In the following there are two classes, Employee and Manager. Manager is a subclass of Employee. The implementation of Employee class is given (Note: no getter methods are provided, so you are not able to access instance variables through getter methods in the subclass). The skeleton of the Manager class definition is given. Complete the constructor method, and the toString method for the subclass (see the details below). public class Employee { private String name; private double salary; // Make an employee with a given name and salary. public Employee(String aName, double aSalary) { name = aName; salary = aSalary; } // Provide a string description of an employee. public String toString() { return "Name: " + name + "\tSalary: " + salary; } } public class Manager extends Employee { private String department; // Make a manager with a given name, salary, and department. public Manager(String name, double salary, String department) { // put your code below } // Provide a string description of a manager. public String toString() { // put your code below } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In the following there are two classes, Employee and Manager. Manager is a subclass of Employee. The implementation of Employee class is given (Note: no getter methods are provided, so you are not able to access instance variables through getter methods in the subclass). The skeleton of the Manager class definition is given. Complete the constructor method, and the toString method for the subclass (see the details below).
public class Employee {
private String name;
private double salary;
// Make an employee with a given name and salary.
public Employee(String aName, double aSalary) {
name = aName;
salary = aSalary;
}
// Provide a string description of an employee.
public String toString() {
return "Name: " + name + "\tSalary: " + salary;
}
}
public class Manager extends Employee {
private String department;
// Make a manager with a given name, salary, and department.
public Manager(String name, double salary, String department)
{ // put your code below
}
// Provide a string description of a manager.
public String toString()
{ // put your code below
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

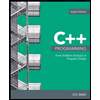
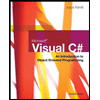
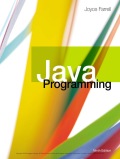
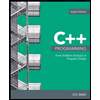
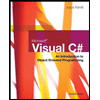
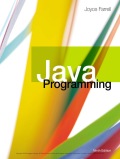