In this task, you will implement a recursive function all_perm(n: int) -> set[tuple[int, that takes an integer n> 0 and returns a set containing all the permutations of 1,2,3,..., n. Each per- mutation must be represented as a tuple. For example: ...]] • all_perm(1) • all_perm(2) • all_perm(3) {(1,)} == {(1,2), (2,1)} == == {(1,2,3), (1,3,2), (2,1,3), (2,3,1), (3,1,2), (3,2,1)} Restrictions: Do not write a helper function. Your function must be recursive, and must work with sets and tuples directly. You are not allowed to import anything. Hints: Consider how we can use all_perm(2) to create the answer for all_perm(3). Perhaps the following diagram will help (pay close attention to the color coding and numbers in boldface fonts): all_perm(2) == {(1,2), (2, 1)} all_perm(3) == {(3,1,2), (1,3,2), (1,2,3), (3,2, 1), (2,3, 1), (2, 1,3)} all_perm(4)== {(4,3,1,2), (3,4, 1,2), (3, 1, 4, 2), (3, 1, 2, 4), (4, 1,3,2), (1,4,3,2), (1,3,4, 2), (1,3,2,4), (4,1,2,3), (1,4,2,3), (1,2,4, 3), (1,2,3, 4), (4,3,2,1), (3,4,2, 1), (3,2,4, 1), (3,2, 1,4), (4,2,3, 1), (2,4,3, 1), (2,3, 4, 1), (2,3, 1,4), (4,2, 1,3), (2,4, 1,3), (2,1,4,3), (2,1,3,4)}
In this task, you will implement a recursive function all_perm(n: int) -> set[tuple[int, that takes an integer n> 0 and returns a set containing all the permutations of 1,2,3,..., n. Each per- mutation must be represented as a tuple. For example: ...]] • all_perm(1) • all_perm(2) • all_perm(3) {(1,)} == {(1,2), (2,1)} == == {(1,2,3), (1,3,2), (2,1,3), (2,3,1), (3,1,2), (3,2,1)} Restrictions: Do not write a helper function. Your function must be recursive, and must work with sets and tuples directly. You are not allowed to import anything. Hints: Consider how we can use all_perm(2) to create the answer for all_perm(3). Perhaps the following diagram will help (pay close attention to the color coding and numbers in boldface fonts): all_perm(2) == {(1,2), (2, 1)} all_perm(3) == {(3,1,2), (1,3,2), (1,2,3), (3,2, 1), (2,3, 1), (2, 1,3)} all_perm(4)== {(4,3,1,2), (3,4, 1,2), (3, 1, 4, 2), (3, 1, 2, 4), (4, 1,3,2), (1,4,3,2), (1,3,4, 2), (1,3,2,4), (4,1,2,3), (1,4,2,3), (1,2,4, 3), (1,2,3, 4), (4,3,2,1), (3,4,2, 1), (3,2,4, 1), (3,2, 1,4), (4,2,3, 1), (2,4,3, 1), (2,3, 4, 1), (2,3, 1,4), (4,2, 1,3), (2,4, 1,3), (2,1,4,3), (2,1,3,4)}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
With python
Please begin by implementing the function: all_perm(n: int) -> set[tuple[int, ...]]
![In this task, you will implement a recursive function all_perm(n: int) -> set[tuple[int, ...]]
that takes an integer n> 0 and returns a set containing all the permutations of 1,2,3,..., n. Each per-
mutation must be represented as a tuple. For example:
• all_perm(1)
• all_perm(2)
• all_perm(3)
{(1,)}
{(1,2), (2,1)}
==
{(1,2,3), (1,3,2), (2,1,3), (2,3,1), (3,1,2), (3,2,1)}
%3D%3D
Restrictions: Do not write a helper function. Your function must be recursive, and must work with
sets and tuples directly. You are not allowed to import anything.
