Method main: Print the message "Math Quiz". Call method display_menu and assign the return value which is the difficulty level, to a variable. Initialize a variable that will hold the number of correct answers given by the user to 0 (zero). Write a for-loop that will iterate three times. Inside this for-loop block is the following code: Call method generate_random passing it the difficulty level and assign the return value which is a random integer to a variable. This is the first number. Call method generate_random again passing it the difficulty level and assign the return value which is a random integer to a second variable. This is the second number. Print the message "Please calculate the following:". Prompt the user for what number1 plus number2 is, gather the user's response (answer) as an int and assign it to a variable. Write an if-statement that determines if the user's response is the correct answer of number1 plus number2. If so, increment the correct answer count variable. Print the difficulty level and the number of correct answers. Method display_menu: This method does not receive any parameters. It returns the difficulty level as an integer. Initialize any necessary variables for the following loop. Write a user input validation loop that does the following: Print the menu as follows: "Difficulty Levels are:" "1 - Easy" "2 = Intermediate" "3 = Hard" Prompt the user for the difficulty level, gather the user's response (answer) as an int and assign it to a variable. Write an if-statement that checks if the user entered 1, 2 or 3. If they did not, then print the following message "Please enter a difficulty level 1, 2 or 3", loop and prompt the user again until a valid response is entered. Return the user's response which is the integer representing the difficulty level. This return value is the difficulty level that is assigned to a variable in the main method. Method generate_random: This method receives the difficulty level as an integer parameter. It returns a random integer. Write an if-elif-else statement that checks if the difficulty level is 1 (one). If so, then generate a random integer between 1 (one) and 9 (nine) inclusive. Else if the difficulty level is 2 (two), then generate a random integer between 10 (ten) and 99 (ninety-nine) inclusive. Else generate a random integer between 100 (one hundred) and 999. Return the random integer. This return value is assigned to the number1 and number2 variables in the main method. Call the main method.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
I'm trying to create this menu-driven modular program in python. I'm quite inexperienced with python and would appcreciate the assistance in creating it!
Both images attached contain information on how it should be done. One being pseudo code and the other being a sample output.
Thank you!
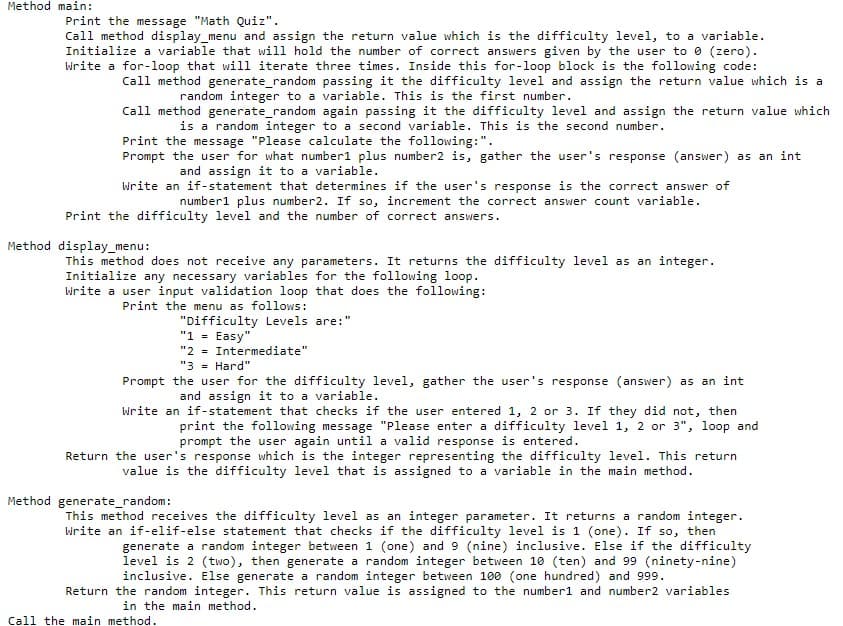
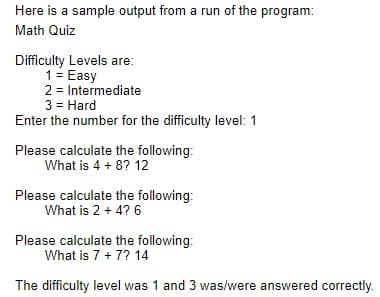

Step by step
Solved in 3 steps with 3 images

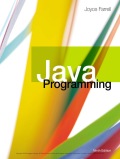
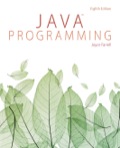
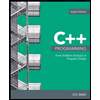
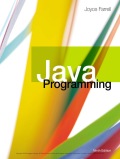
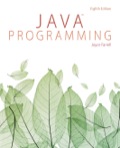
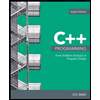
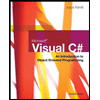