You are responsible for adding the appropriate testing code to your main. a. The getValedictorian method returns the valedictorian of the class, that is the student with the highest grade point average. Implement this new method. b. The getHonorsPercent method returns the percentage of students in the class who are in the honors program. Use 3.75 as the cutoff GPA. Implement this method. Also print the list of students, by using the isHonor method, in the Honors program. c. An alternate implementation for the School class is being considered, in which the students are stored in an ArrayList instead of an array. Show how the declaration of the data field students would look in this alternate design. MAIN CLASS: import java.util.ArrayList; public class Main { public static void main(String[] args) { System.out.println("Unit07Project2 by Your Name"); System.out.println(); int size = 10; School onw = new School(size); /* Add the code necessary to test the project here. */ } } class School { private ArrayList students; //private Student[] students; private int size; public School (int s) { size = s; students = new ArrayList(); addData(); } public void addData() { String[] names = {"Tom", "Ann", "Bob", "Jan", "Joe", "Sue", "Jay", "Meg", "Art", "Deb"}; int[] ages = {21, 34, 18, 45, 27, 19, 30, 38, 40, 35}; double[] gpas = {1.685, 3.875, 2.5, 4.0, 2.975, 3.225, 3.65, 2.0, 3.999, 2.125}; for(int i = 0; i < size; i++) { Student stu = new Student(names[i], ages[i], gpas[i]); students.add(stu); } } //returns the valedictorian of the class public Student getValidictorian() { } //return the percentage of students in the honors program public double getHonorsPercentage() { } // returns true if the student is in the honors program public boolean isHonors() { } //sort by name public void bubbleSort() { int n = size - 1; for (int p = 1; p <= n; p++) { for(int q = 0; q <= n - p; q++ ) { if(students.get(q).getName().compareTo(students.get(q+1).getName()) > 0) { Student temp = students.get(q); students.set(q, students.get(q+1)); students.set(q+1, temp); } } } } //sort by age public void selectionSort() { int min; for(int i = 0; i < size; i++) { min = i; for(int k = i + 1; k < size; k++) { if(students.get(k).getAge() < students.get(min).getAge()) { min = k; } } Student temp = students.get(i); students.set(i, students.get(min)); students.set(min, temp); } } public void insertionSort() { int n = size-1; for(int i = 0; i <= n; i++) { Student temp = students.get(i); double tempGPA = temp.getGPA(); int pos = i + 1; int index = 0; while (index < pos && tempGPA > students.get(index).getGPA()) { index++; } for(int j = pos - 1; j > index; j--) { students.set(j, students.get(j - 1)); } students.set(index, temp); } } public String toString() { String stu = ""; for(Student student : students) { stu = stu + student.toString(); } return stu; } } STUDENT CLASS: // Student.java // Used by the Lab06 and Lab07 Assignments as well public class Student { private String name; private int age; private double gpa; public Student (String n, int a, double g) { name = n; age = a; gpa = g; } public String getName() { return name; } public int getAge() { return age; } public double getGPA() { return gpa; } public String toString() { String temp = name + " " + age + " " + gpa + "\n"; return temp; } }
You are responsible for adding the appropriate testing code to your main.
a. The getValedictorian method returns the valedictorian of the class, that is the student with the highest grade point average. Implement this new method.
b. The getHonorsPercent method returns the percentage of students in the class who are in the honors program. Use 3.75 as the cutoff GPA. Implement this method. Also print the list of students, by using the isHonor method, in the Honors program.
c. An alternate implementation for the School class is being considered, in which the students are stored in an ArrayList instead of an array. Show how the declaration of the data field students would look in this alternate design.
MAIN CLASS:
STUDENT CLASS:

The JAVA code is given below with output screenshot
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

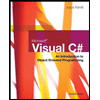
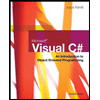