Please broadly explain what the code in the driver class does for both of them. What do you think about the two driver class file? Which one was better? public class PersonFoodListDriver { public static void main(String[] args) { PersonFoodList myList = new PersonFoodList(); myList.addNode(new PersonFoodNode(new PersonFood("Emilia","Lemon Pudding Cake"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); PersonFood friend2 = new PersonFood("Peter", "Ramen"); PersonFoodNode friendPeter = new PersonFoodNode(friend2); myList.addNode(friendPeter); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); myList.addNode(new PersonFoodNode(new PersonFood("Isaac","Ziti"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); myList.addNode(new PersonFoodNode(new PersonFood("Isaac","Mac & Cheese"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); myList.addNode(new PersonFoodNode(new PersonFood("Eloise","Croissants"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); myList.addNode(new PersonFoodNode(new PersonFood("Amy","Fish Chews"))); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); System.out.println("DONE ADDING NAMES!"); System.out.println(); System.out.println(); System.out.println("Removing ISAAC"); myList.removeByName("Isaac"); System.out.println(myList.stringByName()); System.out.println(myList.stringByFood()); } } public class LLNodeDriver { public static String foodNodesString(myLLNode m) { String result = ""; myLLNode current = m; while (current.nextfood != null) { result = result + current.foodNodeString(); current = current.nextfood; } result = result + current.foodNodeString(); return result; } public static String nameNodesString(myLLNode m) { String result = ""; myLLNode current = m; while (current.nextname != null) { result = result + current.nameNodeString(); current = current.nextname; } result = result + current.nameNodeString(); return result; } public static void main(String[] args) { myLLNode myNode1 = new myLLNode("Francis"); myLLNode myNode2 = new myLLNode("Nini"); myLLNode myNode3 = new myLLNode("Norah"); myLLNode myNode4 = new myLLNode("Sarah"); myLLNode myNode5 = new myLLNode("Emily"); myNode3.nextname = myNode4; myNode2.nextname = myNode3; myNode1.nextname = myNode2; myNode5.nextname = myNode1; myLLNode front = myNode5; myLLNode current = myNode5; while (current.nextname != null) { System.out.print(current.nameNodeString()); current = current.nextname; } System.out.println(current.nameNodeString()); myLLNode newFriend = new myLLNode("Mako"); current = front; if(current.name.compareToIgnoreCase(newFriend.name) < 0) { if(current.nextname.name.compareToIgnoreCase(newFriend.name) < 0) { current = current.nextname; } else { if(current.nextname.name.compareToIgnoreCase(newFriend.name) >= 0) { newFriend.nextname = current.nextname; current.nextname = newFriend; break; } } } } if(current.nextname == null) { if(current.name.compareToIgnoreCase(newFriend.name) < 0) { current.nextname = newFriend; } } current = front; System.out.println(nameNodesString(current)); current = front; current.favfood = "Apple Sauce"; current = current.nextname; current.favfood = "Ice Cream"; current = current.nextname; current.favfood = "Chocolate Chip Cookies"; current = current.nextname; current.favfood = "Lasagna"; current = current.nextname; current.favfood = "Lentil Soup"; current = current.nextname; current.favfood = "Baked Potatoes"; current = front; System.out.print(nameNodesString(current)); System.out.println(); System.out.println(); current = front; myLLNode front2 = new myLLNode(current.name, current.favfood); myLLNode currfood = front2; while (current!= null) { String newName = current.name; String newFood = current.favfood; myLLNode nextName = current.nextname; myLLNode newNode = new myLLNode(newName, newFood, nextName); boolean newNodePlaced = false; if (newNode.favfood.compareToIgnoreCase(front2.favfood) < 0) { newNode.nextfood = front2; front2 = newNode; newNodePlaced = true; currfood = front2; // advance current current = current.nextname; } while (currfood.nextfood != null && newNodePlaced == false) { if (currfood.favfood.compareToIgnoreCase(newNode.favfood) < 0) { if(currfood.nextfood.favfood.compareToIgnoreCase(newNode.favfood) < 0) { currfood = currfood.nextfood; } else { if(currfood.nextfood.favfood.compareToIgnoreCase(newNode.favfood) >= 0) { newNode.nextfood = currfood.nextfood; currfood.nextfood = newNode; currfood = front2; newNodePlaced = true; current = current.nextname; break; } } } else { newNode.nextfood = currfood; currfood = newNode; currfood = front2; newNodePlaced = true; current = current.nextname; } } if (currfood.nextfood == null && newNodePlaced == false) { currfood.nextfood = newNode; newNode.nextfood = null; currfood = front2; newNodePlaced = true; current = current.nextname; } } current = front2; System.out.println("In FOOD order: " + foodNodesString(front2)); current = front; System.out.println("In NAME order: " + nameNodesString(front)); } }
- Please broadly explain what the code in the driver class does for both of them.
