inventory.py templete #========The beginning of the class ========== classShoe: def__init__(self,country,code,product,cost,quantity): pass ''' In this function, you must initialise the following attributes: ● country, ● code, ● product, ● cost, and ● quantity. ''' defget_cost(self): pass ''' Add the code to return the cost of the shoe in this method. '''defget_quantity(self):pass''' Add the code to return the quantity of the shoes. ''' def__str__(self): pass ''' Add a code to returns a string representation of a class. ''' #=============Shoe list==========='''The list will be used to store a list of objects of shoes.''' shoe_list=[] #==========Functions outside the class============== defread_shoes_data(): pass ''' This function will open the file inventory.txt and read the data from this file, then create a shoes object with this data and append this object into the shoes list. One line in this file represents data to create one object of shoes. You must use the try-except in this function for error handling. Remember to skip the first line using your code. ''' defcapture_shoes(): pass ''' This function will allow a user to capture data about a shoe and use this data to create a shoe object and append this object inside the shoe list. ''' defview_all(): pass ''' This function will iterate over the shoes list and print the details of the shoes returned from the __str__ function. Optional: you can organise your data in a table format by using Python’s tabulate module. ''' defre_stock(): pass ''' This function will find the shoe object with the lowest quantity, which is the shoes that need to be re-stocked. Ask the user if they want to add this quantity of shoes and then update it. This quantity should be updated on the file for this shoe. ''' defseach_shoe(): pass ''' This function will search for a shoe from the list using the shoe code and return this object so that it will be printed. ''' defvalue_per_item(): pass ''' This function will calculate the total value for each item. Please keep the formula for value in mind: value = cost * quantity. Print this information on the console for all the shoes. ''' defhighest_qty(): pass ''' Write code to determine the product with the highest quantity and print this shoe as being for sale. ''' #==========Main Menu============= '''Create a menu that executes each function above.This menu should be inside the while loop. Be creative!''' inventory.txt Country,Code,Product,Cost,Quantity South Africa,SKU44386,Air Max 90,2300,20 China,SKU90000,Jordan 1,3200,50 Vietnam,SKU63221,Blazer,1700,19 United States,SKU29077,Cortez,970,60 Russia,SKU89999,Air Force 1,2000,43 Australia,SKU57443,Waffle Racer,2700,4 Canada,SKU68677,Air Max 97,3600,13 Egypt,SKU19888,Dunk SB,1500,26 Britain,SKU76000,Kobe 4,3400,32 France,SKU84500,Pegasus,2490,28 Zimbabwe,SKU20207,Air Presto,2999,7 Morocco,SKU77744,Challenge Court,1450,11 Israel,SKU29888,Air Zoom Generation,2680,6 Uganda,SKU33000,Flyknit Racer,4900,9 Pakistan,SKU77999,Air Yeezy 2,4389,67 Brazil,SKU44600,Air Jordan 11,3870,24 Columbia,SKU87500,Air Huarache,2683,8 India,SKU38773,Air Max 1,1900,29 Vietnam,SKU95000,Air Mag,2000,2 Israel,SKU79084,Air Foamposite,2430,4 China,SKU93222,Air Stab,1630,10 South Korea,SKU66734,Hyperdunk,1899,7 Australia,SKU71827,Zoom Hyperfuse,1400,15 France,SKU20394,Eric Koston 1,2322,17
inventory.py templete #========The beginning of the class ========== classShoe: def__init__(self,country,code,product,cost,quantity): pass ''' In this function, you must initialise the following attributes: ● country, ● code, ● product, ● cost, and ● quantity. ''' defget_cost(self): pass ''' Add the code to return the cost of the shoe in this method. '''defget_quantity(self):pass''' Add the code to return the quantity of the shoes. ''' def__str__(self): pass ''' Add a code to returns a string representation of a class. ''' #=============Shoe list==========='''The list will be used to store a list of objects of shoes.''' shoe_list=[] #==========Functions outside the class============== defread_shoes_data(): pass ''' This function will open the file inventory.txt and read the data from this file, then create a shoes object with this data and append this object into the shoes list. One line in this file represents data to create one object of shoes. You must use the try-except in this function for error handling. Remember to skip the first line using your code. ''' defcapture_shoes(): pass ''' This function will allow a user to capture data about a shoe and use this data to create a shoe object and append this object inside the shoe