C++ Programming Activity: Linked List Stack and Brackets Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow #include "stack.h" #include "linkedlist.h" // SLLStack means Singly Linked List (SLL) Stack class SLLStack : public Stack { LinkedList* list; public: SLLStack() { list = new LinkedList(); } void push(char e) { list->add(e); return; } char pop() { char elem; elem = list->removeTail(); return elem; } char top() { char elem; elem = list->get(size()); return elem; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } };
C++ Programming Activity: Linked List Stack and Brackets Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow #include "stack.h" #include "linkedlist.h" // SLLStack means Singly Linked List (SLL) Stack class SLLStack : public Stack { LinkedList* list; public: SLLStack() { list = new LinkedList(); } void push(char e) { list->add(e); return; } char pop() { char elem; elem = list->removeTail(); return elem; } char top() { char elem; elem = list->get(size()); return elem; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } };
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question
C++ Programming
Activity: Linked List Stack and Brackets
Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow
#include "stack.h"
#include "linkedlist.h"
// SLLStack means Singly Linked List (SLL) Stack
class SLLStack : public Stack {
LinkedList* list;
public:
SLLStack() {
list = new LinkedList();
}
void push(char e) {
list->add(e);
return;
}
char pop() {
char elem;
elem = list->removeTail();
return elem;
}
char top() {
char elem;
elem = list->get(size());
return elem;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
};
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
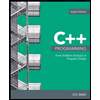
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
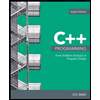
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning