mpare and contrast the array and arrayList. Give at least one example that describe when to use arrayList compared to an array. package javaSvanos; import java.util.Scanner; public class module3project { public static void main(String args[]) { String name; double weight; int height_feet; int height_inch;
I need to add 7 and 8 to my code below....
- Compare and contrast the array and arrayList.
- Give at least one example that describe when to use arrayList compared to an array.
package javaSvanos;
import java.util.Scanner;
public class module3project {
public static void main(String args[])
{
String name;
double weight;
int height_feet;
int height_inch;
String birth_date;
try (Scanner sc = new Scanner(System.in)) {
try
{
System.out.println("Hello! Please enter the name of the patient:\t");
name = sc.nextLine();
System.out.println("Please enter the weight of the patient (in lbs):\t");
weight = sc.nextInt();
System.out.println("Please enter the height of the patient (feet):\t");
height_feet = sc.nextInt();
System.out.println("Please enter the height of the patient (inches):\t");
height_inch = sc.nextInt();
sc.nextLine();
System.out.println("Please enter the patient's birthdate:\t");
birth_date = sc.nextLine();
int height = height_feet * 12 + height_inch;
double bmi = Math.round(((weight * 703)/(height * height))*10)/10;
double lbsweight=weight*2.20462262;
System.out.println("Patient's Name:\t" + name);
System.out.println("Patient's Weight:\t" + weight + " lbs");
System.out.println("Patient's Height:\t" + height_feet + " feets " + height_inch + " inches ");
System.out.println("Patient's Birth Date:\t" + birth_date);
if(bmi < 18.5)
{
System.out.println("Patient's BMI category is underweight and the insurance payment category is low.");
}
else if(bmi >= 18.5 && bmi < 24.9)
{
System.out.println("Patient's BMI Category is Normal and insurance payment category is low.");
}
else if(bmi >= 25 && bmi <= 29.9)
{
System.out.println("BMI Category is Overweight and insurance payment category is high.");
}
else
{
System.out.println("BMI Category is Obesity and insurance payment category is highest.");
}
}
catch(Exception e)
{
System.out.println(e);
}
finally
{
System.out.println("Thank You for using the BMI Insurance Category application!");
}
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

here are the exact instructions for this project;:
You are working as a software developer for a large insurance company. Your company is planning to migrate the existing systems from Visual Basic to Java and this will require new calculations. You will be creating a program that calculates the insurance payment category based on the BMI score.
Your Java program should perform the following things:
- Take the input from the user about the patient name, weight, birthdate, and height.
- Calculate Body Mass Index.
- Display person name and BMI Category.
- If the BMI Score is less than 18.5, then underweight.
- If the BMI Score is between 18.5-24.9, then Normal.
- If the BMI score is between 25 to 29.9, then Overweight.
- If the BMI score is greater than 29.9, then Obesity.
- Calculate Insurance Payment Category based on BMI Category.
- If underweight, then insurance payment category is low.
- If Normal weight, then insurance payment category is low.
- If Overweight, then insurance payment category is high.
- If Obesity, then insurance payment category is highest.
- Implement exception handling.
- Store all the information in the file. You need to use the loop and keep asking users to enter patient name, height, weight, and birthdate. Your program should calculate BMI Category and Insurance payment category and write it to the file. Your program should stop once user enter q character.
- Create an interface called as the patient and then implement the interface as well as all the above methods.
- Store all the patient name in the queue data structure using the enqueue method and use the dequeue to remove patient name from the queue data structure and print it. You should notice the first in first out concept.
And what I have so far is:
package javaSvanos;
import java.util.Scanner;
public class module3project {
public static void main(String args[])
{
String name;
double weight;
int height_feet;
int height_inch;
String birth_date;
try (Scanner sc = new Scanner(System.in)) {
try
{
System.out.println("Hello! Please enter the name of the patient:\t");
name = sc.nextLine();
System.out.println("Please enter the weight of the patient (in lbs):\t");
weight = sc.nextInt();
System.out.println("Please enter the height of the patient (feet):\t");
height_feet = sc.nextInt();
System.out.println("Please enter the height of the patient (inches):\t");
height_inch = sc.nextInt();
sc.nextLine();
System.out.println("Please enter the patient's birthdate:\t");
birth_date = sc.nextLine();
int height = height_feet * 12 + height_inch;
double bmi = Math.round(((weight * 703)/(height * height))*10)/10;
double lbsweight=weight*2.20462262;
System.out.println("Patient's Name:\t" + name);
System.out.println("Patient's Weight:\t" + weight + " lbs");
System.out.println("Patient's Height:\t" + height_feet + " feets " + height_inch + " inches ");
System.out.println("Patient's Birth Date:\t" + birth_date);
if(bmi < 18.5)
{
System.out.println("Patient's BMI category is underweight and the insurance payment category is low.");
}
else if(bmi >= 18.5 && bmi < 24.9)
{
System.out.println("Patient's BMI Category is Normal and insurance payment category is low.");
}
else if(bmi >= 25 && bmi <= 29.9)
{
System.out.println("BMI Category is Overweight and insurance payment category is high.");
}
else
{
System.out.println("BMI Category is Obesity and insurance payment category is highest.");
}
}
catch(Exception e)
{
System.out.println(e);
}
finally
{
System.out.println("Thank You for using the BMI Insurance Category application!");
}
}
}
}
And this was the teachers notes and feedback for this assignment if that helps.
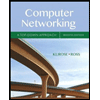
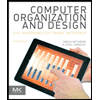
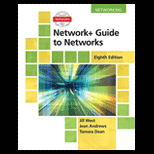
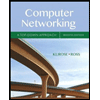
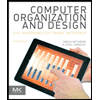
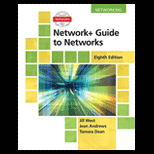
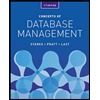
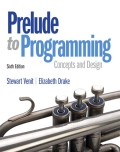
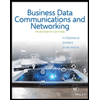