Intro to Programming in C-program 6 (smaller program) Assignment purpose: To compile, build, and execute an interactive program using functions from stdio.h (printf and scanf), simple math in C, conditions, a simple loop, and programmer defined functions, with pass by copy input parameters and with input/output parameters.
Intro to Programming in C-program 6 (smaller program) Assignment purpose: To compile, build, and execute an interactive program using functions from stdio.h (printf and scanf), simple math in C, conditions, a simple loop, and programmer defined functions, with pass by copy input parameters and with input/output parameters.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.1: Function And Parameter Declarations
Problem 11E
Related questions
Question
I need help making this C
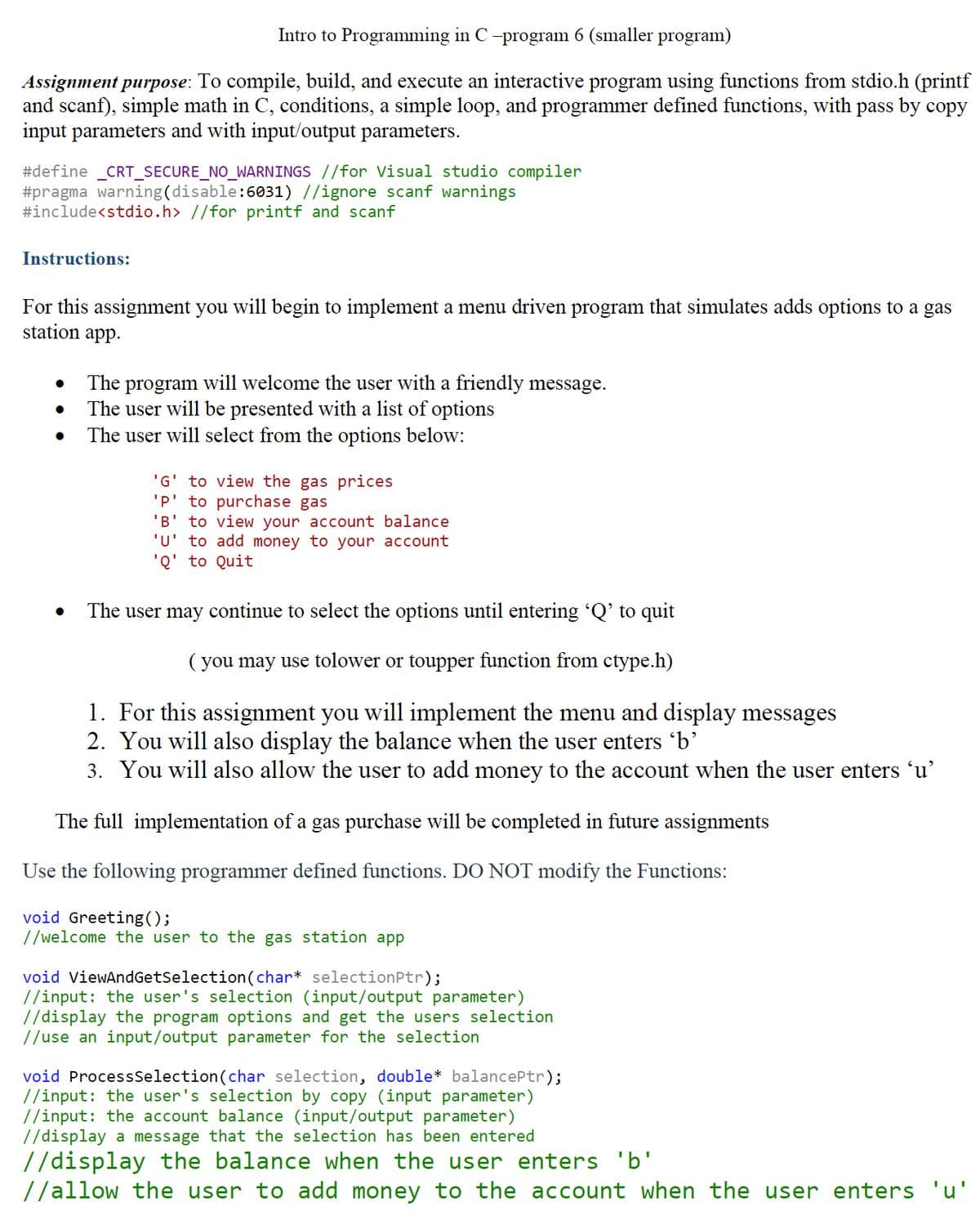
Transcribed Image Text:Intro to Programming in C -program 6 (smaller program)
Assignment purpose: To compile, build, and execute an interactive program using functions from stdio.h (printf
and scanf), simple math in C, conditions, a simple loop, and programmer defined functions, with pass by copy
input parameters and with input/output parameters.
#define _CRT_SECURE_NO_WARNINGS //for Visual studio compiler
#pragma warning (disable:6031) //ignore scanf warnings
#include<stdio.h> //for printf and scanf
Instructions:
For this assignment you will begin to implement a menu driven program that simulates adds options to a gas
station app.
The program will welcome the user with a friendly message.
The user will be presented with a list of options
The user will select from the options below:
'G' to view the gas prices
'P' to purchase gas
'B' to view your account balance
'u' to add money to your account
'Q' to Quit
The user may continue to select the options until entering 'Q' to quit
( you may use tolower or toupper function from ctype.h)
1. For this assignment you will implement the menu and display messages
2. You will also display the balance when the user enters 'b'
3. You will also allow the user to add money to the account when the user enters 'u’
The full implementation of a gas purchase will be completed in future assignments
Use the following programmer defined functions. DO NOT modify the Functions:
void Greeting();
//welcome the user to the gas station app
void ViewAndGetSelection(char* selectionPtr);
//input: the user's selection (input/output parameter)
//display the program options and get the users selection
//use an input/output parameter for the selection
void ProcessSelection(char selection, double* balancePtr);
//input: the user's selection by copy (input parameter)
//input: the account balance (input/output parameter)
//display a message that the selection has been entered
//display the balance when the user enters 'b'
//allow the user to add money to the account when the user enters 'u'
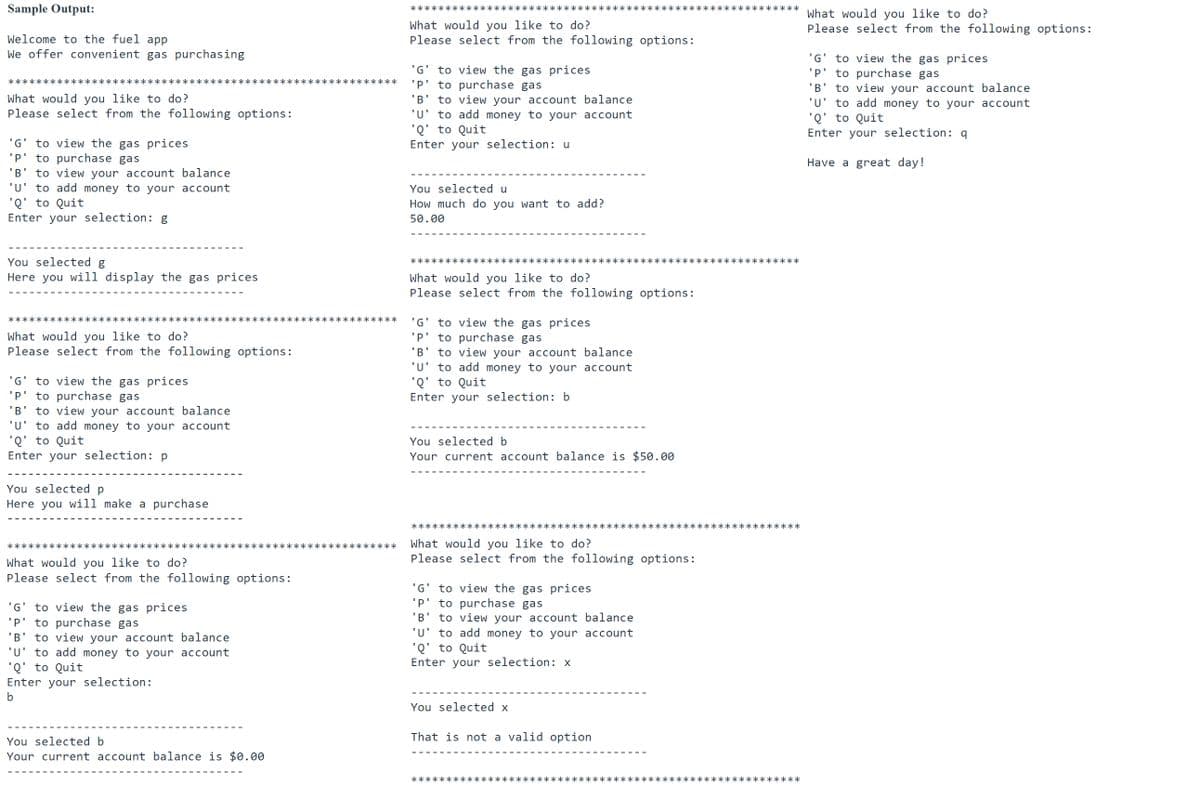
Transcribed Image Text:Sample Output:
**
What would you like to do?
Please select from the following options:
What would you like to do?
Please select from the following options:
Welcome to the fuel app
We offer convenient gas purchasing
to view the gas prices
to purchase gas
to view your account balance
'u' to add money to your account
'Q' to Quit
Enter your selection: q
'G
'G' to view the gas prices
'P' to purchase gas
'B' to view your account balance
'u' to add money to your account
'Q' to Quit
Enter your selection: u
'P
********:
******
********************
'B
What would you like to do?
Please select from the following options:
'G' to view the gas prices
'P' to purchase gas
B' to view your account balance
'u' to add money to your account
'Q' to Quit
Enter your selection: g
Have a great day!
You selected u
How much do you want to add?
50.00
You selected g
******
Here you will display the gas prices
What would you like to do?
Please select from the following options:
********
**********
*******
to view the gas prices
'P' to purchase gas
to view your account balance
'u' to add money to your account
'Q' to Quit
Enter your selection: b
く
'G
What would you like to do?
Please select from the following options:
'B
'G' to view the gas prices
'P' to purchase gas
'B' to view your account balance
'U' to add money to your account
'Q' to Quit
Enter your selection: p
You selected b
Your current account balance is $50.00
You selected p
Here you will make a purchase
******:
*****************
**********>
*********
What would you like to do?
Please select from the following options:
*********
********
**********************
What would you like to do?
Please select from the following options:
'G' to view the gas prices
'P' to purchase gas
'B' to view your account balance
'u' to add money to your account
'Q' to Quit
Enter your selection:
'G' to view the gas prices
'P' to purchase gas
to view your account balance
'u' to add money to your account
'Q' to Quit
Enter your selection: x
'B
b
You selected x
That is not a valid option
You selected b
Your current account balance is $0.00
**********
************
*********
*******
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
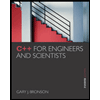
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
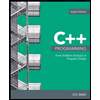
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
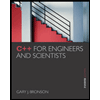
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
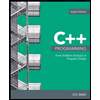
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning