Write a class named Patient that has member variables for first name, middle name, last name, phone number, name and phone number of emergency contact. The Patient class should have a constructor that accepts and argument for each member variable. The Patient class should also have accessor and mutator functions for each member variable. Write a class named Procedure that has member variables for name of procedure, date of procedure, name of physician, and charges for the procedure. This Procedure class represents a medical procedure that is performed on a patient. The Procedure class should have a constructor that accepts an argument for each member variable. The Procedure class should also have accessor and mutator functions for each member variable. Then write a program that creates an instance of the Patient class initialized with sample data that is entered by the user. Then, create an instance of the Procedure class for each procedure that is being performed on the patient. There can be multiple procedures that can be performed on each patient. At the end of the data entry for a patient, display the patient’s information, information about the procedure/s and the total charges for the patient. public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("First Name: "); String firstName = input.nextLine(); System.out.println("Middle Name: "); String middleName = input.nextLine(); System.out.println("Last Name: "); String lastName = input.nextLine(); System.out.println("Phone Number: "); String phoneNumber = input.nextLine(); System.out.println("Emergency Contact Name: "); String emerContName = input.nextLine(); System.out.println("Emergency Contact Phone Number: "); String emerContPhoneNumber = input.nextLine(); Patient patient = new Patient(firstName, middleName, lastName, phoneNumber, emerContName, emerContPhoneNumber); List proceduresList = new ArrayList(); double totalProceduresCost = 0; while(true){ System.out.println(); System.out.println("Enter procedure info..."); System.out.println(); System.out.println(); System.out.println("Name of procedure: "); String nameOfProcedure = input.nextLine(); System.out.println("Day of procedure: "); String dayOfProcedure = input.nextLine(); System.out.println("Name of physician: "); String nameOfPhysician = input.nextLine(); System.out.println("Charges for the procedure:"); double chargesForTheProcedure = Double.valueOf(input.nextLine()); proceduresList.add(new Procedure(nameOfProcedure, dayOfProcedure, nameOfPhysician, chargesForTheProcedure)); System.out.println(); System.out.print("Enter another Procedure? 1 for Yes, 2 for No: "); System.out.println(); int anotherProcedure = input.nextInt(); input.nextLine(); if(anotherProcedure == 2){ break; } } System.out.println(); System.out.println("\n-------------------------------------------------------"); System.out.println("PATIENT REPORT"); System.out.println("\n-------------------------------------------------------"); System.out.println(); System.out.println("Patient: " + patient.getFirstName() + patient.getMiddleName() + patient.getLastName()); System.out.println("Phone number of patient:" + patient.getPhoneNumber()); System.out.println(); System.out.println(); System.out.println("Emergency contact name: " + patient.getEmerContName()); System.out.println("Emergency contact phone number:" + patient.getEmerContPhoneNumber()); System.out.println(); for (int procedureNo = 1; procedureNo <= proceduresList.size(); procedureNo++) { Procedure procedure = proceduresList.get(procedureNo - 1); totalProceduresCost += procedure.getChargesForTheProcedure(); System.out.println("Procedure:" + procedureNo); System.out.println("name of procedure: " + procedure.getNameOfProcedure()); System.out.println("Day of procedure: " + procedure.getDayOfProcedure()); System.out.println("Name of physician: " + procedure.getNameOfPhysician()); System.out.println("Charges for the procedure: $" + procedure.getChargesForTheProcedure()); System.out.println("\n-------------------------------------------------------"); } System.out.println(); System.out.println("Total charge for the patient is: $" + totalProceduresCost); } } Here is my code, my professor said everything is good. I just need you guys help me right here. " System.out.println("Patient: " + patient.getFirstName() + patient.getMiddleName() + patient.getLastName());". How can I split FISRT, MIDDLE AND LAST NAME when I run the program ? The laguage I am using is java.