- What do you think about the two driver class file? Which one was better?
public class PersonFoodListDriver {
public static void main(String[] args) {
PersonFoodList myList = new PersonFoodList();
myList.addNode(new PersonFoodNode(new PersonFood("Emilia","Lemon Pudding Cake")));
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
PersonFood friend2 = new PersonFood("Peter", "Ramen");
PersonFoodNode friendPeter = new PersonFoodNode(friend2);
myList.addNode(friendPeter);
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
myList.addNode(new PersonFoodNode(new PersonFood("Isaac","Ziti")));
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
myList.addNode(new PersonFoodNode(new PersonFood("Isaac","Mac & Cheese")));
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
myList.addNode(new PersonFoodNode(new PersonFood("Eloise","Croissants")));
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
myList.addNode(new PersonFoodNode(new PersonFood("Amy","Fish Chews")));
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
System.out.println("DONE ADDING NAMES!");
System.out.println();
System.out.println();
System.out.println("Removing ISAAC");
myList.removeByName("Isaac");
System.out.println(myList.stringByName());
System.out.println(myList.stringByFood());
}
}
public class LLNodeDriver {
public static String
foodNodesString(myLLNode m) {
String result = "";
myLLNode current = m;
while (current.nextfood !=
null) {
result = result +
current.foodNodeString();
current = current.nextfood;
}
result = result +
current.foodNodeString();
return result;
}
public static String
nameNodesString(myLLNode m) {
String result = "";
myLLNode current = m;
while (current.nextname !=
null) {
result = result +
current.nameNodeString();
current = current.nextname;
}
result = result +
current.nameNodeString();
return result;
}
public static void
main(String[] args) {
myLLNode myNode1 = new
myLLNode("Francis");
myLLNode myNode2 = new
myLLNode("Nini");
myLLNode myNode3 = new
myLLNode("Norah");
myLLNode myNode4 = new
myLLNode("Sarah");
myLLNode myNode5 = new
myLLNode("Emily");
myNode3.nextname = myNode4;
myNode2.nextname = myNode3;
myNode1.nextname = myNode2;
myNode5.nextname = myNode1;
myLLNode front = myNode5;
myLLNode current = myNode5;
while (current.nextname !=
null) {
System.out.print(current.nameNodeString());
current = current.nextname;
}
System.out.println(current.nameNodeString());
myLLNode newFriend = new
myLLNode("Mako");
current = front;
if(current.name.compareToIgnoreCase(newFriend.name)
< 0) {
if(current.nextname.name.compareToIgnoreCase(newFriend.name)
< 0) {
current = current.nextname;
}
else {
if(current.nextname.name.compareToIgnoreCase(newFriend.name)
>= 0) {
newFriend.nextname =
current.nextname;
current.nextname = newFriend;
break;
}
}
}
}
if(current.nextname == null)
{
if(current.name.compareToIgnoreCase(newFriend.name)
< 0) {
current.nextname = newFriend;
}
}
current = front;
System.out.println(nameNodesString(current));
current = front;
current.favfood = "Apple
Sauce";
current = current.nextname;
current.favfood = "Ice
Cream";
current = current.nextname;
current.favfood =
"Chocolate Chip Cookies";
current = current.nextname;
current.favfood =
"Lasagna";
current = current.nextname;
current.favfood =
"Lentil Soup";
current = current.nextname;
current.favfood = "Baked
Potatoes";
current = front;
System.out.print(nameNodesString(current));
System.out.println();
System.out.println();
current = front;
myLLNode front2 = new
myLLNode(current.name, current.favfood);
myLLNode currfood = front2;
while (current!= null) {
String newName =
current.name;
String newFood =
current.favfood;
myLLNode nextName =
current.nextname;
myLLNode newNode = new
myLLNode(newName, newFood, nextName);
boolean newNodePlaced =
false;
if
(newNode.favfood.compareToIgnoreCase(front2.favfood) < 0) {
newNode.nextfood = front2;
front2 = newNode;
newNodePlaced = true;
currfood = front2;
// advance current
current = current.nextname;
}
while (currfood.nextfood !=
null && newNodePlaced == false) {
if
(currfood.favfood.compareToIgnoreCase(newNode.favfood) < 0) {
if(currfood.nextfood.favfood.compareToIgnoreCase(newNode.favfood)
< 0) {
currfood = currfood.nextfood;
}
else {
if(currfood.nextfood.favfood.compareToIgnoreCase(newNode.favfood)
>= 0) {
newNode.nextfood =
currfood.nextfood;
currfood.nextfood = newNode;
currfood = front2;
newNodePlaced = true;
current = current.nextname;
break;
}
}
}
else {
newNode.nextfood = currfood;
currfood = newNode;
currfood = front2;
newNodePlaced = true;
current = current.nextname;
}
}
if (currfood.nextfood == null
&& newNodePlaced == false) {
currfood.nextfood = newNode;
newNode.nextfood = null;
currfood = front2;
newNodePlaced = true;
current = current.nextname;
}
}
current = front2;
System.out.println("In
FOOD order: " + foodNodesString(front2));
current = front;
System.out.println("In
NAME order: " + nameNodesString(front));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

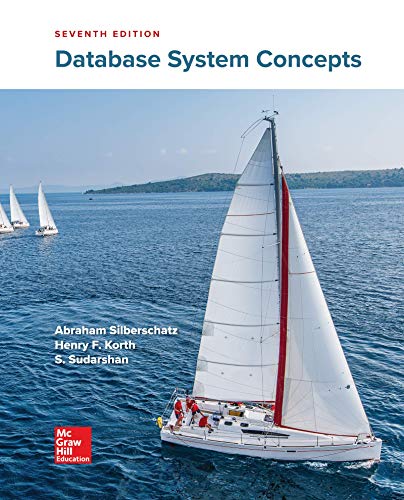
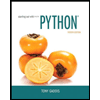
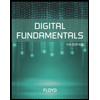
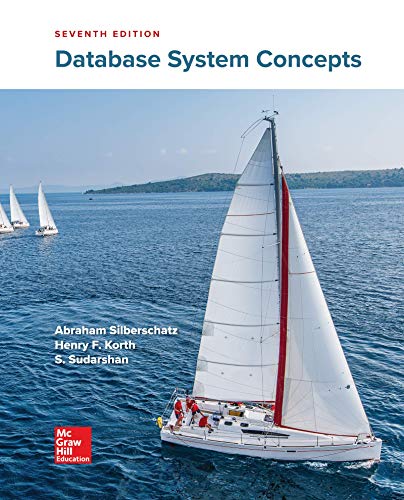
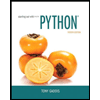
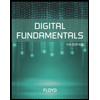
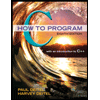
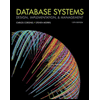
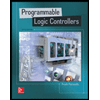