MATCH OUTPUT AS IT IS . FOLLOW QUESTION ----------------------------------------- Dr. Varun needs an application for his clinic to maintain all the appointments for the day. The application should make appointments on the basis of first come first serve basis. And it should also perform the following functions: insert new appointment, delete an appointment, display all the appointments, show next appointment, check if there is no appointment or number of appointments are full for a day. Use class templates to perform the above-mentioned operations. Write a C++ program to perform the Queue operation. Note: Use the following class template for Queue creation. template class Queue { private: int front,rear; T *queue; int maxsize; }; Define the following function in the class Queue class Method name Description int isFull() The method is used to check whether the queue is full or not. void insert(T) The method is used to display the rear element in the queue (if the queue is stored with the element(s)). void deletion() The method is used to delete the front element from the queue. void atFront() The method is used to display the front element in the queue (if the queue is stored with the element(s)). void atRear() The method is used to add data to the rear end of the queue. void display() The method is used to display all the data in the queue. int isEmpty() The method is used to check whether the queue is empty or not. Input and Output Format: The first line of input corresponds to the size of the array. Refer sample input and output for formatting specifications. Sample Input and Output : [All text in bold corresponds to input and the rest corresponds to output] Enter the array size 3 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:1 Enter the element to insert : 1 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:1 Enter the element to insert : 2 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:1 Enter the element to insert : 3 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:1 Enter the element to insert : 4 Queue is full! 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:5 Queue elements are : 1 2 3 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:3 Front element of the queue is : 1 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:4 Rear element of the queue is : 3 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:2 Deleted element of the queue is : 1 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:5 Queue elements are : 2 3 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:3 Front element of the queue is : 2 1.Insertion 2.Deletion 3.Display Front Element 4.Display Rear Element 5.Display Queue 6.Exit Enter your Choice:6
MATCH OUTPUT AS IT IS . FOLLOW QUESTION
-----------------------------------------
Dr. Varun needs an application for his clinic to maintain all the appointments for the day. The application should make appointments on the basis of first come first serve basis. And it should also perform the following functions: insert new appointment, delete an appointment, display all the appointments, show next appointment, check if there is no appointment or number of appointments are full for a day. Use class templates to perform the above-mentioned operations.
Write a C++ program to perform the Queue operation.
Note:
Use the following class template for Queue creation.
template <class T>
class Queue
{
private:
int front,rear;
T *queue;
int maxsize;
};
Define the following function in the class Queue class
Method name | Description |
int isFull() | The method is used to check whether the queue is full or not. |
void insert(T) | The method is used to display the rear element in the queue (if the queue is stored with the element(s)). |
void deletion() | The method is used to delete the front element from the queue. |
void atFront() | The method is used to display the front element in the queue (if the queue is stored with the element(s)). |
void atRear() | The method is used to add data to the rear end of the queue. |
void display() | The method is used to display all the data in the queue. |
int isEmpty() | The method is used to check whether the queue is empty or not. |
The first line of input corresponds to the size of the array.
Sample Input and Output :
[All text in bold corresponds to input and the rest corresponds to output]
Enter the array size
3
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:1
Enter the element to insert : 1
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:1
Enter the element to insert : 2
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:1
Enter the element to insert : 3
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:1
Enter the element to insert : 4
Queue is full!
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:5
Queue elements are : 1 2 3
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:3
Front element of the queue is : 1
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:4
Rear element of the queue is : 3
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:2
Deleted element of the queue is : 1
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:5
Queue elements are : 2 3
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:3
Front element of the queue is : 2
1.Insertion
2.Deletion
3.Display Front Element
4.Display Rear Element
5.Display Queue
6.Exit
Enter your Choice:6

Step by step
Solved in 3 steps with 1 images

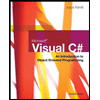
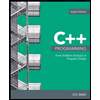
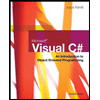
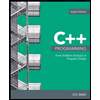