Little debugging help The program runs correctly but doesn't follow instructions completely. Currently, I have a parameterized constructor in the circle class when it asked for default I need help to fix the circle subclass. Please Write a default constructor that initializes the name of the Circle object to "Circle". You will need to call the constructor of the parent Shape class. The program runs everything in ShapeDriver /* * * Write a Circle class that extends the Shape class. Do the following: * 1. Write the class header. * 2. Write a default constructor that initializes the name of the Circle object to "Circle". * You will need to call the constructor of the parent Shape class. * 3. Write a void method printMessage() that prints the message " I have no sides". * */ //Your code goes here public class Circle extends Shape { public Circle(String myName) { super(myName); } public void printMessage() { System.out.println("I have no sides"); } } /** * * Write a Rectangle class that extends the Shape class. Do the following: * 1. Write the class header. * 2. Write a default constructor that initializes the name of the Rectangle object to "Rectangle". * You will need to call the constructor of the parent Shape class. * 3. Write a void method printMessage() that prints the message "I am a 4 sided figure". * */ //Your code goes here public class Rectangle extends Shape { public Rectangle(String myName) { super(myName); } public void printMessage() { System.out.println("I am a 4 sided figure"); } } /** * * Write a Shape class. Do the following: * 1. Write a class header for the Shape class. * 2. Create a String attribute that holds the name of the Shape object. Use the keyword String to * declare a variable of your choice. * 3. Write a parameterized constructor that initializes the name of the Shape object. * 4. Write a void method printName() that prints the name of the Shape object. * */ //Your code goes here public class Shape { public Shape(String myName) { } public void printName(String myName) { System.out.println("I am a " + myName); } } /** * In this lab you will practice inheritance You will need * to use System.out.println (String) to print messages to the console. * * Write a driver class to test the methods of the Shape class and it's sub classes * Do the following: * 1. Create a Shape object called "Irregular shape". Call it myShape. * 2. Print myShape's name. * 3. Create a Rectangle object called "Rectangle". Call it myRect. * 4. Print myRect's name. * 5. Print myRect's message. * 6. Create a Square object. Call it mySqr. * 7. Print mySqr's name. * 8. Print mySqr's message. * 9. Create a Circle object. Call it myCircle. * 7. Print myCircle's name. * 8. Print myCircle's message. * */ public class ShapesDriver { public static void main(String[] args) { Shape myShape = new Shape("Irregular Shape"); myShape.printName("Irregular Shape"); Rectangle myRect = new Rectangle("Rectangle"); myRect.printName("Rectangle"); myRect.printMessage(); Square mySqr = new Square("Rectangle"); mySqr.printName("Rectangle"); mySqr.printMessage(); Circle myCircle = new Circle("Circle"); myCircle.printName("Circle"); myCircle.printMessage(); } } /** * Write a Square class that extends the Rectangle class. Do the following: * 1. Write the class header. * 2. Write a default constructor that initializes the name of the Square object to "Rectangle". * You will need to call the constructor of the parent Rectangle class. * 3. Write a void method printMessage() that prints the message * "I am a 4 sided figure * I have equal sides" * You must call the parent's printMessage() method using super.printMessage(). * */ //Your code goes here public class Square extends Rectangle { public Square(String myName) { super(myName); } public void printMessage() { System.out.println("I am a 4 sided figure \n I have equal sides"); } }
Little debugging help
The program runs correctly but doesn't follow instructions completely. Currently, I have a parameterized constructor in the circle class when it asked for default
I need help to fix the circle subclass. Please Write a default constructor that initializes the name of the Circle object to "Circle". You will need to call the constructor of the parent Shape class.
The program runs everything in ShapeDriver
/*
*
* Write a Circle class that extends the Shape class. Do the following:
* 1. Write the class header.
* 2. Write a default constructor that initializes the name of the Circle object to "Circle".
* You will need to call the constructor of the parent Shape class.
* 3. Write a void method printMessage() that prints the message " I have no sides".
*
*/
//Your code goes here
public class Circle extends Shape {
public Circle(String myName) {
super(myName);
}
public void printMessage() {
System.out.println("I have no sides");
}
}
/**
*
* Write a Rectangle class that extends the Shape class. Do the following:
* 1. Write the class header.
* 2. Write a default constructor that initializes the name of the Rectangle object to "Rectangle".
* You will need to call the constructor of the parent Shape class.
* 3. Write a void method printMessage() that prints the message "I am a 4 sided figure".
*
*/
//Your code goes here
public class Rectangle extends Shape {
public Rectangle(String myName) {
super(myName);
}
public void printMessage() {
System.out.println("I am a 4 sided figure");
}
}
/**
*
* Write a Shape class. Do the following:
* 1. Write a class header for the Shape class.
* 2. Create a String attribute that holds the name of the Shape object. Use the keyword String to
* declare a variable of your choice.
* 3. Write a parameterized constructor that initializes the name of the Shape object.
* 4. Write a void method printName() that prints the name of the Shape object.
*
*/
//Your code goes here
public class Shape {
public Shape(String myName) {
}
public void printName(String myName) {
System.out.println("I am a " + myName);
}
}
/**
* In this lab you will practice inheritance You will need
* to use System.out.println (String) to print messages to the console.
*
* Write a driver class to test the methods of the Shape class and it's sub classes
* Do the following:
* 1. Create a Shape object called "Irregular shape". Call it myShape.
* 2. Print myShape's name.
* 3. Create a Rectangle object called "Rectangle". Call it myRect.
* 4. Print myRect's name.
* 5. Print myRect's message.
* 6. Create a Square object. Call it mySqr.
* 7. Print mySqr's name.
* 8. Print mySqr's message.
* 9. Create a Circle object. Call it myCircle.
* 7. Print myCircle's name.
* 8. Print myCircle's message.
*
*/
public class ShapesDriver {
public static void main(String[] args) {
Shape myShape = new Shape("Irregular Shape");
myShape.printName("Irregular Shape");
Rectangle myRect = new Rectangle("Rectangle");
myRect.printName("Rectangle");
myRect.printMessage();
Square mySqr = new Square("Rectangle");
mySqr.printName("Rectangle");
mySqr.printMessage();
Circle myCircle = new Circle("Circle");
myCircle.printName("Circle");
myCircle.printMessage();
}
}
/**
* Write a Square class that extends the Rectangle class. Do the following:
* 1. Write the class header.
* 2. Write a default constructor that initializes the name of the Square object to "Rectangle".
* You will need to call the constructor of the parent Rectangle class.
* 3. Write a void method printMessage() that prints the message
* "I am a 4 sided figure
* I have equal sides"
* You must call the parent's printMessage() method using super.printMessage().
*
*/
//Your code goes here
public class Square extends Rectangle {
public Square(String myName) {
super(myName);
}
public void printMessage() {
System.out.println("I am a 4 sided figure \n I have equal sides");
}
}

Step by step
Solved in 3 steps

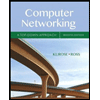
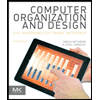
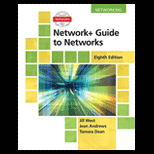
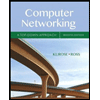
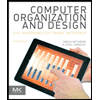
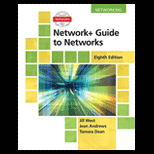
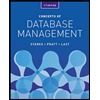
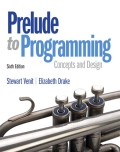
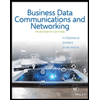