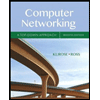
PLEASE USE PYTHON PROGRAMMING
Create a class called Numbers, which has a single class attribute called MULTIPLIER, and a constructor which takes the parameters x and y (these should all be numbers).
- Write a method called add which returns the sum of the attributes x and y.
- Write a class method called multiply, which takes a single number parameter aand returns the product of a and MULTIPLIER.
- Write a static method called subtract, which takes two number parameters, b and c, and returns b - c.
- Write a method called value which returns a tuple containing the values of x and y. Make this method into a property, and write a setter and a deleter for manipulating the values of x and y.
class Numbers:
# TODO: create a class attribute called MULTIPLIER
def __init__(self, x, y):
self.x = x
self.y = y
def add(self):
# TODO: return x + y
def multiply(self, a):
# TODO: return the attribute MULTIPLIER * by a.
@staticmethod
def subtract(b, c):
# TODO:
# TODO: Make this a property
def value(self):
return self.x, self.y
# TODO: Create a setter and a deleter for value.
# test the class.
num = Numbers(5,6)
print(num.add())
print(num.multiply(2))
print(num.subtract(4, 4))

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Answer needs to be in java thanksarrow_forwardWrite in Javaarrow_forwardPython:Please read the following. The Game class The Game class brings together an instance of the GameBoard class, a list of Player objects who are playing the game, and an integer variable “n” indicating how many tokens must be aligned to win (as in the name “Connect N”). The game class has a constructor and two additional methods. The most complicated of these, play, is provided for you and should not be modified. However, you must implement the constructor and the add_player method. __init__(self,n,height,width) The Game constructor needs to initialize three instance variables. The first, self.n, simply holds the integer value “n” provided as a parameter. The second, self.board, should be a new instance of the GameBoard class (described below), built using the provided height and width as dimensions. The third, self.players, should be an initially empty list. add_player(self,name,symbol) The add_player method is provided a name string and a symbol string. These two values should be…arrow_forward
- Write a class that contains the following two methods: /** Convert from Celsius to Fahrenheit */ public static double celsiusToFahrenheit(double celsius) /** Convert from Fahrenheit to Celsius */ public static double fahrenheitToCelsius(double fahrenheit) The formula for the conversion is as follows: fahrenheit = (9.0 / 5) * celsius + 32 celsius = (5.0 / 9) * (fahrenheit – 32) Write a test program that invokes these methods to display the following table: NOTE: Celsius starts at 40 and should be converted to Fahrenheit, on the same line Fahrenheit starts at 120 and should be converted to Celsius Do not forget to Introduce your Program to the user with descriptive information. Do not forget to include a block Comment header at the start of your Source Code.arrow_forwardCreate a Class Pet with the following data members Identification: String species : String (e.g. cat, dog, fish etc) breed: String Age (in days): int Weight: float Dead: boolean 1. Provide a constructor with parameters for all instance variables. 2. Provide getters for all and setters for only breed, and weight 3. Provide a method growOld() that increases the age of the pet by one day. A dead pet wont grow old 4. Provide a method growHealthy(float w) that increases the weight of the pet by the given amount w. A dead pet cannot grow healthy. 5. Provide a method fallSick(float w) that reduces the weight of the pet by the given amount. The least weight a pet can have is 0 which will mean that the pet has died. If the value of weigh is 10 kg and the method is called with an argument of 11 kg then you will set it to 0 and set the dead to an appropriate value to mark the death of the pet 6. Provide a toString method that shows an appropriate well formatted string…arrow_forwardwrite the definition of a class Player containing: an instance variable name of type string, initialized to the empty string an instance variable score of type int, initialized to 0 a method called set_name that has one parameter, whose value it assigns to the instance variable name a method cale set_score that has one parameter, whose value it assigns to teh instance variable score a method called get_name that has no parameters and that returns the value of the instance variable name a method called get_score that has no parameters and that returns the value of the instance variable score no constructor need to be defined using pythonarrow_forward
- In Java Write a Fraction class that implements these methods:• add ─ This method receives a Fraction parameter and adds the parameter fraction to thecalling object fraction.• multiply ─ This method receives a Fraction parameter and multiplies the parameterfraction by the calling object fraction.• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)• Separate accessor methods for each instance variable (numerator , denominator ) in theFraction classProvide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class shouldcontain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new…arrow_forwardwrite a class book, which has 3 attributes: title, price, quantity. Write the following methods, (8 points) a. setter/getter methods for each of the 3 attributes. b. __str__ method to display the complete information for the book, including title, price and quantity c. Method sell_copies(), passing the number of copies sold, which will reducethe value of quantity of the book. write the code in python please and thank youarrow_forwardPYTHON PROGRAMMING ONLY NEED HELParrow_forward
- This is for python class, so please answer in python 9.12 LAB: Product class In main.py define the Product class that will manage product inventory. Product class has three attributes: a product code, the product's price, and the number count of product in inventory. Implement the following methods: A constructor with 3 parameters that sets all 3 attributes to the value in the 3 parameters set_code(self, code) - set the product code (i.e. SKU234) to parameter code get_code(self) - return the product code set_price(self, price) - set the price to parameter price get_price(self) - return the price set_count(self, count) - set the number of items in inventory to parameter count get_count(self) - return the count add_inventory(self, amt) - increase inventory by parameter amt sell_inventory(self, amt) - decrease inventory by parameter amtarrow_forwardJAVA. THIS IS A PROGRAMMING ASSIGNMENT NOT A WRITING ASSIGNMENT IF YOU ANSWERED BEFORE DO NOT ANSWER AGAIN PLEASE DO NOT PASTE A PAPER Design a class to store the following information about computer scientists⦁ Name⦁ Research area(s)⦁ Major contribution A scientist may have more than one area of research.⦁ Implement the usual setters and getters methods ⦁ Implement a method that takes a research area as an input and return thenames of scientists associated with that area ⦁ Implement a method that takes a word as an input and return the names ofscientists whose contribution mention that word Next, perform desktop research to find at least five prominent black computerscientists, with their research area(s) and major contribution, and store this data intoobjects of the class you designed. You can just hardcode this data. No user input needed.Define JUnit tests to check your research area and contribution search methods.arrow_forwardIn Java Write a Fraction class that implements these methods:• add ─ This method receives a Fraction parameter and adds the parameter fraction to thecalling object fraction.• multiply ─ This method receives a Fraction parameter and multiplies the parameterfraction by the calling object fraction.• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)• Separate accessor methods for each instance variable (numerator , denominator ) in theFraction classProvide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class shouldcontain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
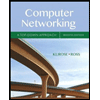
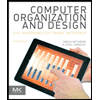
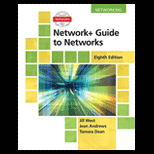
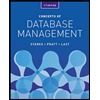
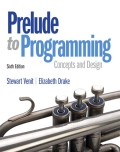
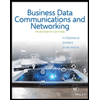