MATCH OUTPUT AS IT IS --------------------------- Write a C++ program to add, sort, and display the details of a user in an array and also to remove the detail in an array. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class User has the following private attributes/member variables Data type Variable string name long long int mobileNumber string username string password Include appropriate constructors for the above class. The class UserBO has the following functions: Member Function Description void adddetails(string name,long long int mobilenumber,string username,string password,int i) The function to add the details of a user in an array object. void sortdetails(int n) The function to sort and display the user details void removeuser(string name, int n) The function to remove the user detail present at the index entered from the array Declare an array in the BO class to store the user details In the main method, create an array of user instances/objects and call the above methods. Input format: The first input corresponds to the name of a user. The second input corresponds to the mobile number. The third input corresponds to the username. The fourth input corresponds to the password. Output format: The output consists of sorting and displaying all the input details. Refer sample input and output for formatting specifications. [All text in bold corresponds to input and the rest corresponds to output] Sample Input and Output 1: Enter the number of users: 2 Enter name: sujatha Enter mobile number: 8909012345 Enter the username: sujauser Enter the password: qwerty Enter name: abinaya Enter mobile number: 8000543250 Enter the username: abi123 Enter the password: passabinaya MENU: 1.To sort and display the user details. 2.To remove the user detail. Enter your choice: 1 User details after Sorting Name Mobilenumber Username Password abinaya 8000543250 abi123 passabinaya sujatha 8909012345 sujauser qwerty Do you wish to continue(y/n): y MENU: 1.To sort and display the user details. 2.To remove the user detail. Enter your choice: 2 Enter the name to be deleted: abinaya Deleted successfully Do you wish to continue(y/n): n Sample Input and Output 2: Enter the number of users: 3 Enter name: usha Enter mobile number: 9078904561 Enter the username: usha12345 Enter the password: ushashankar Enter name: aanand Enter mobile number: 9854432216 Enter the username: aan123aan Enter the password: aanandsunrise Enter name: tina Enter mobile number: 9842234456 Enter the username: tinaforyou Enter the password: qwertypass MENU: 1.To sort and display the user details. 2.To remove the user detail. Enter your choice: 2 Enter the name to be deleted: tina Deleted successfully Do you wish to continue(y/n): y MENU: 1.To sort and display the user details. 2.To remove the user detail. Enter your choice: 1 User details after Sorting Name Mobilenumber Username Password aanand 9854432216 aan123aan aanandsunrise usha 9078904561 usha12345 ushashankar Do you wish to continue(y/n): n Sample Input and Output 3: Enter the number of users: 2 Enter name: siva Enter mobile number: 9870056700 Enter the username: siva123 Enter the password: sivakumar Enter name: krishna Enter mobile number: 8900989011 Enter the username: krish_na Enter the password: krish1234 MENU: 1.To sort and display the user details. 2.To remove the user detail. Enter your choice: 1 User details after Sorting Name Mobilenumber Username Password krishna 8900989011 krish_na krish1234 siva 9870056700 siva123 sivakumar Do you wish to continue(y/n): n Sample Input and Output 4: Enter the number of users: 2 Enter name: kumar Enter mobile number: 9879090867 Enter the username: kumar_123_user Enter the password: kitkat Enter name: joey Enter mobile number: 7012588700 Enter the username: user_joey Enter the password: joey1990 MENU: 1.To sort and display the user details. 2.To remove the user detail. Enter your choice: 2 Enter the name to be deleted: joey Deleted successfully Do you wish to continue(y/n): n
MATCH OUTPUT AS IT IS
---------------------------
Write a C++ program to add, sort, and display the details of a user in an array and also to remove the detail in an array.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
The class User has the following private attributes/member variables
Data type | Variable |
string | name |
long long int | mobileNumber |
string | username |
string | password |
Include appropriate constructors for the above class.
The class UserBO has the following functions:
Member Function | Description |
void adddetails(string name,long long int mobilenumber,string username,string password,int i) | The function to add the details of a user in an array object. |
void sortdetails(int n) | The function to sort and display the user details |
void removeuser(string name, int n) |
The function to remove the user detail present at the |
Declare an array in the BO class to store the user details
In the main method, create an array of user instances/objects and call the above methods.
Refer sample input and output for formatting specifications.
Sample Input and Output 1:
Enter the number of users:
2
Enter name:
sujatha
Enter mobile number:
8909012345
Enter the username:
sujauser
Enter the password:
qwerty
Enter name:
abinaya
Enter mobile number:
8000543250
Enter the username:
abi123
Enter the password:
passabinaya
MENU:
1.To sort and display the user details.
2.To remove the user detail.
Enter your choice:
1
User details after Sorting
Name Mobilenumber Username Password
abinaya 8000543250 abi123 passabinaya
sujatha 8909012345 sujauser qwerty
Do you wish to continue(y/n):
y
MENU:
1.To sort and display the user details.
2.To remove the user detail.
Enter your choice:
2
Enter the name to be deleted:
abinaya
Deleted successfully
Do you wish to continue(y/n):
n
Sample Input and Output 2:
Enter the number of users:
3
Enter name:
usha
Enter mobile number:
9078904561
Enter the username:
usha12345
Enter the password:
ushashankar
Enter name:
aanand
Enter mobile number:
9854432216
Enter the username:
aan123aan
Enter the password:
aanandsunrise
Enter name:
tina
Enter mobile number:
9842234456
Enter the username:
tinaforyou
Enter the password:
qwertypass
MENU:
1.To sort and display the user details.
2.To remove the user detail.
Enter your choice:
2
Enter the name to be deleted:
tina
Deleted successfully
Do you wish to continue(y/n):
y
MENU:
1.To sort and display the user details.
2.To remove the user detail.
Enter your choice:
1
User details after Sorting
Name Mobilenumber Username Password
aanand 9854432216 aan123aan aanandsunrise
usha 9078904561 usha12345 ushashankar
Do you wish to continue(y/n):
n
2
Enter name:
siva
Enter mobile number:
9870056700
Enter the username:
siva123
Enter the password:
sivakumar
Enter name:
krishna
Enter mobile number:
8900989011
Enter the username:
krish_na
Enter the password:
krish1234
MENU:
1.To sort and display the user details.
2.To remove the user detail.
Enter your choice:
1
User details after Sorting
Name Mobilenumber Username Password
krishna 8900989011 krish_na krish1234
siva 9870056700 siva123 sivakumar
Do you wish to continue(y/n):
n
2
Enter name:
kumar
Enter mobile number:
9879090867
Enter the username:
kumar_123_user
Enter the password:
kitkat
Enter name:
joey
Enter mobile number:
7012588700
Enter the username:
user_joey
Enter the password:
joey1990
MENU:
1.To sort and display the user details.
2.To remove the user detail.
Enter your choice:
2
Enter the name to be deleted:
joey
Deleted successfully
Do you wish to continue(y/n):
n

Step by step
Solved in 4 steps with 2 images

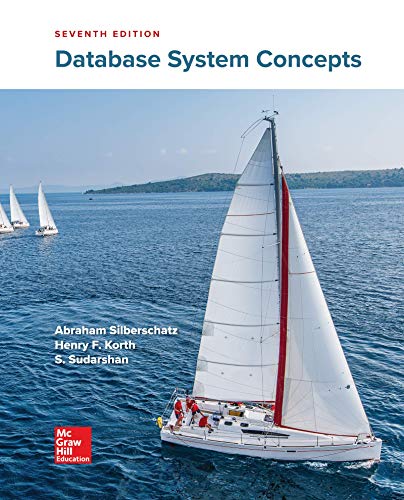
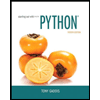
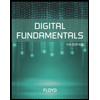
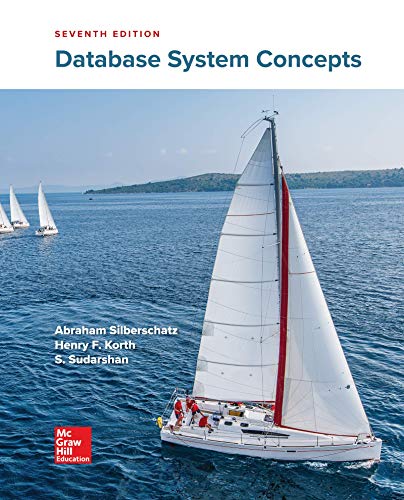
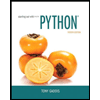
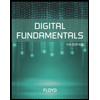
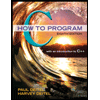
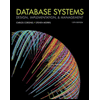
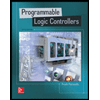