In this java assignment, we will be creating a pay stub for an employee using classes. Each class must be implemented into their own file, a total of 5 classes/files. We are going to implement the following classes: Employee – This class represents an employee. It needs the following fields exposed via getters and setters: Employee ID (we will need to make this unique, for now, just hard code it to 1). First name, Last name, Middle initial Address, City, Zip Phone, Email Hourly Rate PayPeriod – This class represents an employee’s payment information. An employee will eventually have more than one pay period. It must contain the following fields (exposed via getters and setters): Pay period Id (hard code it for now) Employee Id (who is this for) Start Date, End Date Number of hours PayrollManager – This class provides the functionality we need to compute and display our payroll. It should implement the following methods: double CalculateGrossPay (Employee, Payperiod) – This should return the total gross for the payperiod double CalculateRegularPay(Employee, Payperiod) – This should return the gross paid at the regular rate for the pay period double CalculateOvertimePay(Employee, Payperiod) – This should return ONLY the gross overtime for the pay period void PrintPaystub(Employee, Payperiod) – This should print out the paystub for the employee for the period. Note that here, we should use the other methods we defined above to implement this method. TaxPayment - This class will calculate different taxes to be deducted from the total gross. It should implement the following fields: FederalTax - Federal Tax Rate StateTax - State Tax Rate FicaTax - Fica Tax Rate Add an instance of this class to the pay period class so we have a place to store it Create a new class called TaxManagerthat has the following methods: TaxPayment ComputeTaxPayment(double grossPay, TaxRates taxRates) – This should return the taxes for this employee, which can then be stored in the payperiod class We are making TaxManager a separate class so eventually we have a place to put different tax rates Use the following default rates for tax rates FederalRate – 15% StateRate – 5% FicaRate – 3% You should use TaxManager in your printPaystub method to calculate and display the appropriate taxes. Your main program will then do the following: Gather input and put it in an instance of Employee Gather payroll info and put it into an instance of Payperiod (default the dates for now) Call Print Paystub to display it.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Employee – This class represents an employee. It needs the following fields exposed via getters and setters:
- Employee ID (we will need to make this unique, for now, just hard code it to 1).
- First name, Last name, Middle initial
- Address, City, Zip
- Phone, Email
- Hourly Rate
PayPeriod – This class represents an employee’s payment information. An employee will eventually have more than one pay period. It must contain the following fields (exposed via getters and setters):
- Pay period Id (hard code it for now)
- Employee Id (who is this for)
- Start Date, End Date
- Number of hours
PayrollManager – This class provides the functionality we need to compute and display our payroll. It should implement the following methods:
-
double CalculateGrossPay (Employee, Payperiod) – This should return the total gross for the payperiod
-
double CalculateRegularPay(Employee, Payperiod) – This should return the gross paid at the regular rate for the pay period
-
double CalculateOvertimePay(Employee, Payperiod) – This should return ONLY the gross overtime for the pay period
-
void PrintPaystub(Employee, Payperiod) – This should print out the paystub for the employee for the period. Note that here, we should use the other methods we defined above to implement this method.
- FederalTax - Federal Tax Rate
- StateTax - State Tax Rate
- FicaTax - Fica Tax Rate
- Add an instance of this class to the pay period class so we have a place to store it
- Create a new class called TaxManagerthat has the following methods:
- TaxPayment ComputeTaxPayment(double grossPay, TaxRates taxRates) – This should return the taxes for this employee, which can then be stored in the payperiod class
- We are making TaxManager a separate class so eventually we have a place to put different tax rates
- Use the following default rates for tax rates
- FederalRate – 15%
- StateRate – 5%
- FicaRate – 3%
- You should use TaxManager in your printPaystub method to calculate and display the appropriate taxes.
Your main program will then do the following:
- Gather input and put it in an instance of Employee
- Gather payroll info and put it into an instance of Payperiod (default the dates for now)
- Call Print Paystub to display it.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

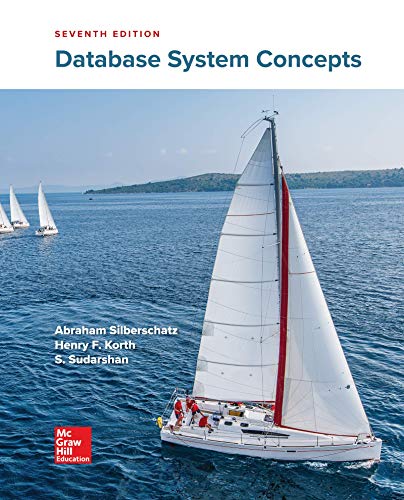
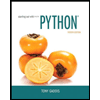
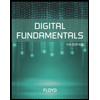
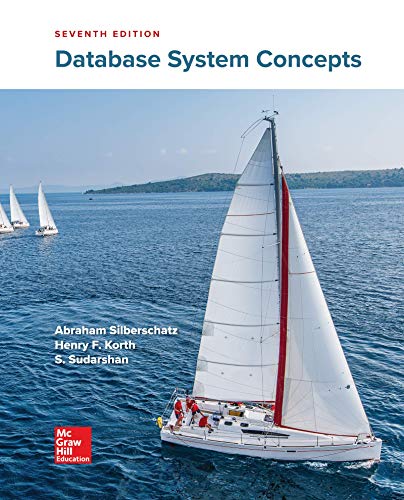
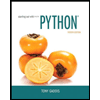
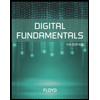
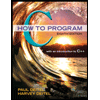
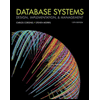
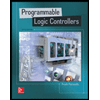