Instructions: Create an application that lets you enter a new customer or a new employee. Console Welcome to the Person Manager Create customer or employee? (c/e): Error! This entry is required. Try again. Create customer or employee? (c/e): p Error! Entry must be 'c' or 'e'. Try again. Create customer or employee? (c/e): c First name: Steve Last name: Trevor Customer number: M10293 You entered a new Customer: Name: Steve Trevor Customer Number: M10293 Continue? (y/n): y Create customer or employee? (c/e): e First name: Diana Last name: Prince SSN: 111-22-3333 You entered a new Employee: Name: Diana Prince SSN: xxx-xx-3333 Continue? (y/n): OK Error! Entry must be 'y' or 'n'. Try again. Continue? (y/n): n Specifications Create a class named Person with these constructors and methods: public Person(String first, String last) public String getFirstName() public void setFirstName(String first) public String getLastName() public void setLastName() The Person class should override the toString() method so it returns the first name and last name in this format: Name: Frank Jones Create a class named Customer that inherits the Person class and contains these constructors and methods: public Customer(String first, String last, String number) public String getCustomerNumber() public void setCustomerNumber(String number) The Customer class should override the toString() method so it returns the value returned by the toString() method of the Person class appended with the customer number, like this: Name: Frank Jones Customer Number: J54128 Create a class named Employee that inherits the Person class and contains these constructors and methods: public Employee(String first, String last, String ssn) public String getSsn() public void setSsn(String ssn) The getSsn() method should return a masked version of the social security number that only reveals the last four numbers. The Employee class should override the toString() method so it returns the value returned by the toString() method of the Person class appended with the social security number, like this: Name: Frank Jones SSN: xxx-xx-1111 [Hint: toString() can invoke getSsn() ] My code: import java.util.Scanner; public class Nic_Finley_Project82 { public static void main(String[] args) { Scanner sc = new Scanner(System.in); Console.displayWelcomeMessage("Person Manager"); do { System.out.print("Create customer or employee? (c/e): "); String fName = Console.getRequiredString(sc, "First name: "); String lName = Console.getRequiredString(sc, "Last name: "); String cNum = Console.getRequiredString(sc, "Customer Number: "); Customer c = new Customer(fName, lName, cNum); System.out.println("You entered a new Customer:"); System.out.println(c); } while (Console.getChoiceString(sc, "Continue? (y/n): ", "y", "n").equals("y")); } } person class: public class Person { private String firstName; private String lastName; public Person(String first, String last) { this.firstName = first; this.lastName = last; } //getter and setter methods public String getFirstName() { return firstName; } public void setFirstName(String x) { this.firstName = x; } public String getLastName() { return lastName; } public void setLastName(String x) { this.lastName = x; } @Override public String toString() { return "Name: " + firstName + " " + lastName; } } customer class: public class Customer extends Person { private String cNum; public Customer(String first, String last, String number) { super(first, last); this.cNum = number; } public String getCustomerNumber() { return cNum; } public void setCustomerNumber(String number) { this.cNum = number; } @Override public String toString() { return super.toString() + "\nCustomer Number: " + cNum; } } Console class: import java.util.Scanner; public class Console { //get specified string. public static String getRequiredString(Scanner sc, String prompt) { String s = ""; boolean isValid = false; while (!isValid) { System.out.print(prompt); s = sc.nextLine(); if (s.equals("")) { System.out.println("Error! This entry is required. Try again."); } else { isValid = true; } } return s; } //get specifeied string. public static String getChoiceString(Scanner sc, String prompt, String s1, String s2) { String s = ""; boolean isValid = false; while (!isValid) { s = getRequiredString(sc, prompt); if (!s.equalsIgnoreCase(s1) && !s.equalsIgnoreCase(s2)) { System.out.println("Error! Entry must be '" + s1 + "' or '" + s2 + "'. Try again."); } else { isValid = true; } } return s; } public static void displayWelcomeMessage(String name) { System.out.println("Welcome to the " + name); } } employee class: public class Employee extends Person { String ssn; public Employee(String first, String last, String ssn) { super(first, last); this.ssn = ssn; } public String getSsn() { return "xxx-xx-" + ssn; } public void setSsn(String ssn) { this.ssn = ssn; } @Override public String toString() { return super.toString() + "\nSSN: " + this.getSsn(); } } I need help implementing the code from the employee class so it works with the main program.
Instructions: Create an application that lets you enter a new customer or a new employee.
Console
Welcome to the Person Manager
Create customer or employee? (c/e):
Error! This entry is required. Try again.
Create customer or employee? (c/e): p
Error! Entry must be 'c' or 'e'. Try again.
Create customer or employee? (c/e): c
First name: Steve
Last name: Trevor
Customer number: M10293
You entered a new Customer:
Name: Steve Trevor
Customer Number: M10293
Continue? (y/n): y
Create customer or employee? (c/e): e
First name: Diana
Last name: Prince
SSN: 111-22-3333
You entered a new Employee:
Name: Diana Prince
SSN: xxx-xx-3333
Continue? (y/n): OK
Error! Entry must be 'y' or 'n'. Try again.
Continue? (y/n): n
Specifications
Create a class named Person with these constructors and methods:
public Person(String first, String last)
public String getFirstName()
public void setFirstName(String first)
public String getLastName()
public void setLastName()
The Person class should override the toString() method so it returns the first name and last name in this format:
Name: Frank Jones
Create a class named Customer that inherits the Person class and contains these constructors and methods:
public Customer(String first, String last, String number)
public String getCustomerNumber()
public void setCustomerNumber(String number)
The Customer class should override the toString() method so it returns the value returned by the toString() method of the Person class appended with the customer number, like this:
Name: Frank Jones
Customer Number: J54128
Create a class named Employee that inherits the Person class and contains these constructors and methods:
public Employee(String first, String last, String ssn)
public String getSsn()
public void setSsn(String ssn)
The getSsn() method should return a masked version of the social security number that only reveals the last four numbers.
The Employee class should override the toString() method so it returns the value returned by the toString() method of the Person class appended with the social security number, like this:
Name: Frank Jones
SSN: xxx-xx-1111
[Hint: toString() can invoke getSsn() ]
My code:
import java.util.Scanner;
public class Nic_Finley_Project82 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Console.displayWelcomeMessage("Person Manager");
do {
System.out.print("Create customer or employee? (c/e): ");
String fName = Console.getRequiredString(sc, "First name: ");
String lName = Console.getRequiredString(sc, "Last name: ");
String cNum = Console.getRequiredString(sc, "Customer Number: ");
Customer c = new Customer(fName, lName, cNum);
System.out.println("You entered a new Customer:");
System.out.println(c);
} while (Console.getChoiceString(sc, "Continue? (y/n): ", "y", "n").equals("y"));
}
}
person class:
public class Person {
private String firstName;
private String lastName;
public Person(String first, String last) {
this.firstName = first;
this.lastName = last;
}
//getter and setter methods
public String getFirstName() {
return firstName;
}
public void setFirstName(String x) {
this.firstName = x;
}
public String getLastName() {
return lastName;
}
public void setLastName(String x) {
this.lastName = x;
}
@Override
public String toString() {
return "Name: " + firstName + " " + lastName;
}
}
customer class:
public class Customer extends Person {
private String cNum;
public Customer(String first, String last, String number) {
super(first, last);
this.cNum = number;
}
public String getCustomerNumber() {
return cNum;
}
public void setCustomerNumber(String number) {
this.cNum = number;
}
@Override
public String toString() {
return super.toString() + "\nCustomer Number: " + cNum;
}
}
Console class:
import java.util.Scanner;
public class Console {
//get specified string.
public static String getRequiredString(Scanner sc, String prompt) {
String s = "";
boolean isValid = false;
while (!isValid) {
System.out.print(prompt);
s = sc.nextLine();
if (s.equals("")) {
System.out.println("Error! This entry is required. Try again.");
} else {
isValid = true;
}
}
return s;
}
//get specifeied string.
public static String getChoiceString(Scanner sc, String prompt,
String s1, String s2) {
String s = "";
boolean isValid = false;
while (!isValid) {
s = getRequiredString(sc, prompt);
if (!s.equalsIgnoreCase(s1) && !s.equalsIgnoreCase(s2)) {
System.out.println("Error! Entry must be '" + s1 + "' or '" + s2 + "'. Try again.");
} else {
isValid = true;
}
}
return s;
}
public static void displayWelcomeMessage(String name) {
System.out.println("Welcome to the " + name);
}
}
employee class:
public class Employee extends Person {
String ssn;
public Employee(String first, String last, String ssn) {
super(first, last);
this.ssn = ssn;
}
public String getSsn() {
return "xxx-xx-" + ssn;
}
public void setSsn(String ssn) {
this.ssn = ssn;
}
@Override
public String toString() {
return super.toString() + "\nSSN: " + this.getSsn();
}
}
I need help implementing the code from the employee class so it works with the main program.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

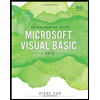
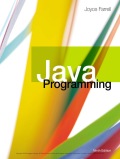
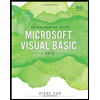
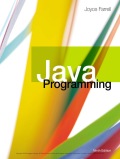