Java Program using Replit, please help. I circled the problem with red, because whenever I run the program, the entire output doesn't show and it tells me that "./Main.java uses unchecked or unsafe operations". How can I stop that from happening? Program in the photos.
Java Program using Replit, please help. I circled the problem with red, because whenever I run the program, the entire output doesn't show and it tells me that "./Main.java uses unchecked or unsafe operations". How can I stop that from happening? Program in the photos.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Java Program using Replit, please help. I circled the problem with red, because whenever I run the program, the entire output doesn't show and it tells me that "./Main.java uses unchecked or unsafe operations". How can I stop that from happening?
Program in the photos.
![57 for (int u = 0; u < V; u++)
58
59 //Doesn't recur if already visited
if (!visited[u])
60
61 if (isCyclicUtil(u,
visited, -1))
62 return true;
63
return false;
64 }
65
66 //Driver Method
67▼ public static void main(String[] args) {
68 // Creates a graph
69
Main g1= new Main (5);
70
g1.addEdge (1, 0);
71
g1.addEdge(0, 2);
72 g1.addEdge (2, 0);
73 g1.addEdge(0, 3);
74 g1.addEdge (3, 4);
if (g1.isCyclic())
System.out.println("Graph
75
contains a cycle");
78
System.out.println("Graph doesn't contain a cycle");
79 Main g2 = new Main(3);
76
77
else
80 g2.addEdge(0, 1);
81
g2.addEdge (1, 2);
82 if (g2.isCyclic())
83 System.out.println("Graph
84
System.out.println("Graph
else
85
86
87 }
88 }
contains a cycle");
doesn't contain a cycle");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4cd5838b-3f34-487e-9642-7770197fe05c%2F79634c60-80ea-4b68-a935-181b01cf9d6b%2Ffz1z65k_processed.png&w=3840&q=75)
Transcribed Image Text:57 for (int u = 0; u < V; u++)
58
59 //Doesn't recur if already visited
if (!visited[u])
60
61 if (isCyclicUtil(u,
visited, -1))
62 return true;
63
return false;
64 }
65
66 //Driver Method
67▼ public static void main(String[] args) {
68 // Creates a graph
69
Main g1= new Main (5);
70
g1.addEdge (1, 0);
71
g1.addEdge(0, 2);
72 g1.addEdge (2, 0);
73 g1.addEdge(0, 3);
74 g1.addEdge (3, 4);
if (g1.isCyclic())
System.out.println("Graph
75
contains a cycle");
78
System.out.println("Graph doesn't contain a cycle");
79 Main g2 = new Main(3);
76
77
else
80 g2.addEdge(0, 1);
81
g2.addEdge (1, 2);
82 if (g2.isCyclic())
83 System.out.println("Graph
84
System.out.println("Graph
else
85
86
87 }
88 }
contains a cycle");
doesn't contain a cycle");
![2 import java.util.LinkedList;
3 import java.util.*;
4 class Main {
5 //Number of Vertices
6 private int V;
79
8 //Adjacency Lists
9 private LinkedList<Integer> adj[];
10
11 //Constructor
12 Main(int v) {
13
V = V
14
adj = new LinkedList[v];
15 for (int i = 0; i < V; ++i)
16 adj[i] = new LinkedList();
17 }
18
19 // Function that adds an edge to the graph
20 void addEdge(int v, int w) {
21 adj[v].add(w);
22 adj[w].add(v);
23 }
24
25 // A recursive function that uses visited [] and parent to detect
cycle in subgraph reachable from vertex v.
26 ▼ Boolean isCyclicUtil(int v, Boolean visited [], int parent) {
27
28 //Marks the current node as visited
29
visited [v] = true;
30
Integer i;
31
32 // Recur for all the vertices adjacent to this vertex
Iterator<Integer> it = adj[v]. iterator();
33
34 while (it.hasNext()) {
35
i= it.next();
36
37 // If an adjacent is not visited, then recur for that adjacent
38
if (!visited[i]) {
39 if (isCyclicUtil(i, visited, v))
40 return true;
41 }
42
// If an adjacent is visited and not parent of current vertex, then
there is a cycle
else if (i != parent)
return true;
43
44
45 }
46 return false;
47 }
48 // Returns true if the graph contains a cycle, else false.
49 Boolean isCyclic() {
50
51 // Mark all the vertices as not visited and not part of recursion
stack
52 Boolean visited [] = new Boolean [V];
53 for (int i = 0; i < V; i++)
54 visited[i] = false;
55
56 // Call the recursive helper function to detect cycle in different
DFS trees](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4cd5838b-3f34-487e-9642-7770197fe05c%2F79634c60-80ea-4b68-a935-181b01cf9d6b%2Flqtpvw_processed.png&w=3840&q=75)
Transcribed Image Text:2 import java.util.LinkedList;
3 import java.util.*;
4 class Main {
5 //Number of Vertices
6 private int V;
79
8 //Adjacency Lists
9 private LinkedList<Integer> adj[];
10
11 //Constructor
12 Main(int v) {
13
V = V
14
adj = new LinkedList[v];
15 for (int i = 0; i < V; ++i)
16 adj[i] = new LinkedList();
17 }
18
19 // Function that adds an edge to the graph
20 void addEdge(int v, int w) {
21 adj[v].add(w);
22 adj[w].add(v);
23 }
24
25 // A recursive function that uses visited [] and parent to detect
cycle in subgraph reachable from vertex v.
26 ▼ Boolean isCyclicUtil(int v, Boolean visited [], int parent) {
27
28 //Marks the current node as visited
29
visited [v] = true;
30
Integer i;
31
32 // Recur for all the vertices adjacent to this vertex
Iterator<Integer> it = adj[v]. iterator();
33
34 while (it.hasNext()) {
35
i= it.next();
36
37 // If an adjacent is not visited, then recur for that adjacent
38
if (!visited[i]) {
39 if (isCyclicUtil(i, visited, v))
40 return true;
41 }
42
// If an adjacent is visited and not parent of current vertex, then
there is a cycle
else if (i != parent)
return true;
43
44
45 }
46 return false;
47 }
48 // Returns true if the graph contains a cycle, else false.
49 Boolean isCyclic() {
50
51 // Mark all the vertices as not visited and not part of recursion
stack
52 Boolean visited [] = new Boolean [V];
53 for (int i = 0; i < V; i++)
54 visited[i] = false;
55
56 // Call the recursive helper function to detect cycle in different
DFS trees
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
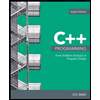
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
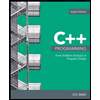
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning