In the code template below, you are given two classes: Point, which represents to a point in 2D space, and Line, which represents a set of collinear points. To get the distance between two points, we just use the Euclidean distance formula for 2D: d = V(#2 – #1)² + (y2 – y1)² The slope of a line given two points (x1.yı) and (x.y:) , is given by this formula: Y2 – Y1 m = To check if a point (x1.y1) lies in a line with slope m, we can just use the slope-intercept form given by: y - Y1 = m(x – 2) The first two Point objects in the points attribute of a Line instance would dictate its slope and intercept. Afterwards, an instance of your Line class should be able to add collinear points to points. You cannot remove the first two points in the points attribute of a Line instance. This is to retain its slope and intercept. Your tasks are: a. To complete the other methods in the Point and Line classes. b. To create instances of the Point class and create an instance of the Line class whose points contain are instances of the Point class that are collinear with each other. Input Format The first line contains an integer n, the number of lines to follow. The next n lines contain the 2D coordinate values of each point, with the following format: Constraints For simplicity, assume that all x and y values in Point objects are integers. Assume that there would be no inputs that would result to lines with an infinite slope. Assume that the input number n will always be greater than 2. Output Format We can override the_str_method in the Point class such that it retums the 2D coordinates in the fomat: (х.у). We can override the str method in the Line class such that it retums a string containing all the collinear points in the line. Sample Input: e, e 1, 1 3, -3 Sample Output: Instatiating a Line: [(0, 0), (1, 1)] Adding More Points: ((0, 0), (1, 1)] Adding Redundant Points: [(0, 0), (1, 1)] Trying to Remove Last Input Point: (6, 0), (1, 1)] Trying to Remove All Points: [(6, 0), (1, 1)]
In the code template below, you are given two classes: Point, which represents to a point in 2D space, and Line, which represents a set of collinear points. To get the distance between two points, we just use the Euclidean distance formula for 2D: d = V(#2 – #1)² + (y2 – y1)² The slope of a line given two points (x1.yı) and (x.y:) , is given by this formula: Y2 – Y1 m = To check if a point (x1.y1) lies in a line with slope m, we can just use the slope-intercept form given by: y - Y1 = m(x – 2) The first two Point objects in the points attribute of a Line instance would dictate its slope and intercept. Afterwards, an instance of your Line class should be able to add collinear points to points. You cannot remove the first two points in the points attribute of a Line instance. This is to retain its slope and intercept. Your tasks are: a. To complete the other methods in the Point and Line classes. b. To create instances of the Point class and create an instance of the Line class whose points contain are instances of the Point class that are collinear with each other. Input Format The first line contains an integer n, the number of lines to follow. The next n lines contain the 2D coordinate values of each point, with the following format: Constraints For simplicity, assume that all x and y values in Point objects are integers. Assume that there would be no inputs that would result to lines with an infinite slope. Assume that the input number n will always be greater than 2. Output Format We can override the_str_method in the Point class such that it retums the 2D coordinates in the fomat: (х.у). We can override the str method in the Line class such that it retums a string containing all the collinear points in the line. Sample Input: e, e 1, 1 3, -3 Sample Output: Instatiating a Line: [(0, 0), (1, 1)] Adding More Points: ((0, 0), (1, 1)] Adding Redundant Points: [(0, 0), (1, 1)] Trying to Remove Last Input Point: (6, 0), (1, 1)] Trying to Remove All Points: [(6, 0), (1, 1)]
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Code Template:
class Point:
def _init_(self, x, y):
self.x = x
self.y = y
def
_str_(self):
" Returns a string showing the coordinates with the format (x,y) "--
# -- YOUR CODE HERE --
def
_eq__(self, other):
" Checks if two points pertain to the same coordinates ""
# -- YOUR CODE HERE --
def get_distance(self, other):
" Calculates the distance between two points """
# -- YOUR CODE HERE .-
class Line:
def _init_(self, point_a, point_b):
self.points = (point_a, point_b]
def_str_(self):
" Returns a string containing all collinear points in the Line instance ""
# -- YOUR CODE HERE --
def get_slope_intercept(self):
Calculates the slope of the line """
# -- YOUR CODE HERE --
def add_point(self, points):
"u" Adds a list of collinear points to the Line instance """
# -- YOUR CODE HERE --
24
25
def remove_point(self, points):
" Removes a list of points from the Line instance """
36
37
# -- YOUR CODE HERE --
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
if
== "
main
":
name
Tist_points = []
n = int(input())
for x in range(n):
input_line = input().split(',')
# -- YOUR CODE HERE
# Instantiate a Line using the first two input points
myLine =
# If your methods are defined correctly, the following lines should
#produce the desired output in the test cases.
print("Instatiating a Line: ")
print(myLine)
myLine.add_points(list_points[2:-1])
print("Adding More Points: ")
print(myLine)
myLine.add_points(list_points)
print("Adding Redundant Points: ")
print(myLine)
print("Trying to Remove Last Input Point:")
myLine.remove_points([list_points[-1]])
print(myLine)
print("Trying to Remove All Points:")
myLine.remove_points(list_points)
print(myLine)
64](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3cf655d-3bdc-4013-8c1f-5d6d56ea7228%2F2053b176-cbf6-48fc-b12e-fe04b664d031%2Fi73dqws_processed.png&w=3840&q=75)
Transcribed Image Text:Code Template:
class Point:
def _init_(self, x, y):
self.x = x
self.y = y
def
_str_(self):
" Returns a string showing the coordinates with the format (x,y) "--
# -- YOUR CODE HERE --
def
_eq__(self, other):
" Checks if two points pertain to the same coordinates ""
# -- YOUR CODE HERE --
def get_distance(self, other):
" Calculates the distance between two points """
# -- YOUR CODE HERE .-
class Line:
def _init_(self, point_a, point_b):
self.points = (point_a, point_b]
def_str_(self):
" Returns a string containing all collinear points in the Line instance ""
# -- YOUR CODE HERE --
def get_slope_intercept(self):
Calculates the slope of the line """
# -- YOUR CODE HERE --
def add_point(self, points):
"u" Adds a list of collinear points to the Line instance """
# -- YOUR CODE HERE --
24
25
def remove_point(self, points):
" Removes a list of points from the Line instance """
36
37
# -- YOUR CODE HERE --
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
if
== "
main
":
name
Tist_points = []
n = int(input())
for x in range(n):
input_line = input().split(',')
# -- YOUR CODE HERE
# Instantiate a Line using the first two input points
myLine =
# If your methods are defined correctly, the following lines should
#produce the desired output in the test cases.
print("Instatiating a Line: ")
print(myLine)
myLine.add_points(list_points[2:-1])
print("Adding More Points: ")
print(myLine)
myLine.add_points(list_points)
print("Adding Redundant Points: ")
print(myLine)
print("Trying to Remove Last Input Point:")
myLine.remove_points([list_points[-1]])
print(myLine)
print("Trying to Remove All Points:")
myLine.remove_points(list_points)
print(myLine)
64
![In the code template below, you are given two classes: Point, which represents to a point in 2D space, and Line,
which represents a set of collinear points.
To get the distance between two points, we just use the Euclidean distance formula for 2D:
d = V(22 - 21)² + (y2 – Y1)²
The slope of a line given two points (x1.yı) and (x.y:) , is given by this formula:
Y2 - Y1
m =
x2 - x1
To check if a point (x1.y1) lies in a line with slope m, we can just use the slope-intercept form given by:
y – Yı = m(x – x1)
The first two Point objects in the points attribute of a Line instance would dictate its slope and intercept.
Afterwards, an instance of your Line class should be able to add collinear points to points.
You cannot remove the first two points in the points attribute of a Line instance. This is to retain its slope and
intercept.
Your tasks are:
a. To complete the other methods in the Point and Line classes.
b. To create instances of the Point class and create an instance of the Line class whose points contain are
instances of the Point class that are collinear with each other.
Input Format
The first line contains an integer n, the number of lines to follow.
The next n lines contain the 2D coordinate values of each point, with the following format:
<x>,<y>
Constraints
For simplicity, assume that all x and y values in Point objects are integers.
Assume that there would be no inputs that would result to lines with an infinite slope.
Assume that the input number n will always be greater than 2.
Output Format
We can override the_str_method in the Point class such that it retums the 2D coordinates in the format:
(х.у).
We can override the_str_method in the Line class such that it retums a string containing all the collinear
points in the line.
Sample Input:
e, e
|1, 1
3, -3
Sample Output:
Instatiating a Line:
[(0, 0), (1, 1)]
Adding More Points:
((0, 0), (1, 1)]
Adding Redundant Points:
[(0, 0), (1, 1)]
Trying to Remove Last Input Point:
[(6, 6), (1, 1)]
Trying to Remove All Points:
[(6, ē), (1, 1)]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3cf655d-3bdc-4013-8c1f-5d6d56ea7228%2F2053b176-cbf6-48fc-b12e-fe04b664d031%2Fhl5pmp_processed.png&w=3840&q=75)
Transcribed Image Text:In the code template below, you are given two classes: Point, which represents to a point in 2D space, and Line,
which represents a set of collinear points.
To get the distance between two points, we just use the Euclidean distance formula for 2D:
d = V(22 - 21)² + (y2 – Y1)²
The slope of a line given two points (x1.yı) and (x.y:) , is given by this formula:
Y2 - Y1
m =
x2 - x1
To check if a point (x1.y1) lies in a line with slope m, we can just use the slope-intercept form given by:
y – Yı = m(x – x1)
The first two Point objects in the points attribute of a Line instance would dictate its slope and intercept.
Afterwards, an instance of your Line class should be able to add collinear points to points.
You cannot remove the first two points in the points attribute of a Line instance. This is to retain its slope and
intercept.
Your tasks are:
a. To complete the other methods in the Point and Line classes.
b. To create instances of the Point class and create an instance of the Line class whose points contain are
instances of the Point class that are collinear with each other.
Input Format
The first line contains an integer n, the number of lines to follow.
The next n lines contain the 2D coordinate values of each point, with the following format:
<x>,<y>
Constraints
For simplicity, assume that all x and y values in Point objects are integers.
Assume that there would be no inputs that would result to lines with an infinite slope.
Assume that the input number n will always be greater than 2.
Output Format
We can override the_str_method in the Point class such that it retums the 2D coordinates in the format:
(х.у).
We can override the_str_method in the Line class such that it retums a string containing all the collinear
points in the line.
Sample Input:
e, e
|1, 1
3, -3
Sample Output:
Instatiating a Line:
[(0, 0), (1, 1)]
Adding More Points:
((0, 0), (1, 1)]
Adding Redundant Points:
[(0, 0), (1, 1)]
Trying to Remove Last Input Point:
[(6, 6), (1, 1)]
Trying to Remove All Points:
[(6, ē), (1, 1)]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
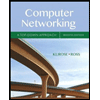
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
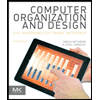
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
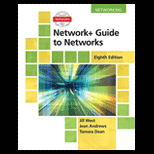
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
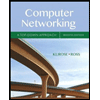
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
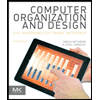
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
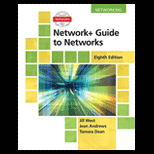
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
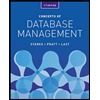
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
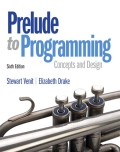
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
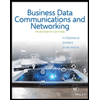
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY