In this lab, you complete a partially written Java program that includes a method that returns a value. The program is a simple calculator that prompts the user for two numbers and an operator ( +, −, *, /,or% ). The two numbers and the operator are passed to the method where the appropriate arithmetic operation is performed. The result is then returned to the main() method where the arithmetic operation and result are displayed. For example, if the user enters 3, 4, and *, the following is displayed: 3.00 * 4.00 = 12.00 The source code file provided for this lab includes the necessary variable declarations, and input and output statements. Comments are included in the file to help you write the remainder of the program. Instructions Write the Java statements as indicated by the comments. Execute the program Tasks Defined performOperation() Input / output tests performOperation unit tests performOperation addition test performOperation multiplication test Use The Given Code // Calculator.java - This program performs arithmetic, ( +. -, *. /, % ) on two numbers // Input: Interactive. // Output: Result of arithmetic operation import java.util.Scanner; public class Calculator { public static void main(String args[]) { double numberOne, numberTwo; String numberOneString, numberTwoString; String operation; double result; Scanner input = new Scanner(System.in); System.out.println("Enter the first number: "); numberOneString = input.nextLine(); numberOne = Double.parseDouble(numberOneString); System.out.println("Enter the second number: "); numberTwoString = input.nextLine(); numberTwo = Double.parseDouble(numberTwoString); System.out.println("Enter an operator (+.-.*,/,%): "); operation = input.nextLine(); // Call performOperation method here System.out.format("%.2f",numberOne); System.out.print(" " + operation + " "); System.out.format("%.2f", numberTwo); System.out.print(" = "); System.out.format("%.2f", result); System.exit(0); } // End of main() method. // Write performOperation method here. } // End of Calculator class.
Writing Methods that Return a Value in Java
In this lab, you complete a partially written Java program that includes a method that returns a value. The program is a simple calculator that prompts the user for two numbers and an operator ( +, −, *, /,or% ). The two numbers and the operator are passed to the method where the appropriate arithmetic operation is performed. The result is then returned to the main() method where the arithmetic operation and result are displayed. For example, if the user enters 3, 4, and *, the following is displayed:
3.00 * 4.00 = 12.00
The source code file provided for this lab includes the necessary variable declarations, and input and output statements. Comments are included in the file to help you write the remainder of the program.
Instructions
- Write the Java statements as indicated by the comments.
- Execute the program
Tasks
- Defined performOperation()
- Input / output tests
- performOperation unit tests
- performOperation addition test
- performOperation multiplication test
Use The Given Code

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

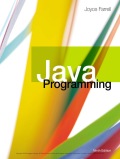
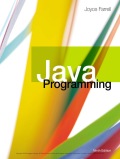