list. ''' defview_all(): pass ''' This function will iterate over the shoes list and print the details of the shoes returned from the __str__ function. Optional: you can organise your data in a table format by using Python’s tabulate module. ''' defre_stock(): pass ''' This function will find the shoe object with the lowest quantity, which is the shoes that need to be re-stocked. Ask the user if they want to add this quantity of shoes and then update it. This quantity should be updated on the file for this shoe. ''' defseach_shoe(): pass ''' This function will search for a shoe from the list using the shoe code and return this object so that it will be printed. ''' defvalue_per_item(): pass ''' This function will calculate the total value for each item. Please keep the formula for value in mind: value = cost * quantity. Print this information on the console for all the shoes. ''' defhighest_qty(): pass ''' Write code to determine the product with the highest quantity and print this shoe as being for sale. ''' #==========Main Menu============= '''Create a menu that executes each function above.This menu should be inside the while loop. Be creative!''' inventory.txt Country,Code,Product,Cost,Quantity South Africa,SKU44386,Air Max 90,2300,20 China,SKU90000,Jordan 1,3200,50 Vietnam,SKU63221,Blazer,1700,19 United States,SKU29077,Cortez,970,60 Russia,SKU89999,Air Force 1,2000,43 Australia,SKU57443,Waffle Racer,2700,4 Canada,SKU68677,Air Max 97,3600,13 Egypt,SKU19888,Dunk SB,1500,26 Britain,SKU76000,Kobe 4,3400,32 France,SKU84500,Pegasus,2490,28 Zimbabwe,SKU20207,Air Presto,2999,7 Morocco,SKU77744,Challenge Court,1450,11 Israel,SKU29888,Air Zoom Generation,2680,6 Uganda,SKU33000,Flyknit Racer,4900,9 Pakistan,SKU77999,Air Yeezy 2,4389,67 Brazil,SKU44600,Air Jordan 11,3870,24 Columbia,SKU87500,Air Huarache,2683,8 India,SKU38773,Air Max 1,1900,29 Vietnam,SKU95000,Air Mag,2000,2 Israel,SKU79084,Air Foamposite,2430,4 China,SKU93222,Air Stab,1630,10 South Korea,SKU66734,Hyperdunk,1899,7 Australia,SKU71827,Zoom Hyperfuse,1400,15 France,SKU20394,Eric Koston 1,2322,17
Chapter14: Files And Streams
Section: Chapter Questions
Problem 1CP
Related questions
Question
inventory.py templete
#========The beginning of the class ==========
classShoe:
def__init__(self,country,code,product,cost,quantity):
pass
''' In this function, you must initialise the following attributes:
● country,
● code,
● product,
● cost,
and ● quantity.
'''
defget_cost(self):
pass
''' Add the code to return the cost of the shoe in this method. '''defget_quantity(self):pass''' Add the code to return the quantity of the shoes. '''
def__str__(self):
pass
''' Add a code to returns a string representation of a class. '''
#=============Shoe list==========='''The list will be used to store a list of objects of shoes.'''
shoe_list=[]
#==========Functions outside the class==============
defread_shoes_data():
pass
''' This function will open the file inventory.txt and read the data from this file, then create a shoes object with this data and append this object into the shoes list. One line in this file represents data to create one object of shoes. You must use the try-except in this function for error handling. Remember to skip the first line using your code. '''
defcapture_shoes():
pass
''' This function will allow a user to capture data about a shoe and use this data to create a shoe object and append this object inside the shoe list. '''
defview_all():
pass
''' This function will iterate over the shoes list and print the details of the shoes returned from the __str__ function. Optional: you can organise your data in a table format by using Python’s tabulate module. '''
defre_stock():
pass
''' This function will find the shoe object with the lowest quantity, which is the shoes that need to be re-stocked. Ask the user if they want to add this quantity of shoes and then update it. This quantity should be updated on the file for this shoe. '''
defseach_shoe():
pass
''' This function will search for a shoe from the list using the shoe code and return this object so that it will be printed. '''
defvalue_per_item():
pass
''' This function will calculate the total value for each item. Please keep the formula for value in mind: value = cost * quantity. Print this information on the console for all the shoes. '''
defhighest_qty():
pass
''' Write code to determine the product with the highest quantity and print this shoe as being for sale. '''
#==========Main Menu=============
'''Create a menu that executes each function above.This menu should be inside the while loop. Be creative!'''
