What is meant by function ?
A set of statements, which performs a particular task is called a function. The program gains modularity by using function. The application is quite easy to understand, read, and edit when they are created with functions. The developer/programmer creates a function and uses main() to call the function. Once the function gets executed, the control is transferred to the main program.
Function declaration
Declaring a function is to inform the compiler about the existence of a specific function. Memory space will not be allocated during declaration. The function declaration has three parts namely data type of return value, name of the function and comma-separated list of parameters and their types.
Syntax
return_type function_name (parameter_list);
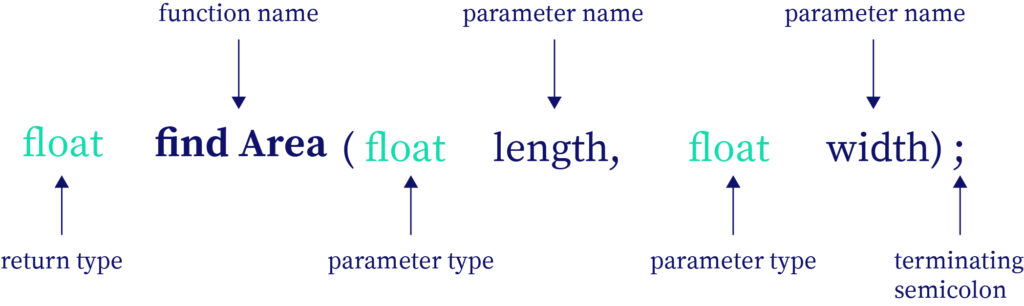
Return type
Return type represents the data type of a value returned by the function. It could be floating-point, integer, character, etc. When the return type is void, it indicates that the function does not return any value.
Name
The function is called using the function name. Function names must obey the identifier rules and it signifies the task carried out by the function.
Parameter list
The parameter list stores the value of arguments passed during function invocation. It is passed through the function call. Some functions do not accept any value in which case the parameter list can be omitted.
Example
int sum_of_num (int n1, int n2); //function declaration
Explanation
From the above code, function sum_of_num() is declared with int data type and two parameters with the int data type. The function sum_of_num() is only declared and not defined.
Function definition
The function definition is also known as function implementation. It represents the actual code the function needs to perform. A function definition has function header and function body. The header is similar to the function declaration and body contains the lines of code enclosed between curly braces that performs the intended task.
Syntax
return_type function_name (parameter_list)
{
//functionbody
}
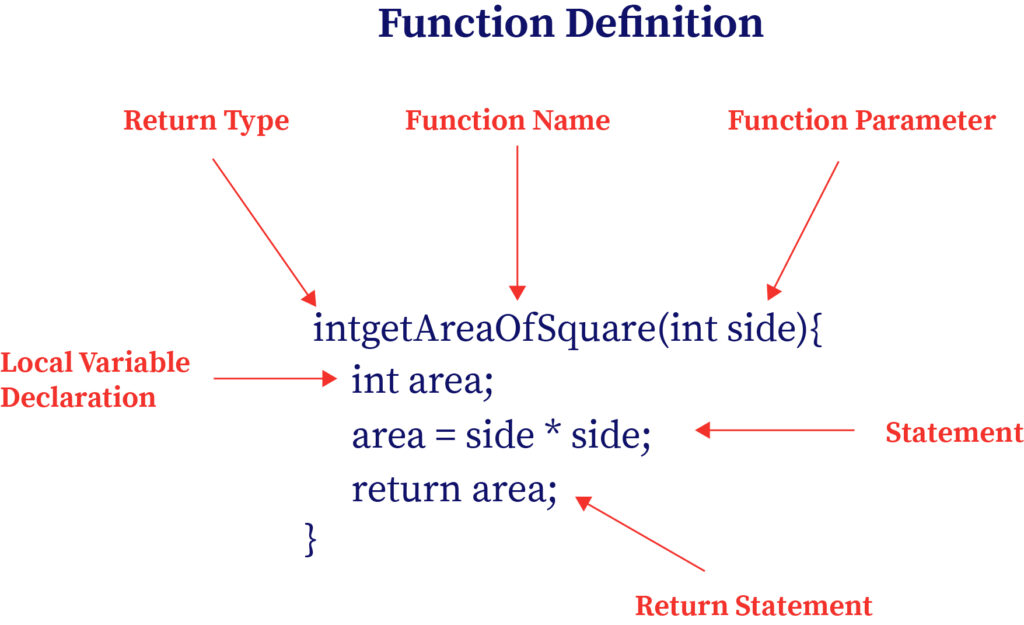
Return type
The data type of a value returned by the function is referred to as the return type of a function. The data type can be integer, floating-point, character, class objects, or even pointer. When the function does not return any value, then the void type is used.
