In the array-based stack project uploaded to the BIO system, you are required to create a demo class called ArrayStackDemo.java to add the following static methods: A generic static method named removeTopNthStackElement( ) that receives two parameters a generic stack and an element of generic type. The method should remove the top Nth element from stack,
In the array-based stack project uploaded to the BIO system, you are required to create a demo class called ArrayStackDemo.java to add the following static methods:
A generic static method named removeTopNthStackElement( ) that receives two parameters a generic stack and an element of generic type. The method should remove the top Nth element from stack, if possible.

Actually, array is a collection of elements.
Code:
package com.okasha;
import java.util.Stack;
public class ArrayStackDemo {
public static void main(String[] args){
Stack<Integer> s1=new Stack<Integer>();
Stack<Integer> s2=new Stack<Integer>();
s1.push(10);
s1.push(15);
s1.push(20);
s1.push(25);
s2.push(5);
s2.push(10);
s2.push(12);
s2.push(14);
s2.push(20);
// calling the methos by passing
// both stack as parameters
int distincsValue=countStackDistinct(s1, s2 );
// Creating an iterator
System.out.println(" the distinct number of elements in the two stacks s1 and s2 is :"+distincsValue);
}
private static int countStackDistinct(Stack<Integer> s1, Stack<Integer> s2) {
// below variable store the all common elements counts in
// both stack s1, s2
int count=0;
// counting the total number of elements in both
// stack s1, s2
int total=s1.size()+s2.size();
// this loop pick one by one element from stack s1
for(Integer val: s1) {
// if val is exist in s2 stack
// common =ture
boolean common=false;
// checking above val value is common in s2 stack or not
// if common then increase count value by 1
for(int i=0; i<s2.size(); i++) {
if(val ==s2.get(i)) {
common=true;
break;
}
}
// if val common in both stack
// increase count value by 1
if(common) {
count++;
}
}
// same like above
// we iterate s2 elements one by one
for(Integer val: s2) {
boolean common=false;
for(int i=0; i<s1.size(); i++) {
if(val ==s1.get(i)) {
common=true;
break;
}
}
if(common) {
count++;
}
}
return total-count;// return the totla- common elements in both stack s1, s2
}
}
Step by step
Solved in 3 steps with 2 images

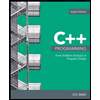
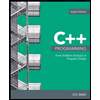