#include #include struct info{ char names[100]; int age; float wage; }; int main(int argc, char* argv[]) { int line_count = 0; int index, i; struct info hr[10]; if (argc != 2) printf("Invalid user_input!\n"); else { FILE* contents = fopen (argv[1], "r"); struct info in; if (contents != NULL) { printf("File has been opened successfully.\n\n"); while (fscanf(contents, "%s %d %f\n",hr[line_count].names, &hr[line_count].age, &hr[line_count].wage) != EOF) { printf("%s %d %f\n", hr[line_count].names, hr[line_count].age, hr[line_count].wage); line_count++; } printf("\nTotal number of lines in document are: %d\n\n", line_count); fclose(contents); printf("Please enter a name: "); char user_input[100]; fgets(user_input, 30, stdin); user_input[strlen(user_input)-1] = '\0'; index = -1; for(i = 0; i < line_count; i++){ if(strcmp(hr[i].names, user_input) == 0){ index = i; break; } } if(index == -1) printf("Name %s not found\n", user_input); else{ while(fread(&in, sizeof(struct info), 1, contents)) printf("Name: %s, Age: %d, Wage: %.2f\n", user_input, in.age, in.wage); } } else { printf("File cannot be opened!"); } } return 0; } In C Write a program that expands on the previous question (i.e. reading data of each record in the file and storing it into your data structure). The expansion involves writing all records to a file (use fprintf, call name the file output.csv). The displayed data must be accessed (and thus written) from your data structure (i.e. array of structs). The format written to the file should be one line per record, with each field separated by a comma (‘,’). The code for this should occur after the code for point 1f above and before point 1g. Close both files. Your program should then output the number of lines in the file – this code can occur after point 1f. You should test the program by creating a text file with at least 10 lines of text (of any composition). Use notepad++ to create the data file.
#include<stdio.h>
#include<string.h>
struct info{
char names[100];
int age;
float wage;
};
int main(int argc, char* argv[]) {
int line_count = 0;
int index, i;
struct info hr[10];
if (argc != 2)
printf("Invalid user_input!\n");
else {
FILE* contents = fopen (argv[1], "r");
struct info in;
if (contents != NULL) {
printf("File has been opened successfully.\n\n");
while (fscanf(contents, "%s %d %f\n",hr[line_count].names, &hr[line_count].age, &hr[line_count].wage) != EOF) {
printf("%s %d %f\n", hr[line_count].names, hr[line_count].age, hr[line_count].wage);
line_count++;
}
printf("\nTotal number of lines in document are: %d\n\n", line_count);
fclose(contents);
printf("Please enter a name: ");
char user_input[100];
fgets(user_input, 30, stdin);
user_input[strlen(user_input)-1] = '\0';
index = -1;
for(i = 0; i < line_count; i++){
if(strcmp(hr[i].names, user_input) == 0){
index = i;
break;
}
}
if(index == -1)
printf("Name %s not found\n", user_input);
else{
while(fread(&in, sizeof(struct info), 1, contents))
printf("Name: %s, Age: %d, Wage: %.2f\n", user_input, in.age, in.wage);
}
} else {
printf("File cannot be opened!");
}
}
return 0;
}
In C
Write a
each record in the file and storing it into your data structure). The expansion
involves writing all records to a file (use fprintf, call name the file
output.csv). The displayed data must be accessed (and thus written) from your
data structure (i.e. array of structs). The format written to the file should be
one line per record, with each field separated by a comma (‘,’).
The code for this should occur after the code for point 1f above and before
point 1g. Close both files.
Your program should then output the number of lines in the file – this code
can occur after point 1f. You should test the program by creating a text file
with at least 10 lines of text (of any composition). Use notepad++ to create the
data file.

Step by step
Solved in 2 steps

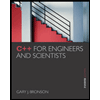
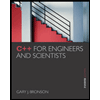