Introduction For this assignment, you are to create a program in Java that asks the user to enter the size of a shape (must be odd), then displays a menu of 4 options. Options 1-3 will print various shapes: closed square, open square, and diamond. Option 4 will allow the user to quit the program. You are to work on this assignment independently. Program Design You should have a single class called Shapes. You will have 5 static methods: main, printOpenSquare, printClosedSquare, printDiamond, and getShapeSize. The printOpenSquare, printClosedSquare, and printDiamond methods should take as input an int parameter called size and print the corresponding shape of the given size. (see sample output at the end) The getShapeSize method should ask the user to enter a number for the size of the shape. This number needs to be an ODD integer. If the user enters a number that is not odd, ask the user to reenter the number until it is odd. Then, the method should return that odd number. The main method should do the following: Enter a loop in which you will display a menu of choices (1-4) and wait for user input (see example at the end). The choices are: "1. Print closed square", "2. Print open square","3. Print diamond", and "4. Quit program". If option 4 is selected, quit the program and print "Good bye! ". Otherwise, ask the user to enter a number for the size of the shape by calling the getShapeSize method. Then, print the corresponding shape with dimensions = size x size, by calling the appropriate method. The exception is the diamond shape, which will have size rows and size+1 columns. See example for sample output. Additional Requirements 1. The name of your Java Class that contains the main method should be Shapes. All your code should be within a single file. 2. Your code should follow good coding practices, including good use of whitespace (indents and line breaks) and use of both inline and block comments. You need to use meaningful identifier names that conform to standard Java naming conventions. At the top of the file, you need to put in a block comment with the following information: your name, date, course name, semester, and assignment name. 5. Your program needs to handle invalid inputs gracefully. For example, entering a number outside of a valid range should not crash the program. Instead, the user should be prompted to enter the number again. 6. The output of your program should exactly match the sample program output given at the end. 3. 4. What to Turn In You will turn in the single Shapes.java file using BlackBoard. What You Need to Know for This Assignment Conditional statements Loops Getting user input Creating and calling methods
Introduction For this assignment, you are to create a program in Java that asks the user to enter the size of a shape (must be odd), then displays a menu of 4 options. Options 1-3 will print various shapes: closed square, open square, and diamond. Option 4 will allow the user to quit the program. You are to work on this assignment independently. Program Design You should have a single class called Shapes. You will have 5 static methods: main, printOpenSquare, printClosedSquare, printDiamond, and getShapeSize. The printOpenSquare, printClosedSquare, and printDiamond methods should take as input an int parameter called size and print the corresponding shape of the given size. (see sample output at the end) The getShapeSize method should ask the user to enter a number for the size of the shape. This number needs to be an ODD integer. If the user enters a number that is not odd, ask the user to reenter the number until it is odd. Then, the method should return that odd number. The main method should do the following: Enter a loop in which you will display a menu of choices (1-4) and wait for user input (see example at the end). The choices are: "1. Print closed square", "2. Print open square","3. Print diamond", and "4. Quit program". If option 4 is selected, quit the program and print "Good bye! ". Otherwise, ask the user to enter a number for the size of the shape by calling the getShapeSize method. Then, print the corresponding shape with dimensions = size x size, by calling the appropriate method. The exception is the diamond shape, which will have size rows and size+1 columns. See example for sample output. Additional Requirements 1. The name of your Java Class that contains the main method should be Shapes. All your code should be within a single file. 2. Your code should follow good coding practices, including good use of whitespace (indents and line breaks) and use of both inline and block comments. You need to use meaningful identifier names that conform to standard Java naming conventions. At the top of the file, you need to put in a block comment with the following information: your name, date, course name, semester, and assignment name. 5. Your program needs to handle invalid inputs gracefully. For example, entering a number outside of a valid range should not crash the program. Instead, the user should be prompted to enter the number again. 6. The output of your program should exactly match the sample program output given at the end. 3. 4. What to Turn In You will turn in the single Shapes.java file using BlackBoard. What You Need to Know for This Assignment Conditional statements Loops Getting user input Creating and calling methods
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Use java and please read the "Additional Requirements" section. This covers indentation, formatting, and comments (both block and inline comments).
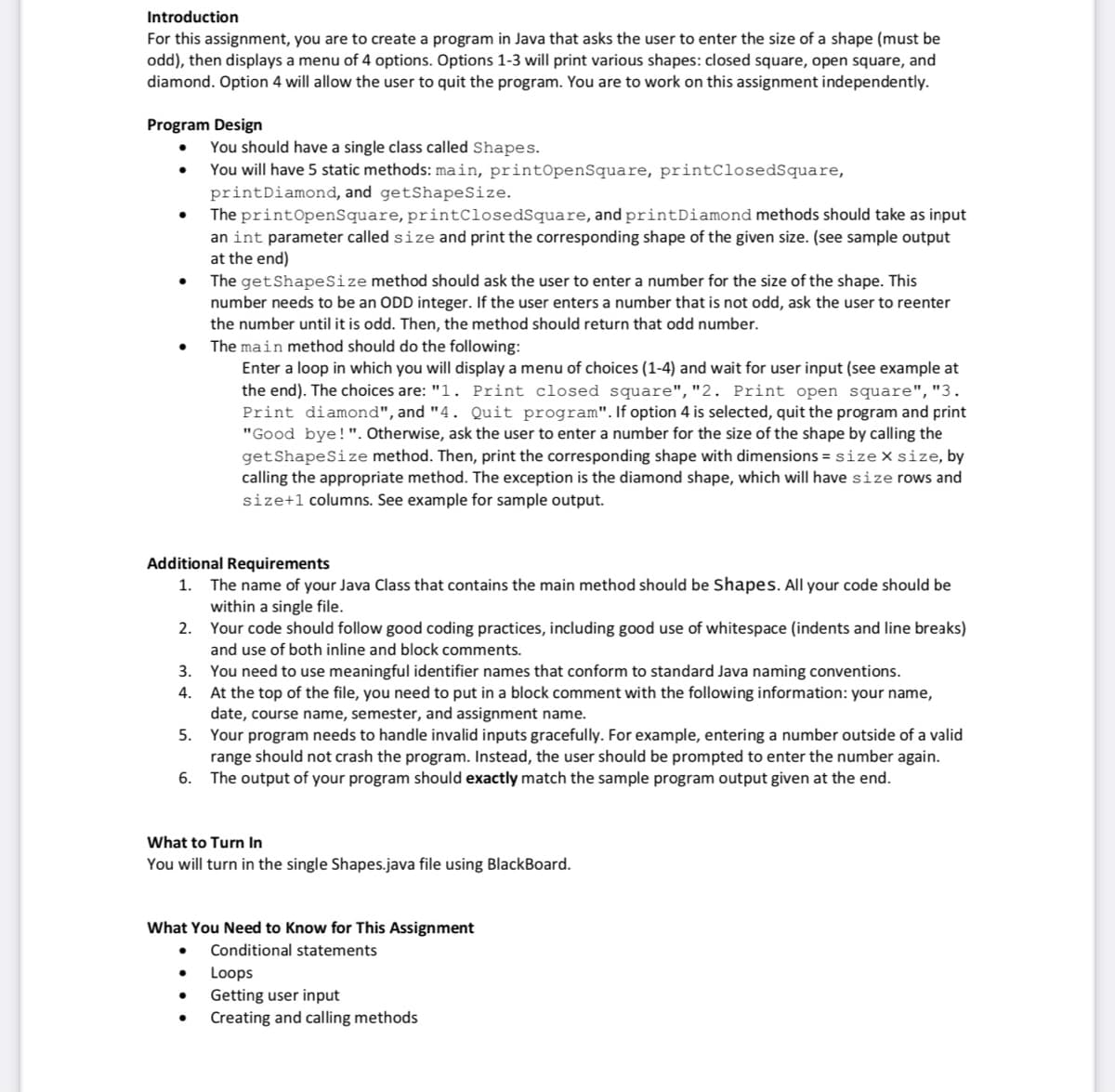
Transcribed Image Text:Introduction
For this assignment, you are to create a program in Java that asks the user to enter the size of a shape (must be
odd), then displays a menu of 4 options. Options 1-3 will print various shapes: closed square, open square, and
diamond. Option 4 will allow the user to quit the program. You are to work on this assignment independently.
