Introduction This particular program implements a game called "Checkers" that is played in real life. For this assignment, you should try to make your program look as nice as possible using the provided code fragment. Problem statement: Write a C++ program that plays the game of Checkers. The game is simple and you can view the instruction video online: https://www.youtube.com/watch?y=m0drB0cx8pQ You can also find a simulation if you want to see the game in action. One specific online simulation is at: http://www.csgnetwork.com/checkers.html Set Up: • Two players, one uses "x's, the other uses "o's. • Checkers may be played on an 8x8, 10x10 or 12x12 square board. • Command line arguments will be used to indicate the size of the board, for example: . /checkers 8 • No matter the size of the board, pieces are placed on the dark squares of the board, leaving the middle two rows blank. For example: How to Play (Game rules): • Players cannot move an opponent's piece. • Turns alternate between players until the game is won. • The player who runs out of pieces first or cannot make a valid move loses the game. • Pieces may only move diagonally into unoccupied squares and only by one square if they are not capturing a piece. • piece may capture opponent's pieces by jumping over them diagonally. So long as there are successive pieces to be captured, the piece may continue moving. The line the piece travels does not have to be straight along one diagonal, it may change directions so long as it continues to progress the board and capture pieces. When a piece has reached the King's Row (furthest row from it's originating side) then it is "kinged" by placing a second token on top of it (You may use "X's or "O's or any other distinguishable symbols to indicate "kinged" pieces). This piece is now permitted to move backwards on the board and allowed to capture backward.
Introduction This particular program implements a game called "Checkers" that is played in real life. For this assignment, you should try to make your program look as nice as possible using the provided code fragment. Problem statement: Write a C++ program that plays the game of Checkers. The game is simple and you can view the instruction video online: https://www.youtube.com/watch?y=m0drB0cx8pQ You can also find a simulation if you want to see the game in action. One specific online simulation is at: http://www.csgnetwork.com/checkers.html Set Up: • Two players, one uses "x's, the other uses "o's. • Checkers may be played on an 8x8, 10x10 or 12x12 square board. • Command line arguments will be used to indicate the size of the board, for example: . /checkers 8 • No matter the size of the board, pieces are placed on the dark squares of the board, leaving the middle two rows blank. For example: How to Play (Game rules): • Players cannot move an opponent's piece. • Turns alternate between players until the game is won. • The player who runs out of pieces first or cannot make a valid move loses the game. • Pieces may only move diagonally into unoccupied squares and only by one square if they are not capturing a piece. • piece may capture opponent's pieces by jumping over them diagonally. So long as there are successive pieces to be captured, the piece may continue moving. The line the piece travels does not have to be straight along one diagonal, it may change directions so long as it continues to progress the board and capture pieces. When a piece has reached the King's Row (furthest row from it's originating side) then it is "kinged" by placing a second token on top of it (You may use "X's or "O's or any other distinguishable symbols to indicate "kinged" pieces). This piece is now permitted to move backwards on the board and allowed to capture backward.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section4.3: Nested If Statements
Problem 7E
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
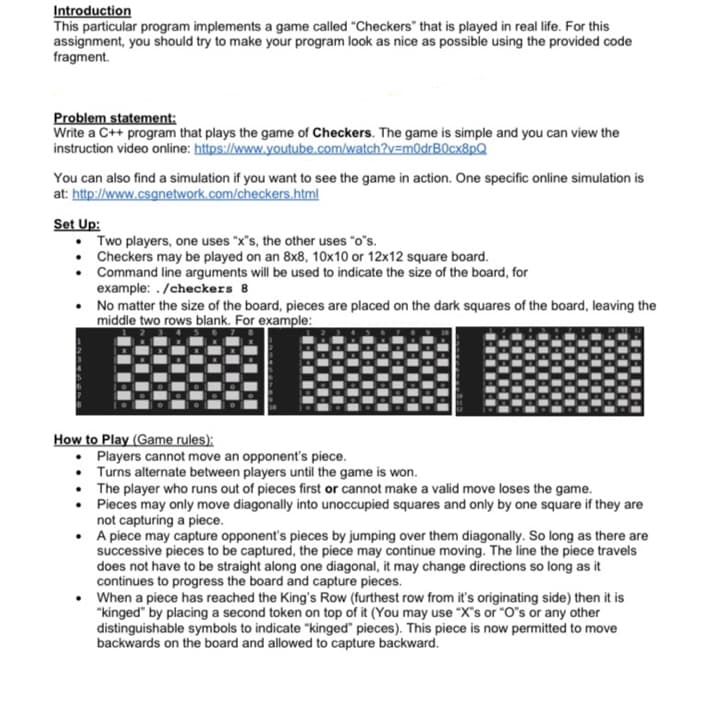
Transcribed Image Text:Introduction
This particular program implements a game called "Checkers" that is played in real life. For this
assignment, you should try to make your program look as nice as possible using the provided code
fragment.
Problem statement:
Write a C++ program that plays the game of Checkers. The game is simple and you can view the
instruction video online: https://www.youtube.com/watch?v=m0drB0cx8pQ
You can also find a simulation if you want to see the game in action. One specific online simulation is
at: http://www.csgnetwork.com/checkers.html
Set Up:
Two players, one uses "x"s, the other uses "o's.
• Checkers may be played on an 8x8, 10x10 or 12x12 square board.
• Command line arguments will be used to indicate the size of the board, for
example: ./checkers 8
• No matter the size of the board, pieces are placed on the dark squares of the board, leaving the
middle two rows blank. For example:
How to Play (Game rules):
• Players cannot move an opponent's piece.
• Turns alternate between players until the game is won.
• The player who runs out of pieces first or cannot make a valid move loses the game.
• Pieces may only move diagonally into unoccupied squares and only by one square if they are
not capturing a piece.
• A piece may capture opponent's pieces by jumping over them diagonally. So long as there are
successive pieces to be captured, the piece may continue moving. The line the piece travels
does not have to be straight along one diagonal, it may change directions so long as it
continues to progress the board and capture pieces.
When a piece has reached the King's Row (furthest row from it's originating side) then it is
"kinged" by placing a second token on top of it (You may use "X"s or "O's or any other
distinguishable symbols to indicate "kinged" pieces). This piece is now permitted to move
backwards on the board and allowed to capture backward.
![Implementation Requirements:
• Establish the size of the board via command line arguments, must include error handling for
too many and too few arguments as well as incorrect input. If an invalid size is provided, then
the program should display a message to indicate the problem, and recover by asking for the
size during runtime.
• The board must be created using two-dimensional, dynamic memory.
• The board must be correctly colored black and white using the following code as a base. The
following code fragment colors a two-dimensional board. It is expected that you will adjust it as
needed to provide the best user interface possible for your program.
for (int i=0; i<rows; i++)
{
for (int j=0; j<cols; j++)
{
if (i % 2 == 0 && j % 2 == 0)
cout « "I\033[30;47m " <« board[i] [j] « " ";
else if (i % 2 == 1 && j % 2 == 1)
cout « "I\033[30;47m " <« board[i][j] « " ";
else
cout « "I\033[0m
" « board[i]G] « " ";
cout « "\033[0m";
cout « endl;
The game must be correctly set up and played as described in the "Set Up" and "How to Play"
sections of this document.
The path of travel shall be received as a single, c-style string, and correctly parsed and error
handled to move the pieces in a correct fashion on the board. The string should be a list of
square coordinates. It is up to you as the programmer to determine the best delimiter and
coordinate system to use. Whatever method that is chosen should be clearly communicated to
the user.
• The board should be printed after each move of a piece and include the defined coordinate
system. This is especially important for a piece that may move multiple squares in a turn.
• After each move, captured pieces should be removed from the board.
• When the move sequence of a turn has been completed, the total number of captured pieces for
each player should be displayed.
• Ifa piece makes the King's Row then it should be "kinged" in some way. Kinged pieces are the
only pieces which may move forwards and backwards on the board.
• The game should end when only one color of piece remains on the board or if a player has run
out of valid moves.
If there is a winner, the program must immediately declare the winner and ask if the player(s)
want to play again (you may use the same board size or a different one by asking for user input).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8759b09c-a99c-4616-917a-1eb84cf5071d%2Fa4616e1b-0eb0-4271-8307-bf054da6b603%2Fpg4eid_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Implementation Requirements:
• Establish the size of the board via command line arguments, must include error handling for
too many and too few arguments as well as incorrect input. If an invalid size is provided, then
the program should display a message to indicate the problem, and recover by asking for the
size during runtime.
• The board must be created using two-dimensional, dynamic memory.
• The board must be correctly colored black and white using the following code as a base. The
following code fragment colors a two-dimensional board. It is expected that you will adjust it as
needed to provide the best user interface possible for your program.
for (int i=0; i<rows; i++)
{
for (int j=0; j<cols; j++)
{
if (i % 2 == 0 && j % 2 == 0)
cout « "I\033[30;47m " <« board[i] [j] « " ";
else if (i % 2 == 1 && j % 2 == 1)
cout « "I\033[30;47m " <« board[i][j] « " ";
else
cout « "I\033[0m
" « board[i]G] « " ";
cout « "\033[0m";
cout « endl;
The game must be correctly set up and played as described in the "Set Up" and "How to Play"
sections of this document.
The path of travel shall be received as a single, c-style string, and correctly parsed and error
handled to move the pieces in a correct fashion on the board. The string should be a list of
square coordinates. It is up to you as the programmer to determine the best delimiter and
coordinate system to use. Whatever method that is chosen should be clearly communicated to
the user.
• The board should be printed after each move of a piece and include the defined coordinate
system. This is especially important for a piece that may move multiple squares in a turn.
• After each move, captured pieces should be removed from the board.
• When the move sequence of a turn has been completed, the total number of captured pieces for
each player should be displayed.
• Ifa piece makes the King's Row then it should be "kinged" in some way. Kinged pieces are the
only pieces which may move forwards and backwards on the board.
• The game should end when only one color of piece remains on the board or if a player has run
out of valid moves.
If there is a winner, the program must immediately declare the winner and ask if the player(s)
want to play again (you may use the same board size or a different one by asking for user input).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
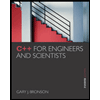
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
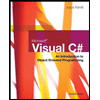
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
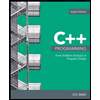
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
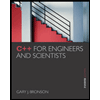
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
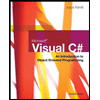
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
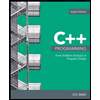
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning