Lab 5- Money class object – 2021 SP CS-116 Define class object Money with the following characteristics: Private attribute: int dollars; • int cents Define any Methods/functions/operators you need for this program. The class object should also have the following overloaded operators: Subtraction operator. If one Money object is subtracted from another, the operator should give the difference between the two Money. For example, if $5.80 is subtracted from $8.75, the result will be $2.95. Addition operator. If one Money object is added from another, the operator should give the sum between the two Money. For example, if $5.80 is subtracted from $8.75, the result will be $14.55. Multiplication operator. If one Money object is multiplying by x the operator should return x* Money object. For example, $8.75 *2 will return $17.50. Equality operator. If one Money object is compared from another (x == y), the operator should return true if x is same as y. For example, $10.01 == 10.01 will return true.
Lab 5- Money class object – 2021 SP CS-116 Define class object Money with the following characteristics: Private attribute: int dollars; • int cents Define any Methods/functions/operators you need for this program. The class object should also have the following overloaded operators: Subtraction operator. If one Money object is subtracted from another, the operator should give the difference between the two Money. For example, if $5.80 is subtracted from $8.75, the result will be $2.95. Addition operator. If one Money object is added from another, the operator should give the sum between the two Money. For example, if $5.80 is subtracted from $8.75, the result will be $14.55. Multiplication operator. If one Money object is multiplying by x the operator should return x* Money object. For example, $8.75 *2 will return $17.50. Equality operator. If one Money object is compared from another (x == y), the operator should return true if x is same as y. For example, $10.01 == 10.01 will return true.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need help with lab 5 money
I can't upload more than 2 images how do I do that?
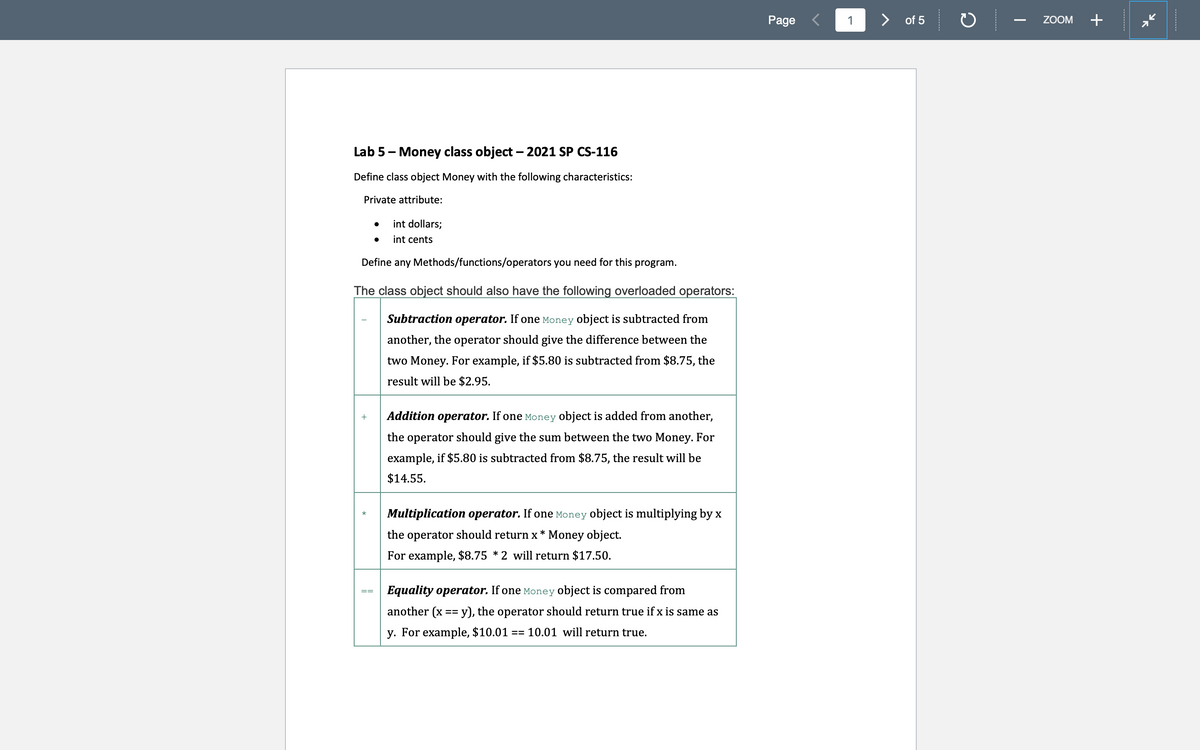
Transcribed Image Text:Page <
> of 5
ZOOM
+
Lab 5- Money class object – 2021 SP CS-116
Define class object Money with the following characteristics:
Private attribute:
int dollars;
int cents
Define any Methods/functions/operators you need for this program.
