List manipulation with exception handling! In this assignment, you are given one list to perform different types of operations in each function. All the functions are provided, you need to find out the kind of exceptions that can happen while performing different operations on the elements and your task is to fill out the code with python exception handling mechanism wherever appropriate.
List manipulation with exception handling! In this assignment, you are given one list to perform different types of operations in each function. All the functions are provided, you need to find out the kind of exceptions that can happen while performing different operations on the elements and your task is to fill out the code with python exception handling mechanism wherever appropriate.
Chapter11: Exception Handling
Section: Chapter Questions
Problem 2CP
Related questions
Question
make sure the code follows template code
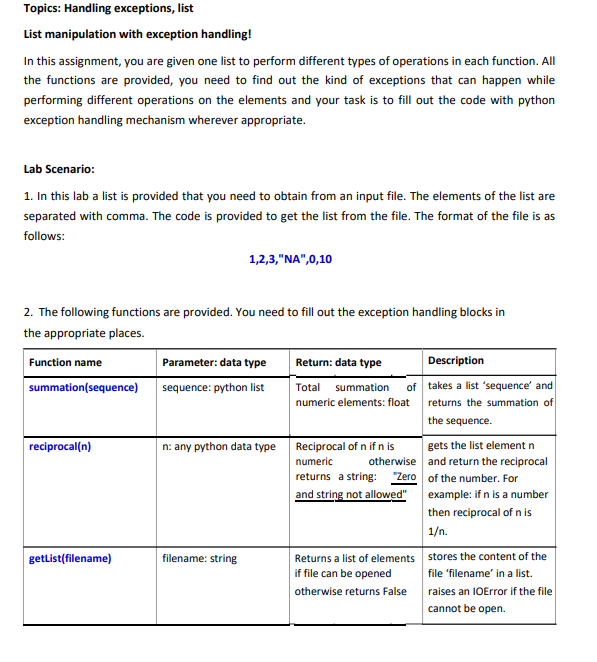
Transcribed Image Text:Topics: Handling exceptions, list
List manipulation with exception handling!
In this assignment, you are given one list to perform different types of operations in each function. All
the functions are provided, you need to find out the kind of exceptions that can happen while
performing different operations on the elements and your task is to fill out the code with python
exception handling mechanism wherever appropriate.
Lab Scenario:
1. In this lab a list is provided that you need to obtain from an input file. The elements of the list are
separated with comma. The code is provided to get the list from the file. The format of the file is as
follows:
1,2,3,"NA",0,10
2. The following functions are provided. You need to fill out the exception handling blocks in
the appropriate places.
Function name
Parameter: data type
Return: data type
Description
of takes a list 'sequence' and
returns the summation of
summation(sequence)
sequence: python list
Total summation
numeric elements: float
the sequence.
reciprocal(n)
n: any python data type
Reciprocal of n if n is
gets the list element n
numeric
otherwise and return the reciprocal
returns a string: "Zero of the number. For
and string not allowed"
example: if n is a number
then reciprocal of n is
1/n.
getlist(filename)
filename: string
Returns a list of elements stores the content of the
if file can be opened
file 'filename' in a list.
otherwise returns False
raises an IOError if the file
cannot be open.
![def summation (sequence):
6. For this lab, you are expected to use python exception handling mechanism try-except block. The
Input: sequence: python list
Return: summation of the numeric elements: int or float
total sum = 0
for s in sequence:
total_sum += float(s)
return total_sum
def reciprocal(n):
above functions are provided, you need to fill out the functions with try-except statements
Input: n: int or float
Returns: reciprocal of n if n is int or float otherwise
wherever needed. One sample code can be as follows:
returns error message
return 1/float(n)
w/0 exception handling (user might enter With try-except block
some non-number values)
try:
def getlist(filename):
x = input("Enter a number: ")
print ("You entered a number")
x = input("Enter a number: ")
Input: filename: string
print ("You entered a number")
Returns: list: content of the file in list
except:
if file can be opened otherwise returns False
print ("Not a valid number. ")
f = open(filename)
temp = f.readline()
return [t.split('\n')[0] for t in temp.split(',') ] #returns the sequence
Sample Output for the given Input file:
def main():
This is just a sample output, you can choose to print other messages as you like, but the code should
sequence = getlist('LA9.txt')
if sequence == False:
have exception handling implemented properly so that it gives you the right output.
print ("File I/0 error")
The sequence is: ['1', '2', '3', "'NA", 'O', '10', '100.0', '23']
**** Calculating Summation ****
Not a number, continue to next element
139.0
****
Calculating Reciprocal ****
else:
print('The sequence is: ', sequence)
print ("**** Calculating Summation ****")
print (summation(sequence))
1:1.0
2:0.5
3:0.3333333333333333
print (" **** Calculating Reciprocal ****")
for s in sequence:
"NA" : Zero and string not allowed
0: Zero and string not allowed
10 : 0.1
100.0 : 0.01
23 : 0.043478260869565216
print ('{} : {}'.format(s , reciprocal(s)))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9eb2eb60-aea6-4c2b-bdb3-b0b3ca45de12%2F1a2bb158-456c-487b-9d4e-dcc0037e696c%2Fbrr46c6_processed.png&w=3840&q=75)
Transcribed Image Text:def summation (sequence):
6. For this lab, you are expected to use python exception handling mechanism try-except block. The
Input: sequence: python list
Return: summation of the numeric elements: int or float
total sum = 0
for s in sequence:
total_sum += float(s)
return total_sum
def reciprocal(n):
above functions are provided, you need to fill out the functions with try-except statements
Input: n: int or float
Returns: reciprocal of n if n is int or float otherwise
wherever needed. One sample code can be as follows:
returns error message
return 1/float(n)
w/0 exception handling (user might enter With try-except block
some non-number values)
try:
def getlist(filename):
x = input("Enter a number: ")
print ("You entered a number")
x = input("Enter a number: ")
Input: filename: string
print ("You entered a number")
Returns: list: content of the file in list
except:
if file can be opened otherwise returns False
print ("Not a valid number. ")
f = open(filename)
temp = f.readline()
return [t.split('\n')[0] for t in temp.split(',') ] #returns the sequence
Sample Output for the given Input file:
def main():
This is just a sample output, you can choose to print other messages as you like, but the code should
sequence = getlist('LA9.txt')
if sequence == False:
have exception handling implemented properly so that it gives you the right output.
print ("File I/0 error")
The sequence is: ['1', '2', '3', "'NA", 'O', '10', '100.0', '23']
**** Calculating Summation ****
Not a number, continue to next element
139.0
****
Calculating Reciprocal ****
else:
print('The sequence is: ', sequence)
print ("**** Calculating Summation ****")
print (summation(sequence))
1:1.0
2:0.5
3:0.3333333333333333
print (" **** Calculating Reciprocal ****")
for s in sequence:
"NA" : Zero and string not allowed
0: Zero and string not allowed
10 : 0.1
100.0 : 0.01
23 : 0.043478260869565216
print ('{} : {}'.format(s , reciprocal(s)))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
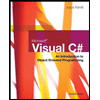
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
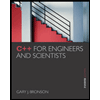
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
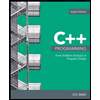
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
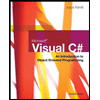
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
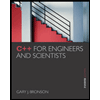
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
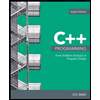
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning