Language is c++ Assignment 6 C: Minesweeper - Simplified. For many years, computers sold with the Windows operating system would contain a game called M inesweeper. The player would be presented with a grid, where they would have to click an empty part of the map. If they clicked on a hidden mine, the game would be instantly over. However, if they clicked a safe spot, a hint about nearby mines would be displayed and the player would click another spot. The goal would be to flag all the hidden mines without hitting one. (As an aside, many people did not know these rules and just clicked around randomly until they hit a mine) We will be developing a simplified version of this game. You will prompt the user for a grid size and then created a 2D array with equal width and height (C++ students: Check the Appendix for more information on how to do this). You will initialize the 2D char array with each element equallying a ‘?’ symbol. You will then randomly generate one “mine” value per column in the 2D and store their row locations in a separate 1D array (the 1D array’s index will represent the column of the 2D array). The player will be prompted the user to enter an X and Y coordinate on the grid. If the space is "free”, you will replace the value at that index with a ‘_’ symbol. If the space has a hidden mine, you will place an ‘X’ symbol at that index instead. Afterwards, print the 2D array again. If the player hit a mine, tell them they lost and that the game is over. Otherwise, conduct a linear search of the 2D array and count how many ‘_’ symbols there are (Hint: Use a nested FOR loop). If the count equals the grid size, tell the player they won. Hint: Reference your code from past Assignments as a starting point for this one. I included sample outputs in the pictures
Language is c++
Assignment 6 C: Minesweeper - Simplified. For many years, computers sold with the Windows
We will be developing a simplified version of this game. You will prompt the user for a grid size and then created a 2D array with equal width and height (C++ students: Check the Appendix for more information on how to do this). You will initialize the 2D char array with each element equallying a ‘?’ symbol. You will then randomly generate one “mine” value per column in the 2D and store their row locations in a separate 1D array (the 1D array’s index will represent the column of the 2D array).
The player will be prompted the user to enter an X and Y coordinate on the grid. If the space is "free”, you will replace the value at that index with a ‘_’ symbol. If the space has a hidden mine,
you will place an ‘X’ symbol at that index instead. Afterwards, print the 2D array again.
If the player hit a mine, tell them they lost and that the game is over. Otherwise, conduct a linear search of the 2D array and count how many ‘_’ symbols there are (Hint: Use a nested FOR loop). If the count equals the grid size, tell the player they won.
Hint: Reference your code from past Assignments as a starting point for this one.
I included sample outputs in the pictures
![Sample Output #2:
[Minesweeper - DOS Edition]
What is the grid size? 3
???
???
???
Enter your X coordinate: o
Enter your Y coordinate: 0
_??
???
???
Enter your X coordinate: 1
Enter your Y coordinate: 1
_??
?_?
???
Enter your X coordinate: 1
Enter your Y coordinate: 2
_??
?_?
?_?
You win!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe799f64b-1f0b-4ff1-9ee3-5090ddf07729%2Fce60e1b1-f4af-4566-9ca4-788d1559b3a5%2Fctg0gop_processed.png&w=3840&q=75)
![Sample Output #1:
[Minesweeper - DOS Edition]
What is the grid size? 6
??????
??????
??????
??????
??????
??????
Enter your X coordinate: 2
Enter your Y coordinate: 5
??????
??????
??????
??????
??????
??X???
Sorry, you hit a mine!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe799f64b-1f0b-4ff1-9ee3-5090ddf07729%2Fce60e1b1-f4af-4566-9ca4-788d1559b3a5%2F7u69l29_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

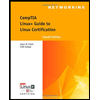
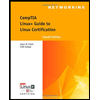