Function name
The function name is chosen such that it represents the operation performed by the function and it should obey the identifier rules.
Parameter list
Parameter lists are the variables, which hold the argument’s value that is passed during the function call. Having a parameter list in a function is optional.
Function body
The main part of a function is the function body, which defines the statement/code that the function needs to perform.
Example
void sum_of_num (int nu1, int nu2) //function definition
{
int nu3; //initialization
nu3= nu1+nu2; //add numbers
cout<< “n The sum of nu1 and nu2 is : ”; //display statement
cout << nu3 << ” n ”; //returning the sum
}
Explanation
From the above code, function sum_of_num() is defined. The memory of the function is allocated when the function is defined.
Function call
Functions are called using function names. When a function is called, then the program control is passed to a particular function to execute the defined task. When there is no argument in the function, then it is called directly using its name. If the function contains an argument, then it is called using call by value or call by reference.
Example for declare, define, and call
C++ program to find the sum using function.
Code
#include <iostream>
using namespace std;
void sum_of_num (int nu1, int nu2); //function declaration
int main()
{
int n1 =22; //variable initialization
int n2 =33;
sum_of_num (n1, n2); //function call
}
void sum_of_num (int nu1, int nu2) //function definition
{
int num3;
num3= nu1+nu2; //add numbers
cout<< “n The sum of nu1 and nu2 is: ”;
cout << num3 << ” n ”; //print sum
}
Output
The sum of nu1 and nu2 is: 55
Code explanation
The above code has been declared with a function sum_of_num(); where n1 and n2 are the integer variables and argument of the sum_of_num () function call. The sum_of_num () function is defined with nu1 and nu2 as its parameter, whereas nu1, nu2 stores the value of n1, n2. The addition operation is executed within the function and displays the result of addition using num3 variable.
Difference between function declaration and function definition
The differences are based on definition, functionality, and consist of (what function contains).
Definition
The major difference between definition and declaration is that the function declaration is a kind of prototype that specifies the name of function along with parameters and return type; but does not contain the function body. Whereas, function definition contains function header which is similar to the declaration and the function body.
Functionality
The functional difference is, the function declaration notifies the compiler about the function and how it is to be called whereas, definition represents the actual functionality which is performed.
Consist of
The declaration consists of name, parameter, and return type whereas, the definition consists of name, parameter, return type, and function body (statements).
Function argument
Comma-separated list of variables in the function declaration or header is known as the function’s formal parameters. The behavior of the formal parameter is the same as the local variable inside the function; it is created at the function entry and destroyed at the function exit. During function call in C, the arguments are passed using two different methods.
Call by value
The argument’s actual value is copied to a formal parameter. The changes made to those variables inside the function is not reflected in the calling function.
Call by reference
The argument’s address is copied to the formal parameter in this method. The changes made to those variables inside the function get reflected in the calling function since both refer to the same location.
Context and Applications
This topic is important for Postgraduate and Undergraduate courses, particularly for, Bachelors in Computer Science Engineering and Associate of Science in Computer Science.
Practice Problems
Question 1: Find the incorrect function declaration with the default argument.
a) int func1(int A, int B = 3; int C= 11)
b) int func1(int A = 2, int B = 3; int C)
c) int func1(int A = 2, int B; int C= 11)
d) all are correct
Answer: Option a is correct.
Explanation: In function, the default argument declaration is initialized from right to left. In general, the default argument is used during the program compilation.
Question 2: Find the correct statement.
a) There can be only one default parameter
b) The function can have n number of default parameters
c) A function can have a maximum of two default parameters out of its three parameters
d) None of the above
Answer: Option b is correct.
Explanation: All the parameters in a function can be default parameters and the function can have more than one as its default parameter.
Question 3: Find the incorrect function declaration.
a) int 8fun(int*, float[])
b) int 2fun(float x)
c) int 9fun(double)
d) all the above
Answer: Option d is correct.
Explanation: Function name should not have any numerical values, special characters as its first character. The name can start with an underscore or alphabet.
Question 4: Pick the correct answer
a) Both function declaration and definition are same.
b) Function definition contains function header but not body. Function declaration has both.
c) Function definition has both header and body whereas declaration contains only the header.
d) Both function declaration and definition contains only header.
Answer: Option c is correct.
Explanation: Function declaration contains the prototype (header) whereas definition consists of header as well as body.
Question 5: How many values a function might return?
a) 0
b) 4
c) 1
d) 2
Answer: Option c is correct.
Explanation: A function can return up to one value. If the user tries to return two or more values, it returns only one value and it appears in the rightmost place of a return statement.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Declaring and Defining the function Homework Questions from Fellow Students
Browse our recently answered Declaring and Defining the function homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.