Program Design
You should have a single class called Shapes.
You will have 5 static methods: main, printOpenSquare, printClosedSquare,
printDiamond, and getShapeSize.
The printOpenSquare, printClosedSquare, and printDiamond methods should take as input
an int parameter called size and print the corresponding shape of the given size. (see sample output
at the end)
The getShapeSize method should ask the user to enter a number for the size of the shape. This
number needs to be an ODD integer. If the user enters a number that is not odd, ask the user to reenter
the number until it is odd. Then, the method should return that odd number.
The main method should do the following:
Enter a loop in which you will display a menu of choices (1-4) and wait for user input (see example at
the end). The choices are: "1. Print closed square","2. Print open square", "3.
Print diamond", and "4. Quit program".If option 4 is selected, quit the program and print
"Good bye!". Otherwise, ask the user to enter a number for the size of the shape by calling the
getShapeSize method. Then, print the corresponding shape with dimensions = size Xsize, by
calling the appropriate method. The exception is the diamond shape, which will have size rows and
size+1 columns. See example for sample output.
Additional Requirements
The name of your Java Class that contains the main method should be Shapes. All your code should be
within a single file.
2. Your code should follow good coding practices, including good use of whitespace (indents and line breaks)
and use of both inline and block comments.
1.
3. You need to use meaningful identifier names that conform to standard Java naming conventions.
At the top of the file, you need to put in a block comment with the following information: your name,
date, course name, semester, and assignment name.
Your program needs to handle invalid inputs gracefully. For example, entering a number outside of a valid
range should not crash the program. Instead, the user should be prompted to enter the number again.
The output of your program should exactly match the sample program output given at the end.
4.
5.
6.
What to Turn In
You will turn in the single Shapes.java file using BlackBoard.
What You Need to Know for This Assignment
Conditional statements
Loops
Getting user input
Creating and calling methods
![Sample Program Output
Programming Fundamentals
NAME: [put your name here]
PROGRAMMING ASSIGNMENT 2
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 1
Enter the size of the figure (odd number): 8
Invalid figure size - must be an odd number
Renter the size of the figure: 5
XXXXX
XXXXX
XXXXX
XXXXX
XXXXX
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 2
Enter the size of the figure (odd number): 7
XXXXXXX
X
X
X
X
XXXXXXX
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 3
Enter the size of the figure (odd number): 9
XX
X X
X
X X
XX
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 4
Good bye!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc82e38b1-10c9-42a1-8eb0-c4b9d75b117c%2Fa8c2e79b-ab52-454f-9b30-5c927fade83d%2Fk8inad_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Sample Program Output
Programming Fundamentals
NAME: [put your name here]
PROGRAMMING ASSIGNMENT 2
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 1
Enter the size of the figure (odd number): 8
Invalid figure size - must be an odd number
Renter the size of the figure: 5
XXXXX
XXXXX
XXXXX
XXXXX
XXXXX
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 2
Enter the size of the figure (odd number): 7
XXXXXXX
X
X
X
X
XXXXXXX
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 3
Enter the size of the figure (odd number): 9
XX
X X
X
X X
XX
MENU:
1. Print closed square
2. Print open square
3. Print diamond
4. Quit program
Please select an option: 4
Good bye!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Recommended textbooks for you
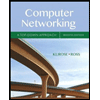
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
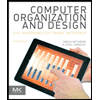
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
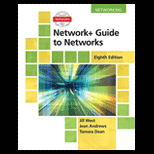
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
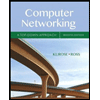
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
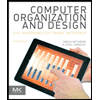
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
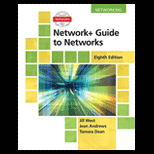
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
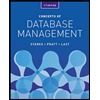
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
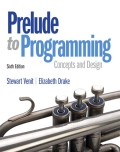
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
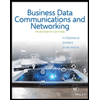
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY