Order ID: 1000 Name: John Doe Butter 1 3.99 3.99 Muffin 2 1.49 2.98 Total 6.97 Order ID: 1001 Name: Jane Doe Juice 1 2.49 2.49 Bread 2 2.99 5.98 Total 8.47 Writing the code 1. main function Complete the main function such that it iterates over the orders array, and for each order, call the order's print method. 2. order: print method The order: :print method will print the order ID, customer name, and call the print method for the product items list. 3. item list::print method item list::print will print out all the items for that order, and then calculate and print out the total cost of the order. You will need to iterate over the items array and call the print method for each item. You will also need to keep a running sum of the total cost of the order. Call the cost method on each item to get the cost associated with each item. 4. item::print method item::print will print out one row of the order which consists of four parts - the product name, the unit price of one item, the quantity, and the cost (as defined below). Since the item object has only the sku, you will need to call find product to get a pointer to the product, which you can then use to get the name and price. 5. item:: cost method item:: cost will return the cost for one item -i.e. quantity multiplied by product unit cost. Like above, since the item object has only the sku, you will need to call Find product to get a pointer to the product, which you can then use to get the price. 6. find product function find product takes a sku as an argument and iterates through the products array comparing the product sku with the sku argument. When you find the matching product, return a pointer to that matching product. In order to compile the program, you need to make sure that both the .cpp files and the .h file are in the same directory. Then, you can compile the program by simply doing the following: * gcc -o hw6 hw6.cpp ordersdb.cpp To run the program, run as you have been doing for previous programs. Just make sure that the orders.db file is in the same directory. In this h you will do create a simple order management system using C++. Download the hw6.cpp, ordersdb.cpp, orders.h, and orders.db files from working directory. This is the skeletal code in hw5.cpp that you will need to complete #include #include "orders.h" using namespace std; product products [] { }; product (1234, "Bread", 2.99), product (5678, "Milk", 4.19), product (9012, "Eggs", 3.49), product (3456, "Butter", 3.99), product (7890, "Juice", 2.49), product (2345, "Muffin", 1.49) product find product (int sku) f } and put them in your // TODO complete the find product function. Iterate // through the products array and return the product // that has the matching sku // TODO write the product constructor and the following methods: // order: print(), item_list::print(), item:: cost(), // item::print() f int main() uint16_t num_orders; order** orders read_orders ("orders.db", &num_orders); if (orders NULL) { } printf("Order database does not exist\n"); for (int i=0; iprint(); // TODO iterate over orders array and call // the print method for each order } The orders.db file contains a database of orders with customer names and a list of items that the customer has purchased. I have provided the read_orders function for you to read the contents of the file and put it in an array called orders-which is an array of pointers to order objects. You can look in orders.h to see the definition of the order class. Also, within the order class is a special class called item list which contains a list of item objects. The definition of both item list and item are also in orders.h. Each item refers to a product that was purchased on the order and the item contains a product sku and a quantity. The product sku refers to a product in the products array which is an array of product objects where each product has a sku, name, and price. Your goal is to output all of the orders in a format that should look something like the following:
orderdp.cpp:
order.h:
#include <string>
#include <stdint.h>
class product {
uint16_t sku;
std::string name;
float price;
public:
product(uint16_t, std::string, float);
std::string getName() {return name;}
float getPrice() {return price;}
friend product* find_product(int sku);
void print();
};
class item {
uint16_t sku;
uint16_t quantity;
public:
item(uint16_t, uint16_t);
void print();
float cost();
};
class item_list {
item **items;
uint16_t num_products;
public:
item_list();
void add(const item&);
void print();
};
class order
{
uint16_t order_id;
std::string customer_name;
item_list product_items;
public:
void addItem(const item&);
void print();
order(uint16_t, std::string);
};
order** read_orders(const char* filename, uint16_t* num_orders);
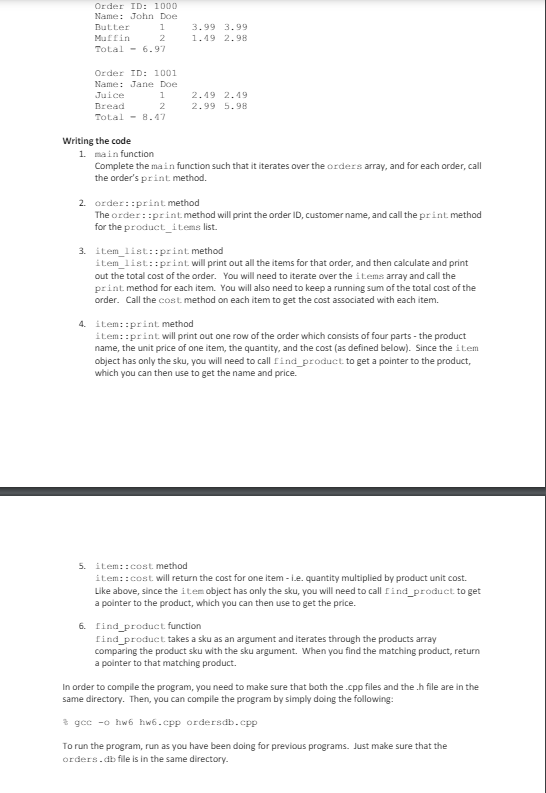
![In this h
you will do create a simple order management system using C++. Download the
hw6.cpp, ordersdb.cpp, orders.h, and orders.db files from
working directory.
This is the skeletal code in hw5.cpp that you will need to complete
#include <stdio.h>
#include "orders.h"
using namespace std;
product products [] {
};
product (1234, "Bread", 2.99),
product (5678, "Milk", 4.19),
product (9012, "Eggs", 3.49),
product (3456, "Butter", 3.99),
product (7890, "Juice", 2.49),
product (2345, "Muffin", 1.49)
product find product (int sku)
f
}
and put them in your
// TODO complete the find product function. Iterate
// through the products array and return the product
// that has the matching sku
// TODO write the product constructor and the following methods:
// order: print(), item_list::print(), item:: cost(),
// item::print()
f
int main()
uint16_t num_orders;
order** orders read_orders ("orders.db", &num_orders);
if (orders NULL) {
}
printf("Order database does not exist\n");
for (int i=0; i<num_orders; i++) {
}
orders[i]->print();
// TODO iterate over orders array and call
// the print method for each order
}
The orders.db file contains a database of orders with customer names and a list of items that the
customer has purchased. I have provided the read_orders function for you to read the contents of
the file and put it in an array called orders-which is an array of pointers to order objects. You can
look in orders.h to see the definition of the order class. Also, within the order class is a special
class called item list which contains a list of item objects. The definition of both item list and
item are also in orders.h. Each item refers to a product that was purchased on the order and the
item contains a product sku and a quantity. The product sku refers to a product in the products array
which is an array of product objects where each product has a sku, name, and price.
Your goal is to output all of the orders in a format that should look something like the following:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F56b64867-19ea-4abe-b1cd-abafded5f4ed%2Fe960574b-2e75-44ad-b697-47b1e0d0231d%2Fw2wu09o_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

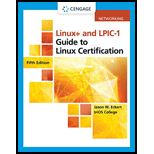
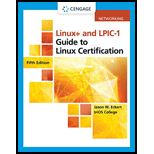