l; while(i
For this problem I have a java code:
import java.util.Arrays; import java.util.Scanner; public class MergeSortWeightedSumInversion { public static long merge(int arr[], int l, int mid, int r) { long weight = 0; int n1 = mid-l+1; int n2 = r-mid; int[] a = new int[n1]; int[] b = new int[n2]; for(int i = 0; i < n1; i++) { a[i] = arr[l+i]; } for(int i = 0; i < n2; i++) { b[i] = arr[mid+i+1]; } int i = 0, j = 0, k = l; while(i<n1 && j<n2) { if(a[i] <= b[j]) { arr[k] = a[i]; k++; i++; } else { weight += (a[i] + b[j]); arr[k] = b[j]; k++; j++; } } while(i<n1) { arr[k] = a[i]; k++; i++; } while(j<n2) { arr[k] = b[j]; k++; j++; } return weight; } public static long mergeSort(int arr[], int l, int r) { long weight = 0; if(l < r) { int mid = (l+r)/2; weight += mergeSort(arr, l, mid); weight += mergeSort(arr,mid+1,r); weight += merge(arr,l,mid,r); } return weight; } public static void main(String[] args) { Scanner scan = new Scanner(System.in); int n; n = scan.nextInt(); int[] arr = new int[n]; for(int i = 0; i < n; i++) { arr[i] = scan.nextInt(); } System.out.println(mergeSort(arr, 0, n-1)); } } This is wrong because I tried testing it on the array: [7,3,8,1,5] and I got a weight of 26, its only adding up half of the inversions. How would I modify it so that it works? Code in java and time complexity must be O(n log(n)) please.
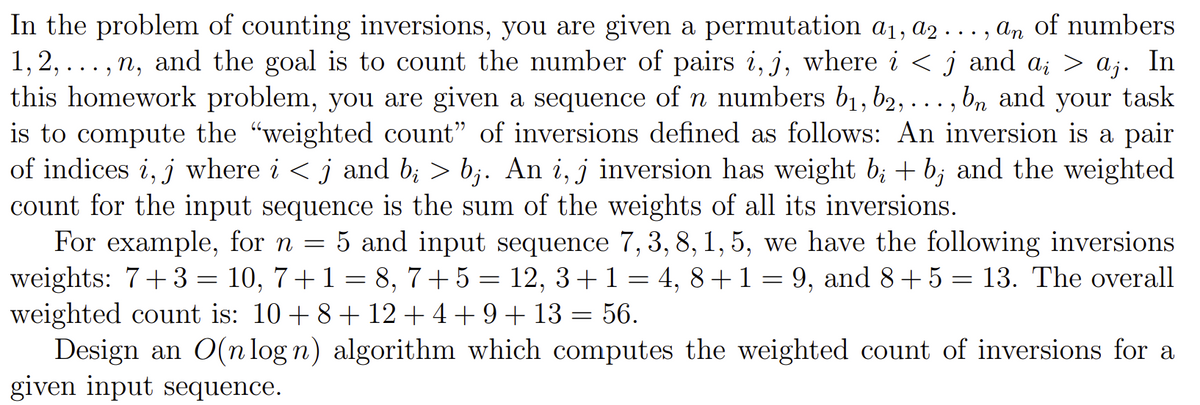

Step by step
Solved in 3 steps with 1 images

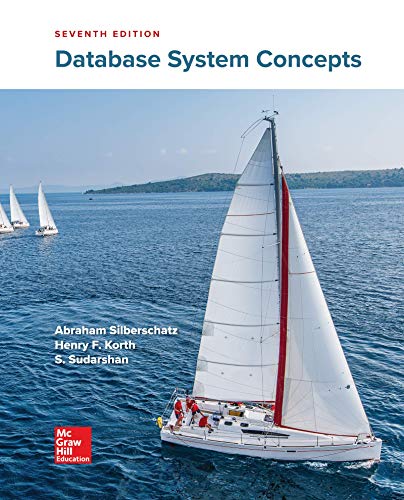
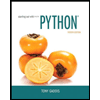
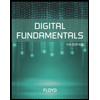
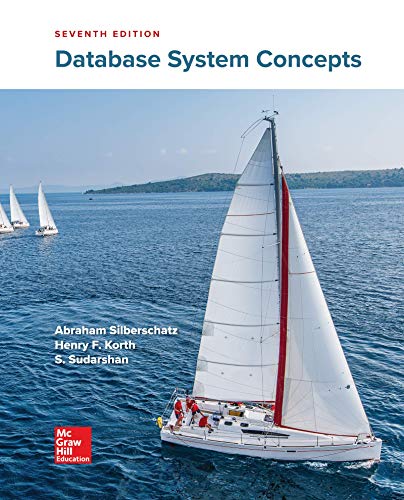
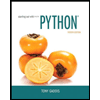
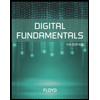
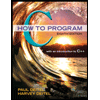
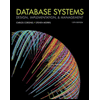
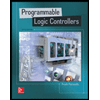