In this assignment, you're going to create your own methods and call them from main. We haven't talked about classes and Object-Oriented Programming (OOP) yet, so we're going to have to "break the rules" a bit. For the Java and C# folks, this means adding a special keyword before each method you write – called static. In simple terms, this means that we don't want to use OOP (though there's much, much more to it). Assignment 7A: What's your grade? In this class (as well as the lecture class), we calculate grades using a process called weighted averages". The formula works by averaging all the games in a particular category, then multiplying the result by a percentage (the weight"). All categories are then added up to give you your final grade. In our class, that would look líike this: Final Grade = (average(Labs) * 0.10) + (average(Assignments) * 0.40) + (average(Midterm) * 0.20) + (average(Final) * 0.30) For this assignment, you will build a program that can calculate your grade in CSE 1321L using the formula above. Your main method will ask users to input the following information: 13 individual lab grades (since the lowest is dropped per FYE policy) • 8 assignment grades • 1 midterm grade 1 final exam grade You will then create and use two methods outside of main to calculate your grade: A method to calculate the average value of a category. The method should take in an array of floats and the integer size of the array, and return a float value for the average of the category. A method to calculate the weighted points of a category. The method should take in the returned value from the previous method and a float value for the category's percentage (e.g. 0.4f) and return a float value for the weighted points of the category.
In this assignment, you're going to create your own methods and call them from main. We haven't talked about classes and Object-Oriented Programming (OOP) yet, so we're going to have to "break the rules" a bit. For the Java and C# folks, this means adding a special keyword before each method you write – called static. In simple terms, this means that we don't want to use OOP (though there's much, much more to it). Assignment 7A: What's your grade? In this class (as well as the lecture class), we calculate grades using a process called weighted averages". The formula works by averaging all the games in a particular category, then multiplying the result by a percentage (the weight"). All categories are then added up to give you your final grade. In our class, that would look líike this: Final Grade = (average(Labs) * 0.10) + (average(Assignments) * 0.40) + (average(Midterm) * 0.20) + (average(Final) * 0.30) For this assignment, you will build a program that can calculate your grade in CSE 1321L using the formula above. Your main method will ask users to input the following information: 13 individual lab grades (since the lowest is dropped per FYE policy) • 8 assignment grades • 1 midterm grade 1 final exam grade You will then create and use two methods outside of main to calculate your grade: A method to calculate the average value of a category. The method should take in an array of floats and the integer size of the array, and return a float value for the average of the category. A method to calculate the weighted points of a category. The method should take in the returned value from the previous method and a float value for the category's percentage (e.g. 0.4f) and return a float value for the weighted points of the category.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
language is c++
sample output included with the user input in bold
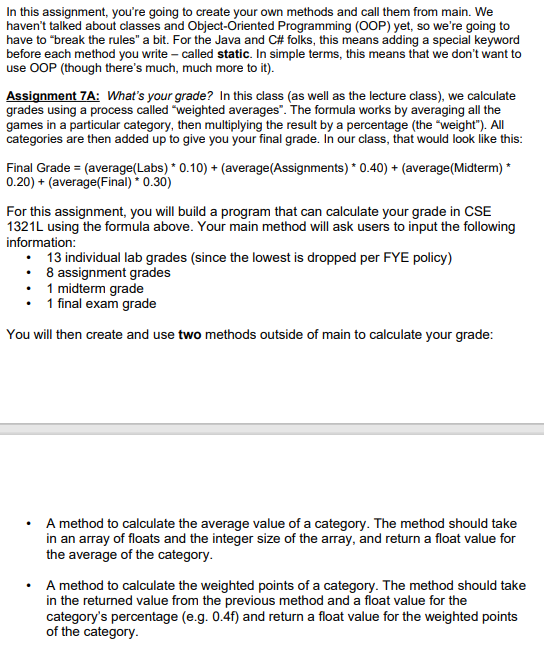
Transcribed Image Text:In this assignment, you're going to create your own methods and call them from main. We
haven't talked about classes and Object-Oriented Programming (OOP) yet, so we're going to
have to "break the rules" a bit. For the Java and C# folks, this means adding a special keyword
before each method you write – called static. In simple terms, this means that we don't want to
use OOP (though there's much, much more to it).
Assignment 7A: What's your grade? In this class (as well as the lecture class), we calculate
grades using a process called "weighted averages". The formula works by averaging all the
games in a particular category, then multiplying the result by a percentage (the "weight"). All
categories are then added up to give you your final grade. In our class, that would look like this:
Final Grade = (average(Labs) * 0.10) + (average(Assignments) * 0.40) + (average(Midterm) *
0.20) + (average(Final) * 0.30)
For this assignment, you will build a program that can calculate your grade in CSE
1321L using the formula above. Your main method will ask users to input the following
information:
• 13 individual lab grades (since the lowest is dropped per FYE policy)
• 8 assignment grades
• 1 midterm grade
1 final exam grade
You will then create and use two methods outside of main to calculate your grade:
• A method to calculate the average value of a category. The method should take
in an array of floats and the integer size of the array, and return a float value for
the average of the category.
• A method to calculate the weighted points of a category. The method should take
in the returned value from the previous method and a float value for the
category's percentage (e.g. 0.4f) and return a float value for the weighted points
of the category.
![Sample Output:
[CSE 1321L Grade Calculator]
Labs
Enter Grade #1: 70
Enter Grade #2: 90
Enter Grade #3: 100
Enter Grade #4: 56
Enter Grade #5: 70
Enter Grade #6: 98
Enter Grade #7: 105
Enter Grade #8: 67
Enter Grade #9: 100
Enter Grade #10: 78
Enter Grade #11: 91
Enter Grade #12: 92
Enter Grade #13: 100
Weighted Points: 8.59
Assignments
Enter Grade #1: 90
Enter Grade #2: 100
Enter Grade #3: 78
Enter Grade #4: 90
Enter Grade #5: 95
Enter Grade #6: 67
Enter Grade #7: 100
Enter Grade #8: 100
Weighted Points: 36.0
Midterm
Enter Grade #1: 98
Weighted Points: 19.6
Exam
Enter Grade #1: 100
Weighted Points: 30.0
Your final grade for CSE1321L is: 94.19](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff04f523d-cad1-46a0-8446-99b6c9606c43%2Fbcf843bf-745b-49bf-af19-f4acf74f9fb0%2F8sevxb_processed.png&w=3840&q=75)
Transcribed Image Text:Sample Output:
[CSE 1321L Grade Calculator]
Labs
Enter Grade #1: 70
Enter Grade #2: 90
Enter Grade #3: 100
Enter Grade #4: 56
Enter Grade #5: 70
Enter Grade #6: 98
Enter Grade #7: 105
Enter Grade #8: 67
Enter Grade #9: 100
Enter Grade #10: 78
Enter Grade #11: 91
Enter Grade #12: 92
Enter Grade #13: 100
Weighted Points: 8.59
Assignments
Enter Grade #1: 90
Enter Grade #2: 100
Enter Grade #3: 78
Enter Grade #4: 90
Enter Grade #5: 95
Enter Grade #6: 67
Enter Grade #7: 100
Enter Grade #8: 100
Weighted Points: 36.0
Midterm
Enter Grade #1: 98
Weighted Points: 19.6
Exam
Enter Grade #1: 100
Weighted Points: 30.0
Your final grade for CSE1321L is: 94.19
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
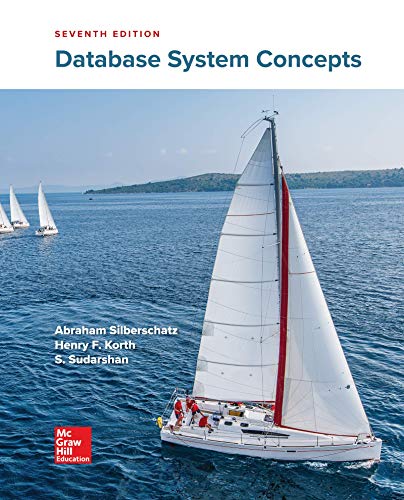
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
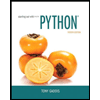
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
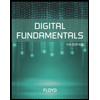
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
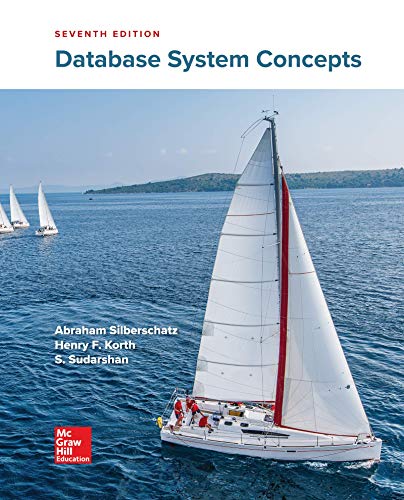
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
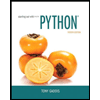
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
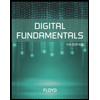
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
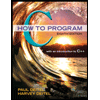
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
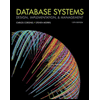
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
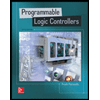
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education