Hints: Consider how we can use all_perm(2) to create the answer for all_perm(3). Perhaps the
following diagram will help (pay close attention to the color coding and numbers in boldface fonts):
all_perm(2) == {(1,2), (2,1)}
all_perm(3) == {(3,1,2), (1,3,2), (1,2,3), (3,2,1), (2,3, 1), (2,1,3)}
all_perm(4) == {(4,3,1,2), (3,4, 1,2), (3, 1, 4, 2), (3, 1,2, 4),
(4, 1,3,2), (1,4,3, 2), (1,3,4,2), (1,3,2,4),
(4, 1,2,3), (1,4,2,3), (1,2,4,3), (1,2,3,4),
(4,3,2, 1), (3, 4, 2, 1), (3,2, 4, 1), (3,2, 1,4),
(4,2,3, 1), (2, 4, 3, 1), (2,3, 4, 1), (2,3,1,4),
(4,2, 1,3), (2,4, 1,3), (2,1,4, 3), (2,1,3,4)}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6954e458-8753-495c-94b2-cdcc9bbfd17e%2F1bba53dd-1c82-44e1-874a-dac76dd15d20%2Ftrprp7a_processed.png&w=3840&q=75)
Transcribed Image Text:In this task, you will implement a recursive function all_perm(n: int) -> set[tuple[int, ...]]
that takes an integer n> 0 and returns a set containing all the permutations of 1,2,3,..., n. Each per-
mutation must be represented as a tuple. For example:
• all_perm(1)
• all_perm(2)
• all_perm(3)
{(1,)}
{(1,2), (2,1)}
==
{(1,2,3), (1,3,2), (2,1,3), (2,3,1), (3,1,2), (3,2,1)}
%3D%3D
Restrictions: Do not write a helper function. Your function must be recursive, and must work with
sets and tuples directly. You are not allowed to import anything.
Hints: Consider how we can use all_perm(2) to create the answer for all_perm(3). Perhaps the
following diagram will help (pay close attention to the color coding and numbers in boldface fonts):
all_perm(2) == {(1,2), (2,1)}
all_perm(3) == {(3,1,2), (1,3,2), (1,2,3), (3,2,1), (2,3, 1), (2,1,3)}
all_perm(4) == {(4,3,1,2), (3,4, 1,2), (3, 1, 4, 2), (3, 1,2, 4),
(4, 1,3,2), (1,4,3, 2), (1,3,4,2), (1,3,2,4),
(4, 1,2,3), (1,4,2,3), (1,2,4,3), (1,2,3,4),
(4,3,2, 1), (3, 4, 2, 1), (3,2, 4, 1), (3,2, 1,4),
(4,2,3, 1), (2, 4, 3, 1), (2,3, 4, 1), (2,3,1,4),
(4,2, 1,3), (2,4, 1,3), (2,1,4, 3), (2,1,3,4)}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
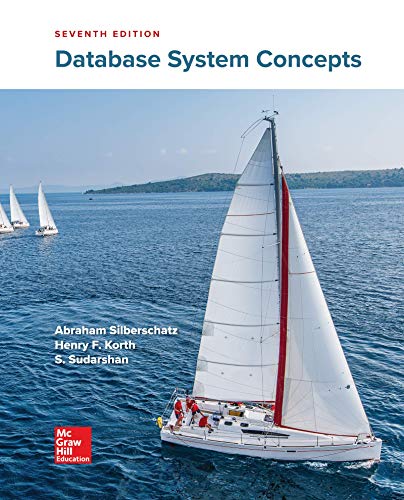
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
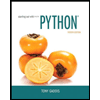
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
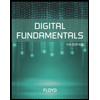
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
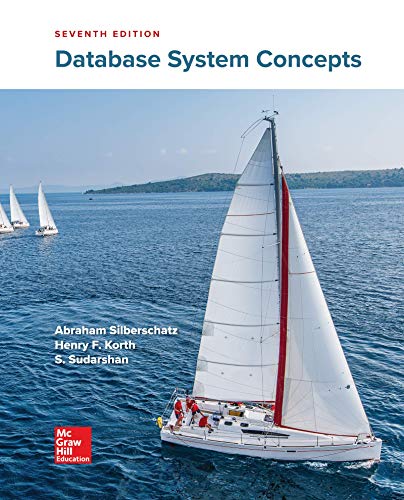
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
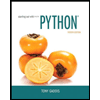
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
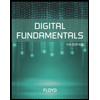
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
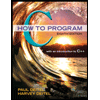
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
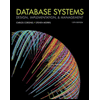
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
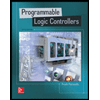
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education