
many item
Output
int
Recurrence of item in aList
>>> get_count([1,4,3.5, '1',3.5, 9, 1, 4, 2], 1)
>>> get_count([1,4,3.5, '1',3.5, 9, 4, 2], 3.5)
>>> get_count([1,4,3.5, '1',3.5, 9, 4, 2], 9)
1
>>> get_count([1,4,3.5, '1',3.5, 9, 4, 2], 'a')
(1.5 points)
Returns a copy of numList with all the occurrences of old replaced by new. The process should not
mutate the original list (alter the memory block referenced by numList). You can assume numList
is a list of numbers and that old and new are either int or float. You are not allowed to use any
replace(numList, old, new)
replace methods from the Python library.
Input
numList A list of numbers
A number
list
int/float old
int/float new
A number
Output
list
Copy of numList with all the occurrences of old replaced by new
>>> input_list
>>> replace(input_list, 1, 99.9)
[99.9, 7, 5.6, 3, 2, 4, 99.9, 9]
>>> input_list
[1, 7, 5.6, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 5.6, 777)
[1, 7, 777, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 8, 99)
[1, 7, 5.6, 3, 2, 4, 1, 9]
[1, 7, 5.6, 3, 2, 4, 1, 9]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F76de5ad5-a780-4d7f-a00a-85f0792f2226%2Fdc35b58b-ba6d-4bd3-afcc-2dee98ee91b0%2Fz8a46s3_processed.jpeg&w=3840&q=75)
Transcribed Image Text:(1.5 points)
get_count(aList, item)
Returns the number of times item appears in aList. You cannot make any assumptions about the
contents of the list. You are not allowed to use any count methods from the Python library.
Input
A list of elements of any type
An object of any type
list
aList
many item
Output
int
Recurrence of item in aList
>>> get_count([1,4,3.5, '1',3.5, 9, 1, 4, 2], 1)
>>> get_count([1,4,3.5, '1',3.5, 9, 4, 2], 3.5)
>>> get_count([1,4,3.5, '1',3.5, 9, 4, 2], 9)
1
>>> get_count([1,4,3.5, '1',3.5, 9, 4, 2], 'a')
(1.5 points)
Returns a copy of numList with all the occurrences of old replaced by new. The process should not
mutate the original list (alter the memory block referenced by numList). You can assume numList
is a list of numbers and that old and new are either int or float. You are not allowed to use any
replace(numList, old, new)
replace methods from the Python library.
Input
numList A list of numbers
A number
list
int/float old
int/float new
A number
Output
list
Copy of numList with all the occurrences of old replaced by new
>>> input_list
>>> replace(input_list, 1, 99.9)
[99.9, 7, 5.6, 3, 2, 4, 99.9, 9]
>>> input_list
[1, 7, 5.6, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 5.6, 777)
[1, 7, 777, 3, 2, 4, 1, 9]
>>> replace([1,7, 5.6, 3, 2, 4, 1, 9], 8, 99)
[1, 7, 5.6, 3, 2, 4, 1, 9]
[1, 7, 5.6, 3, 2, 4, 1, 9]
Expert Solution

Step 1
Note: i have provide screenshot and code below for your reference and follow them for no error. thanks...
Code run succefully.
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
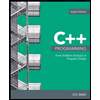
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
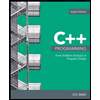
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning