Modify the program below to create a class that contains a static method; the method takes a string as a parameter and returns a boolean value indicating whether the parameter string has repeated characters in it or not. That is, return true if there is at least one character which appears more than once in the string. The string should be input by the .user import java .util.*; public class Main { public static void main(String args[]) { Scanner scn=new Scanner(System.in); // Input the string from user System.out.println ( "Enter the String to check for duplicate characters:"); String str = scn.next(); // Check is variable to store bool value true // It will change to true if there are repeated characters boolean check=false; for (int a = 0; a < str.length(); a++) for (int b = a + 1; b < str.length(); b++) if (str.charAt(a) == str.charAt(b)) check=true; // If check is true then string has repeated characters if (check) System.out.println("The String " + str + " has repeated characters"); else System.out.println("The String " + str + " has no repeated characters"); } } //Ends
Modify the program below to create a class that contains a static method; the method takes a string as a parameter and returns a boolean value indicating whether the parameter string has repeated characters in it or not. That is, return true if there is at least one character which appears more than once in the string. The string should be input by the .user
import java .util.*;
public class Main
{
public static void main(String args[])
{
Scanner scn=new Scanner(System.in);
// Input the string from user
System.out.println ( "Enter the String to check for duplicate characters:");
String str = scn.next();
// Check is variable to store bool value true
// It will change to true if there are repeated characters
boolean check=false;
for (int a = 0; a < str.length(); a++)
for (int b = a + 1; b < str.length(); b++)
if (str.charAt(a) == str.charAt(b))
check=true;
// If check is true then string has repeated characters
if (check)
System.out.println("The String " + str + " has repeated characters");
else
System.out.println("The String " + str + " has no repeated characters");
}
}
//Ends

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

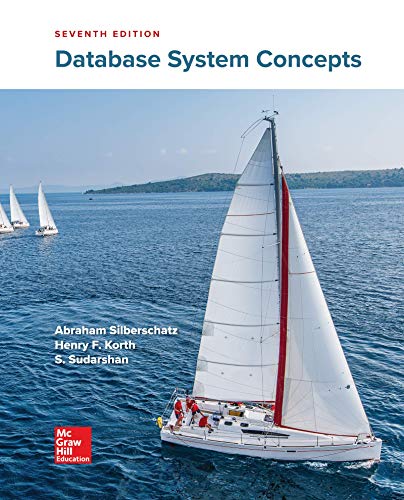
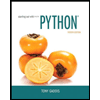
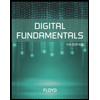
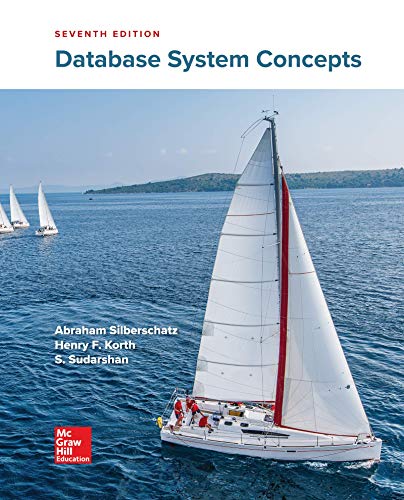
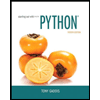
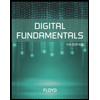
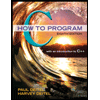
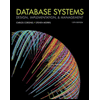
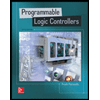