Write a class named Patient that has member variables for first name, middle name, last name, phone number, name and phone number of emergency contact. The Patient class should have a constructor that accepts and argument for each member variable. The Patient class should also have accessor and mutator functions for each member variable. Write a class named Procedure that has member variables for name of procedure, date of procedure, name of physician, and charges for the procedure. This Procedure class represents a medical procedure that is performed on a patient. The Procedure class should have a constructor that accepts an argument for each member variable. The Procedure class should also have accessor and mutator functions for each member variable. Then write a program that creates an instance of the Patient class initialized with sample data that is entered by the user. Then, create an instance of the Procedure class for each procedure that is being performed on the patient. There can be multiple procedures that can be performed on each patient. At the end of the data entry for a patient, display the patient’s information, information about the procedure/s and the total charges for the patient.
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("First Name: ");
String firstName = input.nextLine();
System.out.println("Middle Name: ");
String middleName = input.nextLine();
System.out.println("Last Name: ");
String lastName = input.nextLine();
System.out.println("Phone Number: ");
String phoneNumber = input.nextLine();
System.out.println("Emergency Contact Name: ");
String emerContName = input.nextLine();
System.out.println("Emergency Contact Phone Number: ");
String emerContPhoneNumber = input.nextLine();
Patient patient = new Patient(firstName, middleName, lastName, phoneNumber, emerContName, emerContPhoneNumber);
List<Procedure> proceduresList = new ArrayList();
double totalProceduresCost = 0;
while(true){
System.out.println();
System.out.println("Enter procedure info...");
System.out.println();
System.out.println();
System.out.println("Name of procedure: ");
String nameOfProcedure = input.nextLine();
System.out.println("Day of procedure: ");
String dayOfProcedure = input.nextLine();
System.out.println("Name of physician: ");
String nameOfPhysician = input.nextLine();
System.out.println("Charges for the procedure:");
double chargesForTheProcedure = Double.valueOf(input.nextLine());
proceduresList.add(new Procedure(nameOfProcedure, dayOfProcedure, nameOfPhysician, chargesForTheProcedure));
System.out.println();
System.out.print("Enter another Procedure? 1 for Yes, 2 for No: ");
System.out.println();
int anotherProcedure = input.nextInt();
input.nextLine();
if(anotherProcedure == 2){
break;
}
}
System.out.println();
System.out.println("\n-------------------------------------------------------");
System.out.println("PATIENT REPORT");
System.out.println("\n-------------------------------------------------------");
System.out.println();
System.out.println("Patient: " + patient.getFirstName() + patient.getMiddleName() + patient.getLastName());
System.out.println("Phone number of patient:" + patient.getPhoneNumber());
System.out.println();
System.out.println();
System.out.println("Emergency contact name: " + patient.getEmerContName());
System.out.println("Emergency contact phone number:" + patient.getEmerContPhoneNumber());
System.out.println();
for (int procedureNo = 1; procedureNo <= proceduresList.size(); procedureNo++) {
Procedure procedure = proceduresList.get(procedureNo - 1);
totalProceduresCost += procedure.getChargesForTheProcedure();
System.out.println("Procedure:" + procedureNo);
System.out.println("name of procedure: " + procedure.getNameOfProcedure());
System.out.println("Day of procedure: " + procedure.getDayOfProcedure());
System.out.println("Name of physician: " + procedure.getNameOfPhysician());
System.out.println("Charges for the procedure: $" + procedure.getChargesForTheProcedure());
System.out.println("\n-------------------------------------------------------");
}
System.out.println();
System.out.println("Total charge for the patient is: $" + totalProceduresCost);
}
}
Here is my code, my professor said everything is good. I just need you guys help me right here. " System.out.println("Patient: " + patient.getFirstName() + patient.getMiddleName() + patient.getLastName());". How can I split FISRT, MIDDLE AND LAST NAME when I run the program ? The laguage I am using is java.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

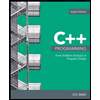
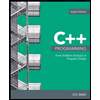