Instructions Write a program to illustrate how to use the class temporary, designed in Exercises 13 and _14_ of this chapter. Your program should not use the statements given in Exercises 15 and _ 16_. Also, your program must contain statements that would ask the user to enter data of an object and use the member function set to initialize the object. The header file for the class temporary from Exercise 13 has been provided. Must include 3 files (and what is already provided): 1. Main.cpp #include using namespace std; int main() { // Write your main here return 0; } 2. temporary.h #include using namespace std; class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst() const; double getSecond() const; temporary(string = "", double = 0, double = 0); private: string description; double first; double second; }; 3. temporaryImp.cpp Test Contents TEST(Shapes, 1) { testing::internal::CaptureStdout(); temporary myObject; temporary newObject("rectangle", 8.5, 3.9); string objectName; cout << fixed << showpoint << setprecision(2); myObject.print(); newObject.print(); myObject.set("circle", 5, 0); cout << endl << "After setting myObject: " << endl; myObject.print(); std::string output = testing::internal::GetCapturedStdout(); ASSERT_EQ(output, " -- invalid shape.\nrectangle: length = 8.50, width = 3.90, area = 33.15\n\nAfter setting myObject: \ncircle: radius = 5.00, area = 78.54\n");}
Instructions
Write a
The header file for the class temporary from Exercise 13 has been provided.
Must include 3 files (and what is already provided):
1. Main.cpp
#include <iostream>
using namespace std;
int main() {
// Write your main here
return 0;
}
2. temporary.h
#include <string>
using namespace std;
class temporary
{
public:
void set(string, double, double);
void print();
double manipulate();
void get(string&, double&, double&);
void setDescription(string);
void setFirst(double);
void setSecond(double);
string getDescription() const;
double getFirst() const;
double getSecond() const;
temporary(string = "", double = 0, double = 0);
private:
string description;
double first;
double second;
};
3. temporaryImp.cpp
Test Contents
TEST(Shapes, 1) {
testing::internal::CaptureStdout();
temporary myObject;
temporary newObject("rectangle", 8.5, 3.9);
string objectName;
cout << fixed << showpoint << setprecision(2);
myObject.print();
newObject.print();
myObject.set("circle", 5, 0);
cout << endl << "After setting myObject: " << endl;
myObject.print();
std::string output = testing::internal::GetCapturedStdout();
ASSERT_EQ(output, " -- invalid shape.\nrectangle: length = 8.50, width = 3.90, area = 33.15\n\nAfter setting myObject: \ncircle: radius = 5.00, area = 78.54\n");}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

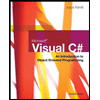
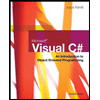