mplement the undo/redo functionalities through stacks by modifying the given code in C Language: #include #include #include struct node{ char data[100]; struct node* next; }; int elements = 0; // function for add values to stack struct node* add(struct node* stack, char *str){ struct node* tp = (struct node*)malloc(sizeof(struct node)) ; strcpy(tp->data,str); tp->next = stack; elements++; return tp; } //redo function void redo(){ elements++; } //undo function void undo(){ elements--; } //print function int print(struct node* stack,int idx){ if(stack == NULL) return 0; idx = print(stack->next,idx); if(idxdata); return idx+1; } //function for save in file int save_command(FILE* filePointer, struct node* stack,int idx) { if(stack == NULL){ return 0; } idx = save_command(filePointer, stack->next,idx); if(idxdata); return idx+1; } //main function int main(){ //stacks for undo and redo struct node *stack1 = NULL; char input[100]; while(1==1){ printf("MyCommand > "); gets(input); if(strcmp("undo",input)==0){ //if input is undo undo(); printf("result >\n"); print(stack1,elements); } else if(strcmp("redo",input)==0){ //if input is redo redo(); printf("result >\n"); print(stack1,elements); } else if(strcmp("print",input)==0){ //if input is print printf("result >\n"); print(stack1,elements); } else if(strcmp("save", input)==0){ //if input is save FILE* filePointer; filePointer=fopen("output.txt","w"); save_command(filePointer,stack1,elements); fclose(filePointer); } else if(strcmp("quit",input)==0) //if input is quit { FILE* filePointer; filePointer=fopen("output.txt","w"); save_command(filePointer,stack1,elements); fclose(filePointer); printf("result > Good Bye!\n"); exit(0); } else //if input is any other string add to stack stack1 = add(stack1,input); } return 0; }
Implement the undo/redo functionalities through stacks by modifying the given code in C Language:
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
struct node{
char data[100];
struct node* next;
};
int elements = 0;
// function for add values to stack
struct node* add(struct node* stack, char *str){
struct node* tp = (struct node*)malloc(sizeof(struct node)) ;
strcpy(tp->data,str);
tp->next = stack;
elements++;
return tp;
}
//redo function
void redo(){
elements++;
}
//undo function
void undo(){
elements--;
}
//print function
int print(struct node* stack,int idx){
if(stack == NULL)
return 0;
idx = print(stack->next,idx);
if(idx<elements)
printf("%s\n",stack->data);
return idx+1;
}
//function for save in file
int save_command(FILE* filePointer, struct node* stack,int idx)
{
if(stack == NULL){
return 0;
}
idx = save_command(filePointer, stack->next,idx);
if(idx<elements)
fprintf(filePointer,"%s\n",stack->data);
return idx+1;
}
//main function
int main(){
//stacks for undo and redo
struct node *stack1 = NULL;
char input[100];
while(1==1){
printf("MyCommand > ");
gets(input);
if(strcmp("undo",input)==0){ //if input is undo
undo();
printf("result >\n"); print(stack1,elements);
}
else if(strcmp("redo",input)==0){ //if input is redo
redo();
printf("result >\n"); print(stack1,elements);
}
else if(strcmp("print",input)==0){ //if input is print
printf("result >\n");
print(stack1,elements);
}
else if(strcmp("save", input)==0){ //if input is save
FILE* filePointer;
filePointer=fopen("output.txt","w");
save_command(filePointer,stack1,elements);
fclose(filePointer);
}
else if(strcmp("quit",input)==0) //if input is quit
{
FILE* filePointer;
filePointer=fopen("output.txt","w");
save_command(filePointer,stack1,elements);
fclose(filePointer);
printf("result > Good Bye!\n");
exit(0);
}
else //if input is any other string add to stack
stack1 = add(stack1,input);
}
return 0;
}

Step by step
Solved in 2 steps

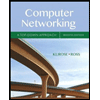
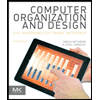
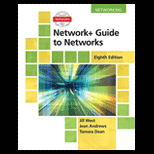
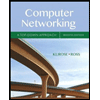
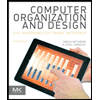
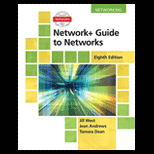
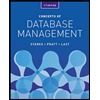
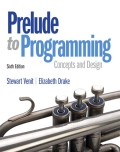
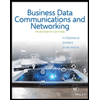