Write an equals method for the StepsFitnessTracker class that tests whether the one object is equal to another object that is passed as an argument (Object otherObject). The Object superclass provides an equals method that tests if two object references are equal. Your new equals method should override the Object equals method, and should test the following: • Return true if the current (this) object, and otherObject are identical references (hint: you can use the == operator for this). • Returns false if otherObject is null. • Returns false if the current (this) object and otherObject have different classes (hint: you can use the getClass() method provided by the Object superclass, see the Java 8 API documentation for details). • Casts otherObject to a StepsFitnessTracker, and tests for equality of each instance variable1 , if the instance variables are the same the method should return true. Now write an equals method for the DistanceFitnessTracker and HeartRateFitnessTracker classes that: • Use the superclass equals method, and return false if this method fails. • Casts the parameter to either HeartRateFitnessTracker or DistanceFitnessTracker. • Tests for equality of the subclass instance fields. Please, use JUnit testing and write a test class named TestFTEquals to test that these new equals methods work properly. For inspiration, you can have a look at the test classes provided (TestFitnessTracker, TestDistanceFitnessTracker, TestStepsFitnessTracker, and TestHeartRateFitnessTracker) as they are already providing you some basic tests about how to test for equality of objects. I already did the first part and added the equal method I need help to write the TESTFTEQUALS TEST PLease public class HeartRateFitnessTracker extends FitnessTracker { // Cumulative moving average HeartRate HeartRate avgHeartRate; // Number of heart rate measurements int numMeasurements; public HeartRateFitnessTracker(String modelName, HeartRate heartRate) { super(modelName); // Only one HeartRate to begin with; average is equal to single measurement this.avgHeartRate = heartRate; this.numMeasurements = 1; } public void addHeartRate(HeartRate heartRateToAdd) { // Calculate cumulative moving average of heart rate // See https://en.wikipedia.org/wiki/Moving_average double newHR = heartRateToAdd.getValue(); double cmaHR = this.avgHeartRate.getValue(); double cmaNext = (newHR + (cmaHR * numMeasurements)) / (numMeasurements + 1); this.avgHeartRate.setValue(cmaNext); numMeasurements ++; } // Getter for average heart rate public HeartRate getAvgHeartRate() { return avgHeartRate; } public String toString() { return "Heart Rate Tracker " + getModelName() + "; Average Heart Rate: " + getAvgHeartRate().getValue() + ", for " + numMeasurements + " measurements"; } @Override public boolean equals(Object obj) { // TODO Implement a method to check equality if(this == obj) // it will check if both the references are refers to same object return true; if(obj == null || obj.getClass()!= this.getClass()) //it check the argument of the type HeartRateFitnessTracker by comparing the classes of the passed argument and this object. return false; HeartRateFitnessTracker HRFT=(HeartRateFitnessTracker)obj; // type casting of the argument. return (HRFT.avgHeartRate == this.avgHeartRate);// comparing the state of argument with the state of 'this' Object. } } public class DistanceFitnessTracker extends FitnessTracker{ private Distance totalDistance; public DistanceFitnessTracker(String modelName, Distance distance) { super(modelName); this.totalDistance = distance ; } public Distance getDistance() { return totalDistance; } public void addDistance(Distance distance) { this.totalDistance.setValue(this.totalDistance.getValue() + distance.getValue()); } public Distance getTotalDistance() { return totalDistance; } public String toString() { return "Distance Tracker " + getModelName() + "; Total Distance: " + getTotalDistance().getValue(); } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; if (!super.equals(obj)) return false; DistanceFitnessTracker that = (DistanceFitnessTracker) obj; if(this.getDistance() == null && that.getDistance() != null) return false; if(this.getDistance() != null && that.getDistance() == null) return false; if(that.getDistance() != this.getDistance()) return false; return that.getDistance().getValue() == this.getDistance().getValue(); } }
Urgent Quick Question
Write an equals method for the StepsFitnessTracker class that tests whether the one object is equal to another object that is passed as an argument (Object otherObject). The Object superclass provides an equals method that tests if two object references are equal. Your new equals method should override the Object equals method, and should test the following: • Return true if the current (this) object, and otherObject are identical references (hint: you can use the == operator for this). • Returns false if otherObject is null. • Returns false if the current (this) object and otherObject have different classes (hint: you can use the getClass() method provided by the Object superclass, see the Java 8 API documentation for details). • Casts otherObject to a StepsFitnessTracker, and tests for equality of each instance variable1 , if the instance variables are the same the method should return true. Now write an equals method for the DistanceFitnessTracker and HeartRateFitnessTracker classes that: • Use the superclass equals method, and return false if this method fails. • Casts the parameter to either HeartRateFitnessTracker or DistanceFitnessTracker. • Tests for equality of the subclass instance fields. Please, use JUnit testing and write a test class named TestFTEquals to test that these new equals methods work properly. For inspiration, you can have a look at the test classes provided (TestFitnessTracker, TestDistanceFitnessTracker, TestStepsFitnessTracker, and TestHeartRateFitnessTracker) as they are already providing you some basic tests about how to test for equality of objects.
I already did the first part and added the equal method I need help to write the TESTFTEQUALS TEST PLease
public class HeartRateFitnessTracker extends FitnessTracker {
// Cumulative moving average HeartRate
HeartRate avgHeartRate;
// Number of heart rate measurements
int numMeasurements;
public HeartRateFitnessTracker(String modelName, HeartRate heartRate) {
super(modelName);
// Only one HeartRate to begin with; average is equal to single measurement
this.avgHeartRate = heartRate;
this.numMeasurements = 1;
}
public void addHeartRate(HeartRate heartRateToAdd) {
// Calculate cumulative moving average of heart rate
// See https://en.wikipedia.org/wiki/Moving_average
double newHR = heartRateToAdd.getValue();
double cmaHR = this.avgHeartRate.getValue();
double cmaNext = (newHR + (cmaHR * numMeasurements)) / (numMeasurements + 1);
this.avgHeartRate.setValue(cmaNext);
numMeasurements ++;
}
// Getter for average heart rate
public HeartRate getAvgHeartRate() {
return avgHeartRate;
}
public String toString() {
return "Heart Rate Tracker " + getModelName() +
"; Average Heart Rate: " + getAvgHeartRate().getValue() +
", for " + numMeasurements + " measurements";
}
@Override
public boolean equals(Object obj) {
// TODO Implement a method to check equality
if(this == obj) // it will check if both the references are refers to same object
return true;
if(obj == null || obj.getClass()!= this.getClass()) //it check the argument of the type HeartRateFitnessTracker by comparing the classes of the passed argument and this object.
return false;
HeartRateFitnessTracker HRFT=(HeartRateFitnessTracker)obj; // type casting of the argument.
return (HRFT.avgHeartRate == this.avgHeartRate);// comparing the state of argument with the state of 'this' Object.
}
}
public class DistanceFitnessTracker extends FitnessTracker{
private Distance totalDistance;
public DistanceFitnessTracker(String modelName, Distance distance) {
super(modelName);
this.totalDistance = distance ;
}
public Distance getDistance() {
return totalDistance;
}
public void addDistance(Distance distance) {
this.totalDistance.setValue(this.totalDistance.getValue() + distance.getValue());
}
public Distance getTotalDistance() {
return totalDistance;
}
public String toString() {
return "Distance Tracker " + getModelName() +
"; Total Distance: " + getTotalDistance().getValue();
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
if (!super.equals(obj)) return false;
DistanceFitnessTracker that = (DistanceFitnessTracker) obj;
if(this.getDistance() == null && that.getDistance() != null) return false;
if(this.getDistance() != null && that.getDistance() == null) return false;
if(that.getDistance() != this.getDistance()) return false;
return that.getDistance().getValue() == this.getDistance().getValue();
}
}
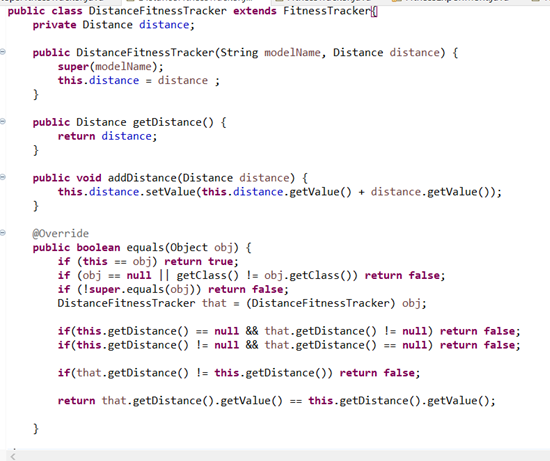
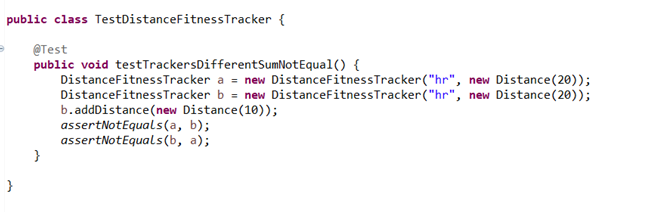

Step by step
Solved in 2 steps

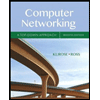
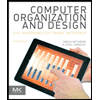
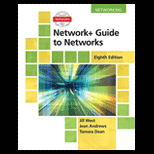
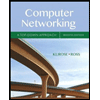
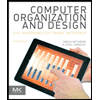
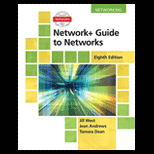
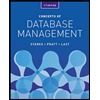
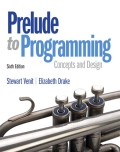
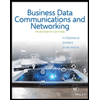