My homework This program reads a number of up to 9 digits. It then prints the number in English. In other words, if you run the program and enter 12345678, it should respond with "one hundred twenty three million four hundred fifty six thousand seven hundred eighty nine". If you enter 10000, it ought to print "ten thousand". This program is intended to give you practice using the switch statement and variable parameters in functions. C++ just only use and SWITCH statement. Thank you so much And my this code, help me fix it. #include using namespace std; //Read number one digit void one(int x) { switch(x){ case 1: cout<<"-one"; break; case 2: cout<<"-two"; break; case 3: cout<<"-three"; break; case 4: cout<<"-four"; break; case 5: cout<<"-five"; break; case 6: cout<<"-six"; break; case 7: cout<<"-seven"; break; case 8: cout<<"eight"; break; case 9: cout<<"-nine"; break; } } void digit2(int x) { switch(x) { case 0: cout<<"ten"; break; case 1: cout<<"Eleven"; break; case 2: cout<<"Twelve"; break; case 3: cout<<"Thirteen"; break; case 4: cout<<"Fourteen"; break; case 5: cout<<"Fifteen"; break; case 6: cout<<"Sixteen"; break; case 7: cout<<"Seventeen"; break; case 8: cout<<"Eighteen"; break; case 9: cout<<"Nineteen"; break; } } //Read ten number void ten(int x) { switch(x){ case 2: cout<<"twenty"; break; case 3: cout<<"thirty"; break; case 4: cout<<"forty"; break; case 5: cout<<"fifty"; break; case 6: cout<<"sixty"; break; case 7: cout<<"seventy"; break; case 8: cout<<"eighty"; break; case 9: cout<<"ninety"; break; } } //Read number hundred void hundred(int x) { one(x); cout<<" hundred"; } //Read a thousand number void thousand(int x) { one(x); cout<<" thousand"; } //Read a ten thousand number void tensthousand(int x) { ten(x); cout<<" thousand"; } //Read a number tens of thousands void tenshundredthousands(int x) { one(x); cout<<" hundred thousand"; } //Read a number million void million(int x) { one(x); cout<<" million"; } //Read a number ten million void tenmillion(int x) { ten(x); cout<<" million"; } //Read a number hundred million void hundredmillion(int x) { one(x); cout<<" hundred million"; } int main() { int n; char a, b, c, d, e, f, g, h, t, j; cout<<"Input a 9 digit positive: "; cin>>n; //Separate numbers in millions, hundreds of thousands, tens of thousands, thousands, hundreds, tens, and units a = n%10; b = (n/10)%10; c = (n/100)%10; d = (n/1000)%10; e = (n/10000)%10; f = (n/100000)%10; g = (n/1000000)%10; h = (n/10000000)%10; t = (n/100000000)%10; // Read the numbers just split above if(n<10) { one(a); cout<=10 && n<100) { ten(b); cout<=100 && n<1000) { hundred(c); cout<=1000 && n<10000) { thousand(d); cout<=10000 && n<100000) { tensthousand(f); cout<=100000 && n<1000000) { tenshundredthousands(g); cout<=1000000 && n<10000000) { million(h); cout<=10000000 && n<100000000) { tenmillion(f); cout<
My homework
This program reads a number of up to 9 digits. It then prints the number in English. In other words, if you run the program and enter 12345678, it should respond with "one hundred twenty three million four hundred fifty six thousand seven hundred eighty nine". If you enter 10000, it ought to print "ten thousand". This program is intended to give you practice using the switch statement and variable parameters in functions.
C++ just only use <iostream> and SWITCH statement. Thank you so much
And my this code, help me fix it.
#include <iostream>
using namespace std;
//Read number one digit
void one(int x)
{
switch(x){
case 1:
cout<<"-one";
break;
case 2:
cout<<"-two";
break;
case 3:
cout<<"-three";
break;
case 4:
cout<<"-four";
break;
case 5:
cout<<"-five";
break;
case 6:
cout<<"-six";
break;
case 7:
cout<<"-seven";
break;
case 8:
cout<<"eight";
break;
case 9:
cout<<"-nine";
break;
}
}
void digit2(int x)
{
switch(x)
{
case 0:
cout<<"ten";
break;
case 1:
cout<<"Eleven";
break;
case 2:
cout<<"Twelve";
break;
case 3:
cout<<"Thirteen";
break;
case 4:
cout<<"Fourteen";
break;
case 5:
cout<<"Fifteen";
break;
case 6:
cout<<"Sixteen";
break;
case 7:
cout<<"Seventeen";
break;
case 8:
cout<<"Eighteen";
break;
case 9:
cout<<"Nineteen";
break;
}
}
//Read ten number
void ten(int x)
{
switch(x){
case 2:
cout<<"twenty";
break;
case 3:
cout<<"thirty";
break;
case 4:
cout<<"forty";
break;
case 5:
cout<<"fifty";
break;
case 6:
cout<<"sixty";
break;
case 7:
cout<<"seventy";
break;
case 8:
cout<<"eighty";
break;
case 9:
cout<<"ninety";
break;
}
}
//Read number hundred
void hundred(int x)
{
one(x);
cout<<" hundred";
}
//Read a thousand number
void thousand(int x)
{
one(x);
cout<<" thousand";
}
//Read a ten thousand number
void tensthousand(int x)
{
ten(x);
cout<<" thousand";
}
//Read a number tens of thousands
void tenshundredthousands(int x)
{
one(x);
cout<<" hundred thousand";
}
//Read a number million
void million(int x)
{
one(x);
cout<<" million";
}
//Read a number ten million
void tenmillion(int x)
{
ten(x);
cout<<" million";
}
//Read a number hundred million
void hundredmillion(int x)
{
one(x);
cout<<" hundred million";
}
int main()
{
int n;
char a, b, c, d, e, f, g, h, t, j;
cout<<"Input a 9 digit positive: ";
cin>>n;
//Separate numbers in millions, hundreds of thousands, tens of thousands, thousands, hundreds, tens, and units
a = n%10;
b = (n/10)%10;
c = (n/100)%10;
d = (n/1000)%10;
e = (n/10000)%10;
f = (n/100000)%10;
g = (n/1000000)%10;
h = (n/10000000)%10;
t = (n/100000000)%10;
// Read the numbers just split above
if(n<10)
{
one(a);
cout<<a<<endl;
}
else if(n>=10 && n<100)
{
ten(b);
cout<<b<<endl;
}
else if(n>=100 && n<1000)
{
hundred(c);
cout<<c<<endl;
}
else if(n>=1000 && n<10000)
{
thousand(d);
cout<<d<<endl;
}
else if(n>=10000 && n<100000)
{
tensthousand(f);
cout<<f<<endl;
}
else if(n>=100000 && n<1000000)
{
tenshundredthousands(g);
cout<<g<<endl;
}
else if(n>=1000000 && n<10000000)
{
million(h);
cout<<h<<endl;
}
else if(n>=10000000 && n<100000000)
{
tenmillion(f);
cout<<f<<endl;
}
else
{
hundredmillion(t);
cout<<t<<endl;
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

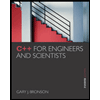
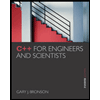