Instructions : - Create modules and functions - Pass data from one function to another - Store data in lists - Create. and use selection control structures - Create. and use iterative control structures - Add comments to Python code This program creates a class registration system. It allows students to log in to add courses, drop courses and list courses they have registered for. This program has 5 functions in 2 modules: a student module and a main module. You must define the following functions in the student module. [ instructions1.png] [instructions2.png] This program uses a few lists to store data. To make grading easier, data will be added to these lists at the beginning of the main function. student_list = [('1001', '111'), ('1002', '222'), ('1003', '333'), ('1004', '444')] course_list = ['CSC101', 'CSC102', 'CSC103'] max_size_list = [3, 2, 1] roster_list = [['1004', '1003'], ['1001'], ['1002']] There are 4 students in this program. ID and PIN of students are stored as tuples in student_list. The first element of each tuple is student ID, while the second element is PIN. Three courses are offered. The course codes are stored in course_list. These courses are CSC101, CSC102 and CSC103. The maximum class size of the courses offered are stored in max_size_list. The max sizes of CSC101, CSC102 and CSC103 are 3, 2 and 1, respectively. Rosters of the three classes offered are stored as three lists, which are three elements of roster_list, which is actually a list of lists. Students 1004 and 1003 are enrolled in CSC101. Student 1001 is enrolled in CSC102. Student 1002 is enrolled in CSC103. The program should have a loop to create multiple student sessions. In each session, ask user to enter ID, then call the login function to verify the student's identity. If login is successful, use a loop to allow the student to add courses, drop courses and list courses registered. The following is an example. Enter ID to log in, or 0 to quit: 1234 Enter PIN: 123 ID or PIN incorrect Enter ID to log in, or 0 to quit: 1001 Enter PIN: 111 ID and PIN verified Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC121 Course not found Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC102 You are already enrolled in that course. Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC103 Course already full. Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC101 Course added Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2 Enter course you want to drop: CSC121 Course not found Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2 Enter course you want to drop: CSC103 You are not enrolled in that course. Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2 Enter course you want to drop: CSC102 Course dropped Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3 Courses registered: CSC101 Total number: 1 Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC102 Course added Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3 Courses registered: CSC101 CSC102 Total number: 2 Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 0 Session ended. Enter ID to log in, or 0 to quit: 1002 Enter PIN: 222 ID and PIN verified Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3 Courses registered: CSC103 Total number: 1 Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC101 Course already full. Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1 Enter course you want to add: CSC102 Course added Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3 Courses registered: CSC102 CSC103 Total number: 2 Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 0 Session ended. Enter ID to log in, or 0 to quit: 0
I have to write a program that does the following but for some reason it doesn't work, and I'm not quite sure where the error is:
Instructions :
- Create modules and functions
- Pass data from one function to another
- Store data in lists
- Create. and use selection control structures
- Create. and use iterative control structures
- Add comments to Python code
This program creates a class registration system. It allows students to log in to add courses, drop courses and list courses they have registered for.
This program has 5 functions in 2 modules: a student module and a main module.
You must define the following functions in the student module.
[ instructions1.png]
[instructions2.png]
This program uses a few lists to store data. To make grading easier, data will be added to these lists at the beginning of the main function.
student_list = [('1001', '111'), ('1002', '222'), ('1003', '333'), ('1004', '444')] course_list = ['CSC101', 'CSC102', 'CSC103'] max_size_list = [3, 2, 1] roster_list = [['1004', '1003'], ['1001'], ['1002']]
There are 4 students in this program. ID and PIN of students are stored as tuples in student_list. The first element of each tuple is student ID, while the second element is PIN.
Three courses are offered. The course codes are stored in course_list. These courses are CSC101, CSC102 and CSC103.
The maximum class size of the courses offered are stored in max_size_list. The max sizes of CSC101, CSC102 and CSC103 are 3, 2 and 1, respectively.
Rosters of the three classes offered are stored as three lists, which are three elements of roster_list, which is actually a list of lists. Students 1004 and 1003 are enrolled in CSC101. Student 1001 is enrolled in CSC102. Student 1002 is enrolled in CSC103.
The program should have a loop to create multiple student sessions. In each session, ask user to enter ID, then call the login function to verify the student's identity. If login is successful, use a loop to allow the student to add courses, drop courses and list courses registered.
The following is an example.
Enter ID to log in, or 0 to quit: 1234
Enter PIN: 123
ID or PIN incorrect
Enter ID to log in, or 0 to quit: 1001
Enter PIN: 111
ID and PIN verified
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC121
Course not found
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC102
You are already enrolled in that course.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC103
Course already full.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC101
Course added
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2
Enter course you want to drop: CSC121
Course not found
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2
Enter course you want to drop: CSC103
You are not enrolled in that course.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2
Enter course you want to drop: CSC102
Course dropped
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC101
Total number: 1
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC102
Course added
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC101
CSC102
Total number: 2
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 0
Session ended.
Enter ID to log in, or 0 to quit: 1002
Enter PIN: 222
ID and PIN verified
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC103
Total number: 1
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC101
Course already full.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC102
Course added
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC102
CSC103
Total number: 2
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 0
Session ended.
Enter ID to log in, or 0 to quit: 0
Files:
registration.py
https://drive.google.com/file/d/11Gz2VFouvyOyrOSnv3fNEPYIa_E7OubA/view?usp=sharing
student.py
https://drive.google.com/file/d/1TbmxJSPh3ax3bbcV6xq81O96CWO07u84/view?usp=sharing
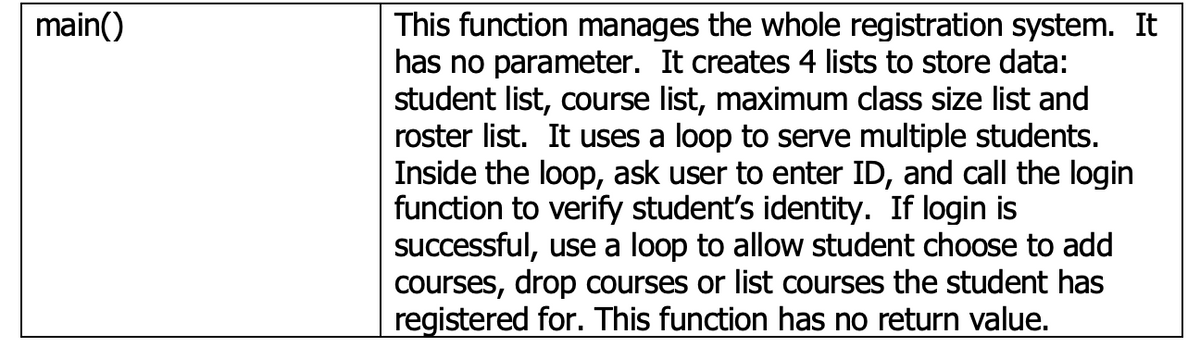
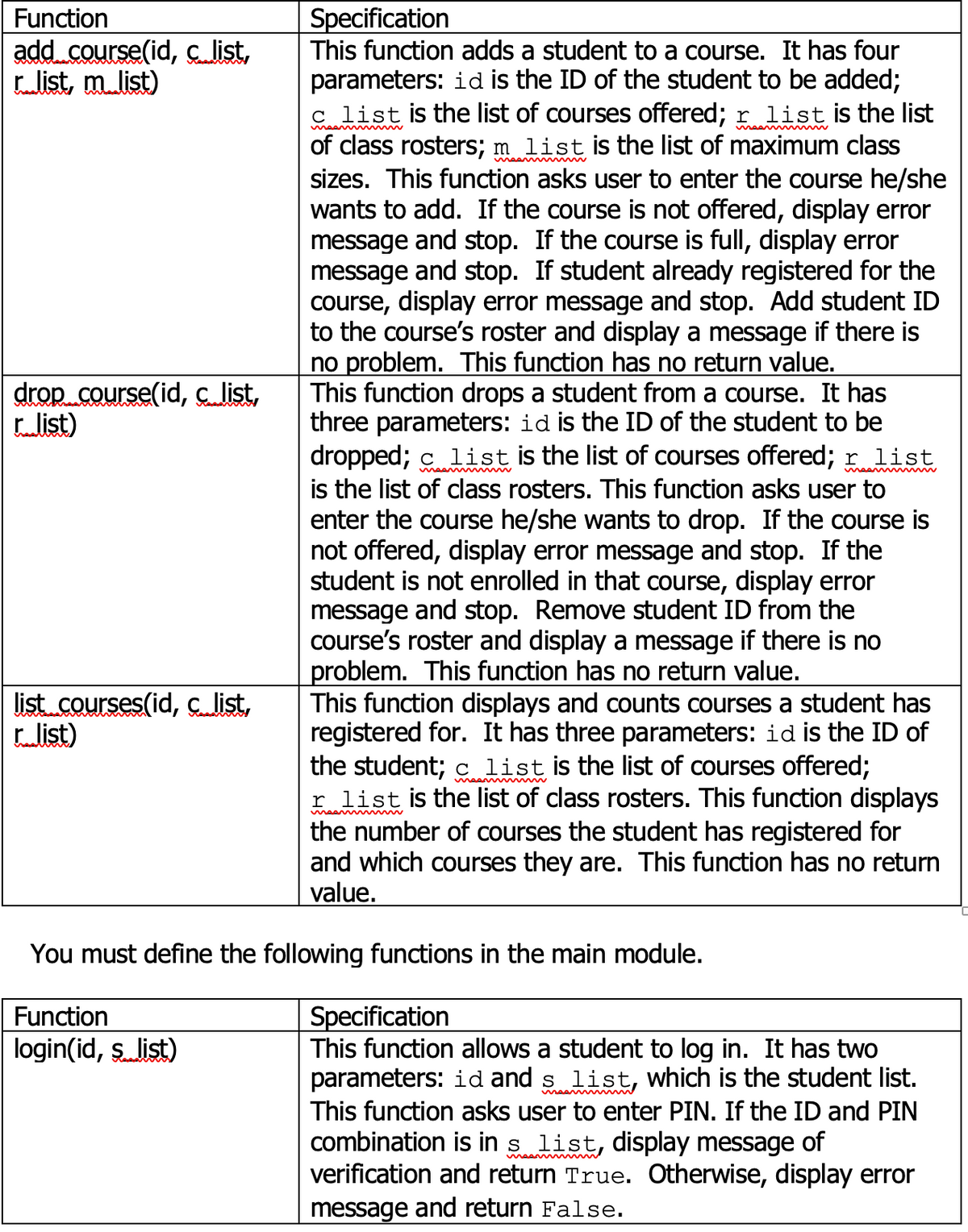

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

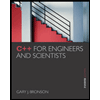
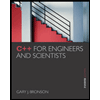