The class object should also have the following overloaded operators:
Subtraction operator. If one Money object is subtracted from
another, the operator should give the difference between the
two Money. For example, if $5.80 is subtracted from $8.75, the
result will be $2.95.
Addition operator. If one Money object is added from another,
the operator should give the sum between the two Money. For
example, if $5.80 is subtracted from $8.75, the result will be
$14.55.
Multiplication operator. If one Money object is multiplying by x
the operator should return x * Money object.
For example, $8.75 *2 will return $17.50.
Equality operator. If one Money object is compared from
==
another (x == y), the operator should return true if x is same as
y. For example, $10.01
== 10.01 will return true.
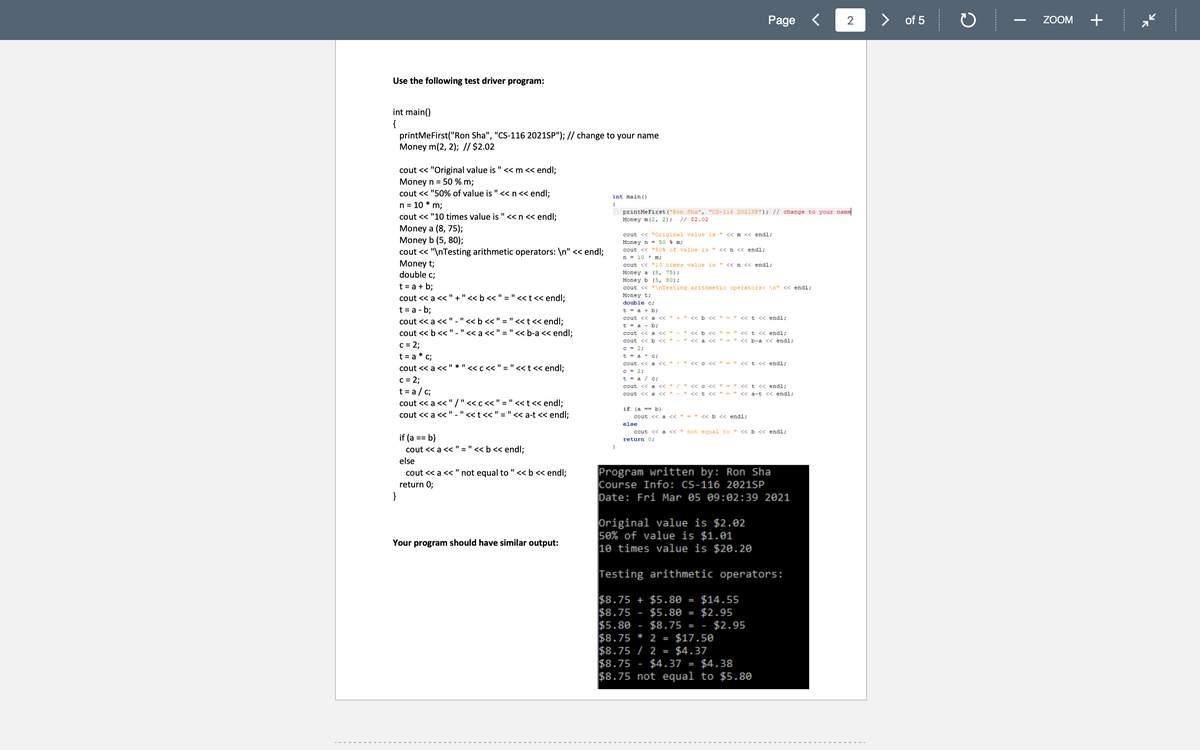
Transcribed Image Text:Page
く
of 5
ZOOM
+
Use the following test driver program:
int main()
{
printMeFirst("Ron Sha", "CS-116 2021SP"); // change to your name
Money m(2, 2); // $2.02
cout << "Original value is " «m << endl;
Money n = 50 % m;
cout << "50% of value is "<< n<< endl;
n = 10 * m;
cout << "10 times value is "<<n << endl;
int main ()
{
printMeFirst ("Ron Sha", "CS-116 2021SP"); // change to your name
Money m(2, 2);
// $2.02
Money a (8, 75);
Money b (5, 80);
cout << "\nTesting arithmetic operators: \n" « endl;
Money t;
double c;
cout << "Original value is " << m << endl;
Money n - 50 m;
cout << "50% of value is " << n << endl;
n = 10 * m;
cout << "10 times value is " <<n << endl;
Money a (8, 75);
Money b (5, 80);
t = a + b;
cout << a <<"+"<< b << " ="<< t<< endl;
t = a - b;
cout << a << "-"<< b << " ="<<t<< endl;
cout << b << "."< a << " ="<< b-a << endl;
c = 2;
t = a * c;
cout << "\nTesting arithmetic operators: \n" << endl;
Money t;
double c;
t - a + b;
cout << a << " +" « b << " - " << t << endl;
t - a - b;
" << b << " = " << t << endl;
- " << b-a << endl;
cout << a << "
cout <く b << " - " <く a <
C - 2;
t - a * c;
cout << a < "*" < c
c - 2;
t - a / c;
cout << a < "/" < c << " = " <«t < endl;
cout << a << " - " «t < " = " << a-t << endl;
" << t << endl;
cout << a <<" * "
:< " = " << t« endl;
c = 2;
t = a / c;
cout << a << " /"<c << " = "<<t<< endl;
cout << a <<" - "<< t << " = "<< a-t << endl;
if (a -- b)
cout << a << " = " « b << endl;
else
cout << a « " not e qual to " « b << endl;
if (a == b)
cout << a <<
return 0;
" = "<< b << endl;
else
Program written by: Ron Sha
Course Info: CS-116 2021SP
Date: Fri Mar 05 09:02:39 2021
cout << a << "not equal to "< b << endl;
return 0;
Original value is $2.02
50% of value is $1.01
10 times value is $20.20
Your program should have similar output:
Testing arithmetic operators:
$8.75 + $5.80
$8.75
$5.80
$8.75 * 2 = $17.50
$8.75 / 2 = $4.37
$8.75
$8.75 not equal to $5.80
$14.55
$2.95
$2.95
%3D
$5.80
$8.75 = -
$4.37 = $4.38
2.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
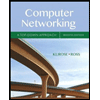
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
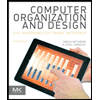
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
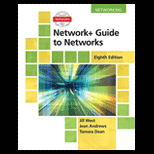
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
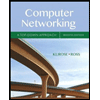
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
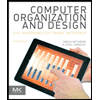
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
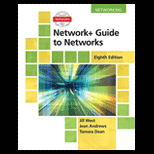
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
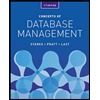
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
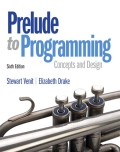
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
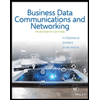
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY