Modify the class Song you created for part 2 by doing the following: (a) Add a default constructor that initializes the song to have invalid as title and artist, and −1 as recording year. (b) Add a constructor Song(title) that initializes the song to the given title (that is, to the title given as argument). The artist and recording year are initialized to unknown and −1, respectively. The argument is a string. (c) Add a constructor Song(title, artist, year) that initializes the song to the given title, artist and recording year. The first two arguments are strings, the third one is an integer. (d) Replace the function equals(s1, s2) by a method equals(other) that returns true if the receiver and the argument have the same title, artist and recording year. (e) Add a method less_than(other) that returns true if the title of the first song is less than the title of the second song. In case the titles of the two songs are identical, the method returns true if the artist of the first song is less than the artist of the second song. In case the titles and artists of the two songs are identical, the method returns true if the first song was recorded before the second one. In all other 1 cases, the method returns false. Titles and artists are compared with respect to alphabetical order. (You can use < on strings for that purpose.) (f) Add methods title(), artist() and year() that return, respectively, the title, artist and recording year of the song. The first two functions return a string by constant reference. The third one returns an integer. Once again, you’ll need to decide how arguments should be passed to the methods. Declare methods to be constant, as appropriate. Use initializers and delegating constructors, as appropriate. Organize your class as discussed in Section 2.1 of the notes and declare methods to be inline, as appropriate. Write (and submit) a test driver for your class. Make sure you test every method My code from part 2: #include #include class Song { private: std::string title; std::string artist; int year; public: // Constructor Song(std::string t, std::string a, int y) : title(t), artist(a), year(y) {} // Methods void print(std::ostream &out = std::cout) const { out << title << std::endl << artist << std::endl << year << std::endl; } void read(std::istream &in = std::cin) { std::getline(in, title); std::getline(in, artist); in >> year; in.ignore(); } // Overloading == operator bool operator==(const Song &other) const { return title == other.title && artist == other.artist && year == other.year; } }; int main() { Song song1("title1", "artist1", 2000); Song song2("title2", "artist2", 2010); Song song3("demo1", "demo1", 0000); // Testing print method song1.print(); std::cout << std::endl; song2.print(); std::cout << std::endl; // Testing read method song3.read(); // song3.print(); std::cout << "\n" << std::endl; // Testing == operator if (song1 == song3) { std::cout << "song1 and song3 are equal" << std::endl; } else { std::cout << "song1 and song3 are not equal" << std::endl; } if (song1 == song2) { std::cout << "song1 and song2 are equal" << std::endl; } else { std::cout << "song1 and song2 are not equal" << std::endl; } return 0; }
Can you help me with this code for C++ please:
(100%) Modify the class Song you created for part 2 by
doing the following:
(a) Add a default constructor that initializes the song to have invalid as
title and artist, and −1 as recording year.
(b) Add a constructor Song(title) that initializes the song to the
given title (that is, to the title given as argument). The artist and
recording year are initialized to unknown and −1, respectively. The
argument is a string.
(c) Add a constructor Song(title, artist, year) that initializes
the song to the given title, artist and recording year. The first two
arguments are strings, the third one is an integer.
(d) Replace the function equals(s1, s2) by a method
equals(other) that returns true if the receiver and the
argument have the same title, artist and recording year.
(e) Add a method less_than(other) that returns true if the title of
the first song is less than the title of the second song. In case the titles
of the two songs are identical, the method returns true if the artist
of the first song is less than the artist of the second song. In case the
titles and artists of the two songs are identical, the method returns
true if the first song was recorded before the second one. In all other
1
cases, the method returns false. Titles and artists are compared
with respect to alphabetical order. (You can use < on strings for that
purpose.)
(f) Add methods title(), artist() and year() that return, respectively, the title, artist and recording year of the song. The first
two functions return a string by constant reference. The third one
returns an integer.
Once again, you’ll need to decide how arguments should be passed to the
methods.
Declare methods to be constant, as appropriate. Use initializers and delegating constructors, as appropriate.
Organize your class as discussed in Section 2.1 of the notes and declare
methods to be inline, as appropriate.
Write (and submit) a test driver for your class. Make sure you test every
method
My code from part 2:
#include <iostream>
#include <string>
class Song {
private:
std::string title;
std::string artist;
int year;
public:
// Constructor
Song(std::string t, std::string a, int y) : title(t), artist(a), year(y) {}
// Methods
void print(std::ostream &out = std::cout) const {
out << title << std::endl << artist << std::endl << year << std::endl;
}
void read(std::istream &in = std::cin) {
std::getline(in, title);
std::getline(in, artist);
in >> year;
in.ignore();
}
// Overloading == operator
bool operator==(const Song &other) const {
return title == other.title && artist == other.artist && year == other.year;
}
};
int main() {
Song song1("title1", "artist1", 2000);
Song song2("title2", "artist2", 2010);
Song song3("demo1", "demo1", 0000);
// Testing print method
song1.print();
std::cout << std::endl;
song2.print();
std::cout << std::endl;
// Testing read method
song3.read();
// song3.print();
std::cout << "\n" << std::endl;
// Testing == operator
if (song1 == song3) {
std::cout << "song1 and song3 are equal" << std::endl;
} else {
std::cout << "song1 and song3 are not equal" << std::endl;
}
if (song1 == song2) {
std::cout << "song1 and song2 are equal" << std::endl;
} else {
std::cout << "song1 and song2 are not equal" << std::endl;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

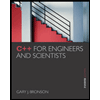
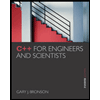