Java Question - Using the bottom code, create another class named "DigitalBook" as a subclass of the given "Book" class. Then, Make sure that the "DigitalBook" class contains two constructors: (1) one is the default constructor that takes no parameter, and (2) the other is a parameterized constructor that take 6 parameters. Be sure to use the “super” keyword in the constructors of the "DigitalBook" class. In the “main()” method of the “EX12_1” class, create four instances: “b1”, “b2”, d1”, and “d2”. Let “b1” and “b2” be the instances of the “Book” class, and let the “d1” and “d2” be the instance of the “DigitalBook” class. Be sure to use the specified constructor (as shown in attached picture) to initialize these instances. The Final Output is shown in the attached pictures. Please make sure that no errors show up in the Java Compiler when testing the code. Thank you. Add the following code to the "class Book" code (Below the ___ line). public class EX12_1 { public static void main(String[] args) { /////add your code here } } ________________________________________________________________________________ class Book { public int totalPage, totalChapter; public double unitPrice; public String title, author, ISBN; public String format = "Paper"; public Book() { } public Book(String tl, String at, String isbn, int tp, int tc, double up) { title = tl; author = at; ISBN = isbn; totalPage = tp; totalChapter = tc; unitPrice = up; } void showBook() { System.out.println("Title: " + title); System.out.println("Author: " + author); System.out.println("ISBN: " + ISBN); System.out.println("Total Pages: " + totalPage); System.out.println("Total Chapters: " + totalChapter); System.out.println("Unit Price: " + unitPrice); System.out.println("Format: " + format + "\n"); } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Java Question - Using the bottom code, create another class named "DigitalBook" as a subclass of the given "Book" class. Then, Make sure that the "DigitalBook" class contains two constructors:
(1) one is the default constructor that takes no parameter, and (2) the other is a parameterized constructor that take 6 parameters. Be sure to use the “super” keyword in the constructors of the "DigitalBook" class. In the “main()” method of the “EX12_1” class, create four instances: “b1”, “b2”, d1”, and “d2”. Let “b1” and “b2” be the instances of the “Book” class, and let the “d1” and “d2” be the instance of the “DigitalBook” class. Be sure to use the specified constructor (as shown in attached picture) to initialize these instances. The Final Output is shown in the attached pictures. Please make sure that no errors show up in the Java Compiler when testing the code. Thank you.
Add the following code to the "class Book" code (Below the ___ line).
public class EX12_1
{
public static void main(String[] args)
{
/////add your code here
}
}
________________________________________________________________________________
class Book
{
public int totalPage, totalChapter;
public double unitPrice;
public String title, author, ISBN;
public String format = "Paper";
public Book() { }
public Book(String tl, String at, String isbn, int tp, int tc, double up)
{
title = tl;
author = at;
ISBN = isbn;
totalPage = tp;
totalChapter = tc;
unitPrice = up;
}
void showBook()
{
System.out.println("Title: " + title);
System.out.println("Author: " + author);
System.out.println("ISBN: " + ISBN);
System.out.println("Total Pages: " + totalPage);
System.out.println("Total Chapters: " + totalChapter);
System.out.println("Unit Price: " + unitPrice);
System.out.println("Format: " + format + "\n");
}
}
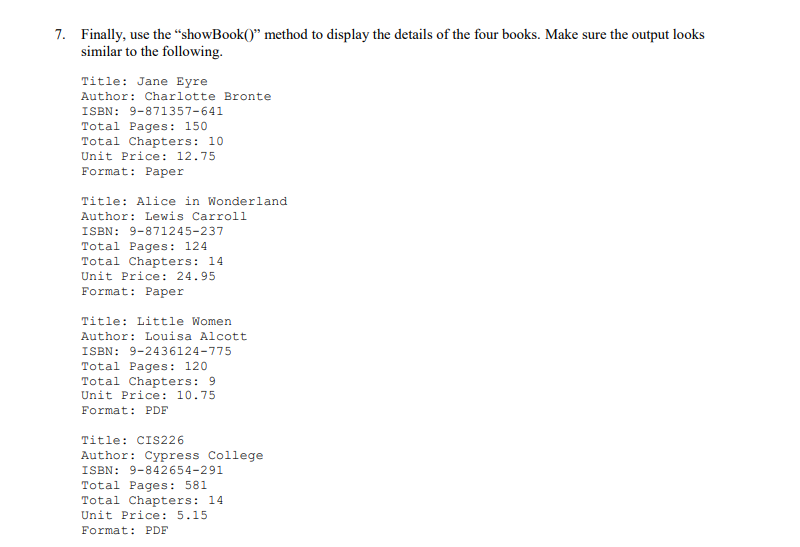
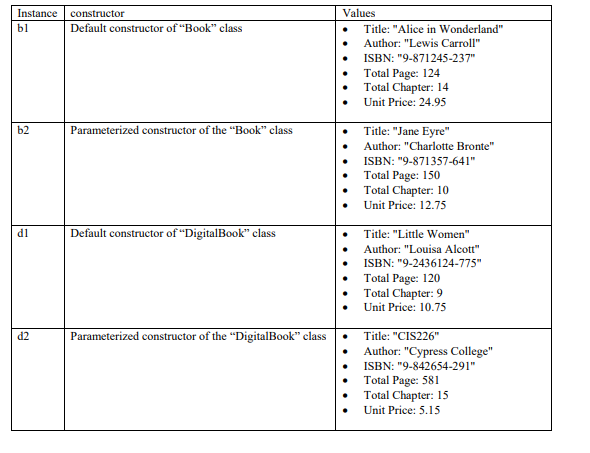

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

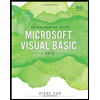
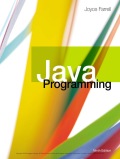
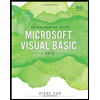
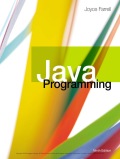
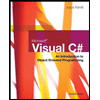