inventory.txt
Country,Code,Product,Cost,Quantity South Africa,SKU44386,Air Max 90,2300,20 China,SKU90000,Jordan 1,3200,50 Vietnam,SKU63221,Blazer,1700,19 United States,SKU29077,Cortez,970,60 Russia,SKU89999,Air Force 1,2000,43 Australia,SKU57443,Waffle Racer,2700,4 Canada,SKU68677,Air Max 97,3600,13 Egypt,SKU19888,Dunk SB,1500,26 Britain,SKU76000,Kobe 4,3400,32 France,SKU84500,Pegasus,2490,28 Zimbabwe,SKU20207,Air Presto,2999,7 Morocco,SKU77744,Challenge Court,1450,11 Israel,SKU29888,Air Zoom Generation,2680,6 Uganda,SKU33000,Flyknit Racer,4900,9 Pakistan,SKU77999,Air Yeezy 2,4389,67 Brazil,SKU44600,Air Jordan 11,3870,24 Columbia,SKU87500,Air Huarache,2683,8 India,SKU38773,Air Max 1,1900,29 Vietnam,SKU95000,Air Mag,2000,2 Israel,SKU79084,Air Foamposite,2430,4 China,SKU93222,Air Stab,1630,10 South Korea,SKU66734,Hyperdunk,1899,7 Australia,SKU71827,Zoom Hyperfuse,1400,15 France,SKU20394,Eric Koston 1,2322,17
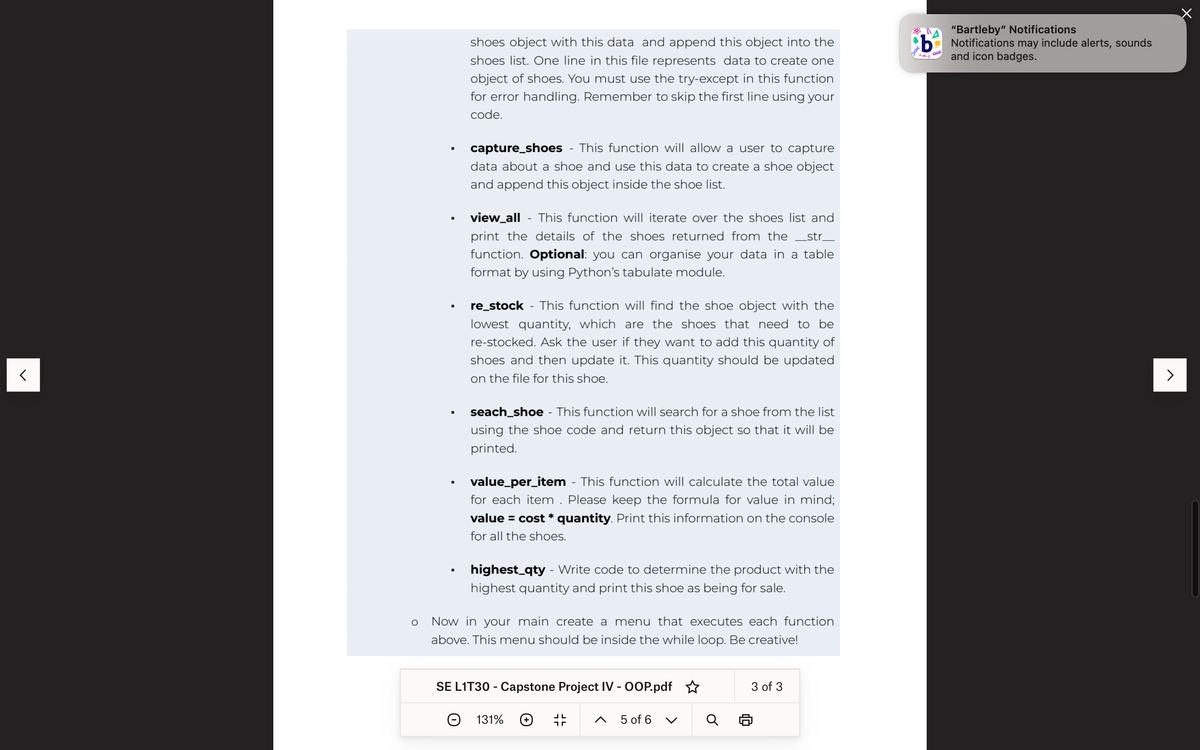
Transcribed Image Text:<
▪
.
▪
shoes object with this data and append this object into the
shoes list. One line in this file represents data to create one
object of shoes. You must use the try-except in this function
for error handling. Remember to skip the first line using your
code.
capture_shoes - This function will allow a user to capture
data about a shoe and use this data to create a shoe object
and append this object inside the shoe list.
view_all - This function will iterate over the shoes list and
print the details of the shoes returned from the _str_
function. Optional: you can organise your data in a table
format by using Python's tabulate module.
re_stock - This function will find the shoe object with the
lowest quantity, which are the shoes that need to be
re-stocked. Ask the user if they want to add this quantity of
shoes and then update it. This quantity should be updated
on the file for this shoe.
seach_shoe - This function will search for a shoe from the list
using the shoe code and return this object so that it will be
printed.
value_per_item - This function will calculate the total value
for each item. Please keep the formula for value in mind;
value = cost * quantity. Print this information on the console
for all the shoes.
highest_qty - Write code to determine the product with the
highest quantity and print this shoe as being for sale.
O Now in your main create a menu that executes each function
above. This menu should be inside the while loop. Be creative!
SE L1T30 - Capstone Project IV - OOP.pdf
131%
5 of 6
3 of 3
"Bartleby" Notifications
b Notifications may include alerts, sounds
()
and icon badges.
>
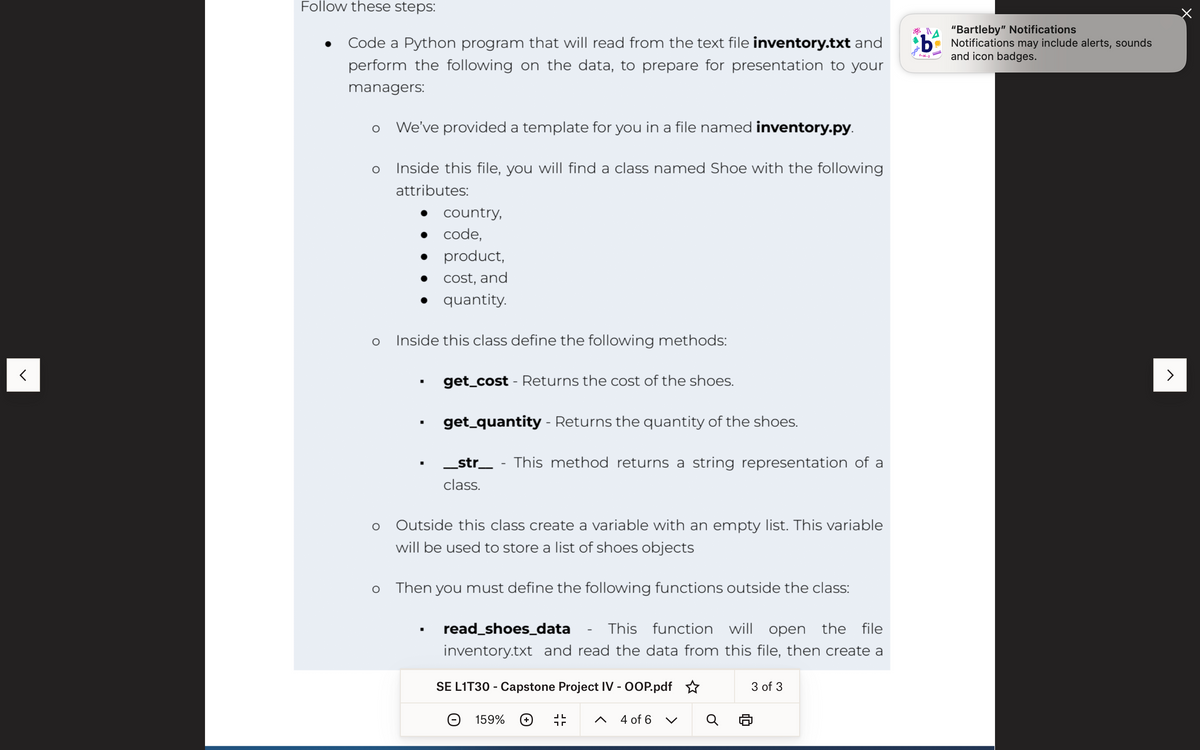
Transcribed Image Text:<
Follow these steps:
Code a Python program that will read from the text file inventory.txt and
perform the following on the data, to prepare for presentation to your
managers:
O
O
O
O
We've provided a template for you in a file named inventory.py.
Inside this file, you will find a class named Shoe with the following
attributes:
●
●
Inside this class define the following methods:
■
■
■
country,
code,
product,
cost, and
quantity.
■
get_cost - Returns the cost of the shoes.
get_quantity - Returns the quantity of the shoes.
_str_ - This method returns a string representation of a
class.
Outside this class create a variable with an empty list. This variable
will be used to store a list of shoes objects
Then you must define the following functions outside the class:
read_shoes_data - This function will open the file
inventory.txt and read the data from this file, then create a
SE L1T30 - Capstone Project IV - OOP.pdf
159%
חר
4 of 6
3 of 3
b
"Bartleby" Notifications
Notifications may include alerts, sounds
and icon badges.
>
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
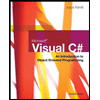
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
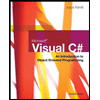
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,