Instructions Complete the following tasks: Use the Class Wizard in Visual Studio to create a class called Student. (Note that the Class Wizard will automatically create the .h and .cpp files.) Your Student class should have the following class members/properties: studentID, firstName, lastName, and studentStatus. (Note that the studentStatus member will be of type Status, which is an enum you will create.) Create a default constructor and a constructor that requires only the Student Id, first name, and last name. (The studentStatus member should not be initialized at this time.) Create a member function called getStudentInfo() that returns a string that contains the students first name, last name, and their status. Create a destructor for the Student class. (Note that the Class Wizard automatically includes a destructor.) Add a status.h file to the solution. Then add a Status enumeration to that file with the following entries: Attending, NonAttending, and Graduated. Then include this file in your class file. Add #pragma once to all of your header files Write a simple program that asks the user for the Student Id, first name, and last name of the student. Then instantiates a new student object with this information. Ask the user if the student is presently attending the school. If the user states "no", ask if a degree was obtain. Use the ternary operator to set studentStatus member of the object to either NonAttending or Graduated based on whether a degree was obtained. If the user states that the student is attending, set the studentStatus to Attending. Display the student's information by calling the getStudentInfo() member function. Add the following to your program: Create a new variable in your main called numStudents to store the number of students to add to the system. Create an array of size 20 called students[] to hold all of the student objects. Create a function called getNumStudents() which will obtain the number of students to add. This function will ask the user for the number of students to add and store that number into the numStudents variable. The numStudents variable should be passed by reference to the function. (Note that because this number will be used in the array, it must be validated to be >= 0 and <= 20.) Use function prototypes as described in your textbook. Use the newly initialized numStudents variable in the main() to create a loop that adds that many student objects to the array. Create getter and setter member functions for each of the members of the class. These functions should be implemented in the header file. (Be sure to mark your getters as const.) Finally, create a function called displayStudents() that accepts an array of student objects and iterates through that array to display all of the students and their information by calling the getters. The function should use the member function getStudentInfo().
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Instructions Complete the following tasks: Use the Class Wizard in Visual Studio to create a class called Student. (Note that the Class Wizard will automatically create the .h and .cpp files.) Your Student class should have the following class members/properties: studentID, firstName, lastName, and studentStatus. (Note that the studentStatus member will be of type Status, which is an enum you will create.) Create a default constructor and a constructor that requires only the Student Id, first name, and last name. (The studentStatus member should not be initialized at this time.) Create a member function called getStudentInfo() that returns a string that contains the students first name, last name, and their status. Create a destructor for the Student class. (Note that the Class Wizard automatically includes a destructor.) Add a status.h file to the solution. Then add a Status enumeration to that file with the following entries: Attending, NonAttending, and Graduated. Then include this file in your class file. Add #pragma once to all of your header files Write a simple program that asks the user for the Student Id, first name, and last name of the student. Then instantiates a new student object with this information. Ask the user if the student is presently attending the school. If the user states "no", ask if a degree was obtain. Use the ternary operator to set studentStatus member of the object to either NonAttending or Graduated based on whether a degree was obtained. If the user states that the student is attending, set the studentStatus to Attending. Display the student's information by calling the getStudentInfo() member function. Add the following to your program: Create a new variable in your main called numStudents to store the number of students to add to the system. Create an array of size 20 called students[] to hold all of the student objects. Create a function called getNumStudents() which will obtain the number of students to add. This function will ask the user for the number of students to add and store that number into the numStudents variable. The numStudents variable should be passed by reference to the function. (Note that because this number will be used in the array, it must be validated to be >= 0 and <= 20.) Use function prototypes as described in your textbook. Use the newly initialized numStudents variable in the main() to create a loop that adds that many student objects to the array. Create getter and setter member functions for each of the members of the class. These functions should be implemented in the header file. (Be sure to mark your getters as const.) Finally, create a function called displayStudents() that accepts an array of student objects and iterates through that array to display all of the students and their information by calling the getters. The function should use the member function getStudentInfo().

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 8 images

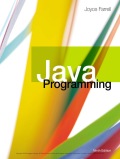
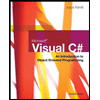
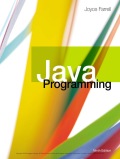
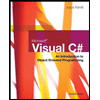