Write a recursive function that, given a sequence of comparable values, returns the count of elements where the current element is less than the following ( next ) element in the given sequence. See the examples given below. def count_ordered ( seq ) : """ Input : A sequence of comparable elements Output : The number of elements that are less than the following element in the sequence Example : >>> count_ordered ( [ 1 , 2 , 3 , 4 , 5 , 6 ] ) 5 >>> count_ordered ( ( 1 , 12, 7.3 , -2,4 ) ) 2 >>> count_ordered ( 'Python' ) 2 >>> count_ordered ( [ 6 ] ) 0 >>> count_ordered ( [ ] ) 0 """ In the first example above , count_ordered ( [ 1,2,3,4,5,6 ] ) the returned answer is 5 because for all the first 5 numbers the current number is less than the next number. In the second example above, count_ordered ( ( 1,12,7.3 , -2,4 ) ) the returned answer is 2 because there are two cases where the current value is less than the next value, i.e. 1 is less than 12 and -2 is less than 4.
Write a recursive function that, given a sequence of comparable values, returns the count of elements where the current element is less than the following ( next ) element in the given sequence. See the examples given below.
def count_ordered ( seq ) :
"""
Input : A sequence of comparable elements
Output : The number of elements that are less than the following element in the sequence
Example :
>>> count_ordered ( [ 1 , 2 , 3 , 4 , 5 , 6 ] )
5
>>> count_ordered ( ( 1 , 12, 7.3 , -2,4 ) )
2
>>> count_ordered ( 'Python' )
2
>>> count_ordered ( [ 6 ] )
0
>>> count_ordered ( [ ] )
0
"""
In the first example above , count_ordered ( [ 1,2,3,4,5,6 ] )
the returned answer is 5 because for all the first 5 numbers the current number is less than the next number.
In the second example above, count_ordered ( ( 1,12,7.3 , -2,4 ) )
the returned answer is 2 because there are two cases where the current value is less than the next value, i.e. 1 is less than 12 and -2 is less than 4.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

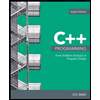
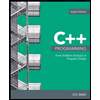