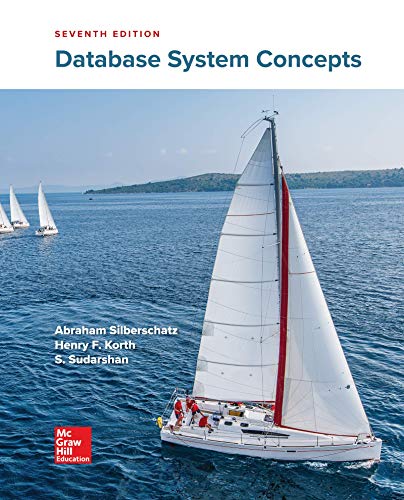
Concept explainers
In C++
Stuck and could use help with coding a loop to find the array location of each source node inside the file input loop in main(). Use get_value() to compare a node’s value to source_name and If the node does not exist, add it to the array using the set_value() function and incrementing n. Save index.
Comment out the output lines in the file input loop in main().
Sample Run
Enter file name: Data.txt
Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Note that Saturday is not shown
Node.h
// Node Declarations
#define ERR -1
#define NODE_MAX 20
#define EDGE_MAX 4
// Node class
class node
{ public:
node(); // Constructor
void set_value(string); // Set string value
string get_value(); // Return string value
void connect(node *); // Connect this node to another
void put(ostream &); // Output node and neighbors
private:
string value; // Node value
node *edge[EDGE_MAX]; // Edges array
};
Node.cpp
#include
#include
using namespace std;
#include "Node.h"
/******************************
* Null constructor
******************************/
node::node()
{ int i;
value = "";
for(i=0;i
edge[i] = NULL;
}
/******************************
* set_value()
******************************/
void node::set_value(string arg)
{ value = arg;
}
/******************************
* get_value()
******************************/
string node::get_value()
{ return value;
}
Main.cpp
#include
#include
#include
#include
using namespace std;
#include "Node.h"
/******************************
* main()
******************************/
void main()
{ int i,n;
int source_index,target_index,distance;
string fname,source_name,target_name;
fstream in;
node map[NODE_MAX];
// Initialize
n = 0;
cout << left;
// Get file name
cout << "Enter file name: ";
cin >> fname;
// Open file
in.open(fname,ios::in);
// Loop through file
while(!in.eof())
{ in >> source_name >> target_name >> distance;
// Add to array
if(in.good())
{ cout << setw(12) << source_name;
cout << setw(12) << target_name;
cout << setw(4) << distance;
cout << endl;
};
};
// Close file
in.close();
// Display array
for(i=0;i
cout << map[i].get_value() << endl;
}
Data.txt
Sunday Monday 10
Sunday Tuesday 20
Monday Thursday 30
Tuesday Friday 30
Wednesday Monday 20
Wednesday Tuesday 10
Thursday Sunday 50
Friday Sunday 60
Thursday Wednesday 10
Friday Wednesday 20
Thursday Saturday 20
Friday Saturday 10

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In this lab, we will practice the use of arrays. We will write a program that follows the steps below: Input 6 numbers from the user user inputs numbers one at a time Loop continuously until the exit condition below: ask the user to search for a query number if the query number is in the list: reports the first location of the query number in the list, change the number in that position to 0, show the updated list if the query number is not in the list: exit the loop You must write at least one new function: findAndReplace(array1,...,query) – take in an array and query number as input (in addition to any other needed inputs); it will return the first location of the query number in the list (or -1 if it is not in the list) and will change the number at that location to 0. You may receive the number inputs and print all outputs in int main if you wish. You may report location based on 0-indexing or 1-indexing (but you need 0-indexing to access the array elements!).arrow_forwardC++ Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. Assume also the last names and first names do not contain whitespaces. The program performs the following tasks: Read the file name of the tsv file from the user. Open the tsv file and read the student information. Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: A: 90 =< x B: 80 =< x < 90 C: 70 =< x < 80 D: 60 =< x < 70 F: x < 60 Compute the average of each exam. Output the last names, first names, exam scores, and letter grades of the…arrow_forwardQuestion R .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forward
- Python please: Given the input file input1.csv write a program that first reads in the name of the input file and then reads the file using the csv.reader() method. The file contains a list of words separated by commas. Your program should output the words in a sorted list. The contents of the input1.csv are: hello,cat,man,hey,dog,boy,Hello,man,cat,woman,dog,Cat,hey,boy Example: If the input is input1.csv the output is: ['Cat', 'Hello', 'boy', 'boy', 'cat', 'cat', 'dog', 'dog', 'hello', 'hey', 'hey', 'man', 'man', 'woman'] Note: There is a newline at the end of the output.arrow_forwardC programming language Topic : Input/Output Program : Product list (product.c) Definition : The program reads a series of items form a file and displays the data in columns. The program obtains the file name from the command line. Each line of the file will have the following form: item, mm-dd-yyyy, price For example, suppose that the file contains the following lines: 123, 12.00, 12/25/2006 124, 18.30, 1/10/2020 Expected output: Item Unit Purchase Price Date 123 $ 12.00 12/25/2006 124 $ 18.30 1/10/2020arrow_forwardC++ Code dynamicarray.h and dynamicarray.cpparrow_forward
- In python don't import librariesarrow_forwardIn python please! I am strugglingarrow_forwardWrite a function that reads all the numbers from a file into an array. The file is already open and there is no bad data in the file. The data is one number per line and an unknown number of lines of data. The function should read data until either end of file is detected or the array is full. Prototype void readData( ifstream& iFile, double numbers[ ], int size, int& count); iFile - already open ifstream numbers[ ] - array to store data size - the size of the array. count - the number of values read and must be set by the function before returning. HTML EditorKeyboard Shortcutsarrow_forward
- Computer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardPut the following code in a main.py file and a module.py file. Define the names sort, list_max and sum_of_list after putting the code into a main file and a module file #predefined modules import random import math #function to sort the list in ascending order def sort(x): #predefined function sort() x.sort() #print the sorted list print("\nSorted list is: ",str(x)) #function to find the sum of list elements def sum_of_list(x): #predefined function sum() Sum=sum(x) #return the sum of list elements return Sum #function to list the maximum from the list def list_max(x): #predefined function max() maximum=max(x) #return maximum return maximum #function to test the above three function def main(): #set a flag variable flag=True #create a list list1=list() #initialize the list element by using randrange() predefined function of random module list1=[random.randrange(1, 50, 1) for i in range(0,7)] #print the…arrow_forwardHow do I do this using pythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
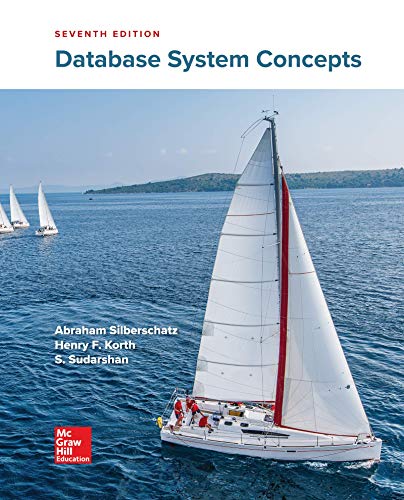
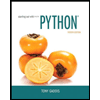
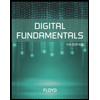
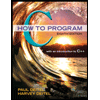
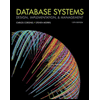
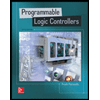