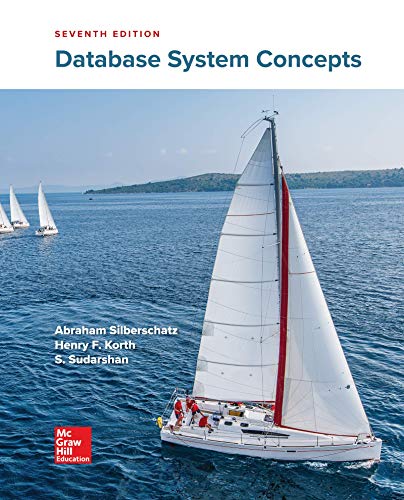
Write a function named “endingWithSlashSlash” that accepts the same
parameters as command line arguments (argc and argv). It returns true if at least
one of the arguments have a “//” at the end and false otherwise.
This function also returns the number of arguments that have “//” at the end.
For example,
“//” will return true and 1
“one// two three// four” will return true and 2
“one” will return false and 0
“one two” will return false and 0
Please note that for question #5, you cannot refer or use string class, string
methods such length() or size() or any string function such as strlen(), strcmp
or strstr(). Please use only array notation, pointer to character, and/or pointer
arithmetic and make use of the fact that this is a C-string.

Answer:
I have done code and also I have attached code and code screenshot as well as output
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Alert dont submit AI generated answer.arrow_forwardWrite a full program that display the area of a rectangle. The program calls the following two functions: • getLengthWidth – This function uses a reference parameter variables to accept an int arguments length and width. This function should ask the user to enter the rectangle’s length and width. Input validation: length and width should be positive integers. • calArea – This function should accept the rectangle’s length and width as arguments and return the rectangle’s area. The area is calculated by multiplying the (length * width).arrow_forwardWrite a function named “HasTheSameEndingChar” that accepts 2parameters the same as command line arguments (argc and argv). It will return true if command line arguments that has the same ending character. If the user has provided no argument or only one argument, it will also return true. If any of the arguments does not end with same character, it will return false. This function should not have cin or cout. This function cannot use string class, either. Write a complete C++ program that accepts command line arguments and call the above function. It will print out “Yes” if the function returns true and “No” if it returns false. Important requirement: This program cannot include header file and cannot use string class and its methods such as “length” or “size” This program requires only the use of array, C-string and pointers No global variable is allowed to be declared and used For example, the following runs will print Yes, Yes, Yes, Yes, No, No, Noand…arrow_forward
- Examples Input 8 Output How about visiting Gibraltar Rock? It is about 8 miles away. Input 30 Output How about visiting Oxnard? It is about 30 miles away. Input 120 Output You provided an invalid distance that is not between 0 and 100 miles. Input -100 Output You provided an invalid distance that is not between 0 and 100 miles. Hints • you can check the type of an input parameter by using the type () function.arrow_forwardHere is the main function in a program int main () { int x = 30; //more code goes here someFunction (x) ; cout « x; return 0; } Assume the heading for someFunction is written correctly with an integer parameter named x, and that this is the body of that function: { x = x * 20; //more code goes here } What will be the output? Just enter a number. If there would be an error, enter -999arrow_forwardAdd a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forward
- //Write a function that loops through and console.log's the numbers from 1 to 100, except multiples of three, log (without quotes) "VERY GOOD" instead of the number, for the multiples of five, log (without quotes) "SUPER AWESOME". For numbers which are multiples of both three and five, log (without quotes) "VERY GOOD SUPER AWESOME" Console log out: 12VERY GOOD4SUPER AWESOMEVERY GOOD78VERY GOODSUPER AWESOME11VERY GOOD1314VERY GOOD SUPER AWESOME1617VERY GOOD19SUPER AWESOMEVERY GOOD2223VERY GOODSUPER AWESOME26VERY GOOD2829VERY GOOD SUPER AWESOMEarrow_forwardWrite in Python Write a function named max that accepts two float values as arguments and returns the value that is the greater of the two. For example, if 7.2 and 12.1 are passed as arguments to the function, the function should return 12.1. Use the function in a program that prompts the user to enter two float values. The program should display the value that is the greater of the two.arrow_forwardX609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forward
- Write a function that has a single input argument grade. If the user's input is a numeric number, the grade letter should be displayed to the command window depending on the value of grade, as shown in the table below. Otherwise, MATLAB should stop the execution and send an error message: "invalid input" to the command window. Grade Grade Letter Grade 2 90 A 90 > Grade 2 80 80 > Grade > 70 70> Grade 2 60 60> Grade Grade >100 or Unknown Grade using namespace std; int main) ( int score; char grade; coutss"Enter score: "; cin>>score; if(score100X cout<arrow_forwardWrite a set of functions that calculate the cost of movie tickets for a family. Using a loop, keep asking: The age of the movie goer. Based on this age, you will charge them these amounts: Under age 3, the ticket is free. If they are between 3 and 12, the ticket is $10.25. If they are over age 12, the ticket is $15.50. If they are 55 or older, the ticket is $12. If they buy at least 4 tickets, they receive a 20% discount. Then, keep a running_total that is output to the screen after each age has been entered. This running_total should be accessed through the functions using a pass-by-reference variable: &running_total The other function parameter variables are to be pass-by-value. Tell them to enter a zero when they want to stop. Finally, calculate the total cost including a 7.5% sales tax. and output it to the screen. Be sure that the code is broken up into functions with good comments. Put as little code in main() as you can get away with and still make sense.…arrow_forwardWrite a function called ConvertToFootInchMeasure (signature below) that takes a parameter for the total inch measure. The function should return the converted measure as a formatted string (with the " and ' as appropriate). Use the following header: string ConvertToFootInchMeasure (int totalInches)/* Pre: totalInches the total number of inchesPost: The converted, formatted measure is returned */arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
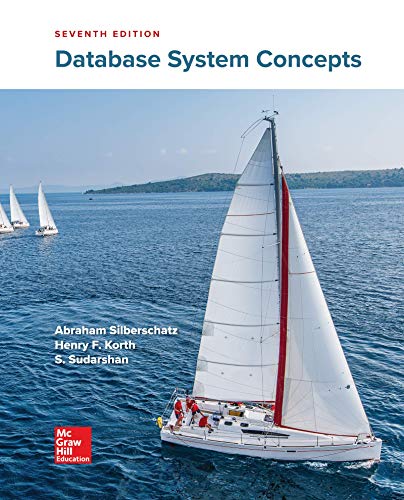
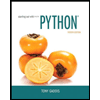
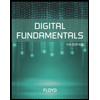
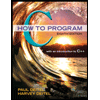
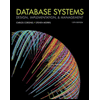
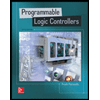