need this in C++ Let’s assume that we work for a company that has been in operation for a number of years. Information regarding company sales has been maintained over the years. One of the files contains sales figures for each quarter of a year. The company has 10 branches, I am providing for you a text that contains sales amounts for each quarter of the year for each of the 10 branches. The contents of the text look something like this: 45374 28632 39744 14521 23008 10621 29439 14639 36284 15901 16774 47521 10292 12226 37623 40141 35628 32840 43037 41682 34371 10317 49328 14117 46777 31706 19771 32610 34077 30228 32118 18654 40335 25232 42942 48181 18642 14075 45192 42640 The first 4 numbers represent the sales for the 4 quarters for the first branch of the company. The next 4 numbers represent the sales for the 4 quarters for the second branch of the company , and so on. Your program will read in the data from the file and store that data in a 2-dimensional array. Your program will then display a menu from which the user can make selections regarding the data contained in the array. Your program must contain the following items: Pre-conditions and post-conditions clearly stated for all functions. Thorough inline documentation for all code. The following variables: A 2-Dimensional array which must be declared in function main() that will store the sales data for the company. Global variables are not permitted. (You may declare global constants) The following functions (you need to determine return types and necessary parameters and whether they are value or reference parameters): main – will drive your application readFile – will read the data from a file and store the data in a 2-Dimensional array. displayMenu – will display a menu from which the user can make selections. The user will be prompted to enter a selection. The selection made by the user will invoke an appropriate function that provides the necessary processing. In addition you will need individual functions that provide the functionality required by each of the menu items. Choose 1 to see a branch’s sales for a particular quarter Choose 2 to see the total sales for a branch. Choose 3 to see the total branch sales for a particular quarter Choose 4 to see the total sales for all branches Choose 5 to see the sales for the highest quarter for a branch Choose 6 to see the sales for the lowest quarter for a branch Choose 7 to see the average quarterly sales for the company Choose 8 to see to see a branch's increase or decrease from a previous quarter Choose 9 to see a list of all quarterly sales figures Choose 10 to see the company's increase or decrease from the previous quarter Choose 12 to exit this program. You should create stubs for any functions not completed or not working properly. Document any enhancements you might make beyond the basic requirements. Input validation – do not accept negative values for any user input from the keyboard. In addition, make the user input more friendy by allowing the user to enter values from 1 to 4 rather than 0 to 3 for the quarters and values from 1 to 10 for rather than 0 to 9 for the branches. Make the necessary code adjustment to make this work. Your functions must be cohesive. They should focus on doing a single thing. Most functions that you create should be between 5 and 15 lines. Anything longer probably needs to be decomposed into additional functions. At a minimum, you must test your program using the below Test Run. You should also test for valid user input. Test Run Menu Choose 1 to see a branch's sales for a particular quarter Choose 2 to see the total sales for a branch. Choose 3 to see the total branch sales for a particular quarter Choose 4 to see the total sales for all branches Choose 5 to see the sales for the highest quarter for a branch Choose 6 to see the sales for the lowest quarter for a branch Choose 7 to see the average quarterly sales for the company Choose 8 to see to see a branch's increase or decrease from a previous quarter Choose 9 to see a list of all quarterly sales figures Choose 10 to see the company's increase or decrease from the previous quarter Choose 12 to exit this program. Enter your choice and press Return: 9 contents of the array Branch # Qtr 1 Qtr 2 Qtr 3 Qtr 4 Branch 1 $45374 $28632 $39744 $14521 Branch 2 $23008 $10621 $29439 $14639 Branch 3 $36284 $15901 $16774 $47521 Branch 4 $10292 $12226 $37623 $40141 Branch 5 $35628 $32840 $43037 $41682 Branch 6 $34371 $10317 $49328 $14117 Branch 7 $46777 $31706 $19771 $32610 Branch 8 $34077 $30228 $32118 $18654 Branch 9 $40335 $25232 $42942 $48181 Branch 10 $18642 $14075 $45192 $42640 Menu Choose 1 to see a branch's sales for a particular quarter Choose 2 to see the total sales for a branch. Choose 3 to see the total branch sales for a particular quarter the following is in the pictu
need this in C++
Let’s assume that we work for a company that has been in operation for a number of years. Information regarding company sales has been maintained over the years. One of the files contains sales figures for each quarter of a year. The company has 10 branches,
I am providing for you a text that contains sales amounts for each quarter of the year for each of the 10 branches. The contents of the text look something like this:
45374
28632
39744
14521
23008
10621
29439
14639
36284
15901
16774
47521
10292
12226
37623
40141
35628
32840
43037
41682
34371
10317
49328
14117
46777
31706
19771
32610
34077
30228
32118
18654
40335
25232
42942
48181
18642
14075
45192
42640
The first 4 numbers represent the sales for the 4 quarters for the first branch of the company. The next 4 numbers represent the sales for the 4 quarters for the second branch of the company , and so on.
Your program will read in the data from the file and store that data in a 2-dimensional array. Your program will then display a menu from which the user can make selections regarding the data contained in the array.
Your program must contain the following items:
- Pre-conditions and post-conditions clearly stated for all functions.
- Thorough inline documentation for all code.
- The following variables:
- A 2-Dimensional array which must be declared in function main() that will store the sales data for the company.
- Global variables are not permitted. (You may declare global constants)
- The following functions (you need to determine return types and necessary parameters and whether they are value or reference parameters):
- main – will drive your application
- readFile – will read the data from a file and store the data in a 2-Dimensional array.
- displayMenu – will display a menu from which the user can make selections. The user will be prompted to enter a selection. The selection made by the user will invoke an appropriate function that provides the necessary processing.
- In addition you will need individual functions that provide the functionality required by each of the menu items.
- Choose 1 to see a branch’s sales for a particular quarter
- Choose 2 to see the total sales for a branch.
- Choose 3 to see the total branch sales for a particular quarter
- Choose 4 to see the total sales for all branches
- Choose 5 to see the sales for the highest quarter for a branch
- Choose 6 to see the sales for the lowest quarter for a branch
- Choose 7 to see the average quarterly sales for the company
- Choose 8 to see to see a branch's increase or decrease from a previous quarter
- Choose 9 to see a list of all quarterly sales figures
- Choose 10 to see the company's increase or decrease from the previous quarter
- Choose 12 to exit this program.
- You should create stubs for any functions not completed or not working properly.
- Document any enhancements you might make beyond the basic requirements.
- Input validation – do not accept negative values for any user input from the keyboard. In addition, make the user input more friendy by allowing the user to enter values from 1 to 4 rather than 0 to 3 for the quarters and values from 1 to 10 for rather than 0 to 9 for the branches. Make the necessary code adjustment to make this work.
- Your functions must be cohesive. They should focus on doing a single thing. Most functions that you create should be between 5 and 15 lines. Anything longer probably needs to be decomposed into additional functions.
- At a minimum, you must test your program using the below Test Run. You should also test for valid user input.
Test Run
Menu
Choose 1 to see a branch's sales for a particular quarter
Choose 2 to see the total sales for a branch.
Choose 3 to see the total branch sales for a particular quarter
Choose 4 to see the total sales for all branches
Choose 5 to see the sales for the highest quarter for a branch
Choose 6 to see the sales for the lowest quarter for a branch
Choose 7 to see the average quarterly sales for the company
Choose 8 to see to see a branch's increase or decrease from a previous quarter
Choose 9 to see a list of all quarterly sales figures
Choose 10 to see the company's increase or decrease from the previous quarter
Choose 12 to exit this program.
Enter your choice and press Return:
9
contents of the array
Branch # Qtr 1 Qtr 2 Qtr 3 Qtr 4
Branch 1 $45374 $28632 $39744 $14521
Branch 2 $23008 $10621 $29439 $14639
Branch 3 $36284 $15901 $16774 $47521
Branch 4 $10292 $12226 $37623 $40141
Branch 5 $35628 $32840 $43037 $41682
Branch 6 $34371 $10317 $49328 $14117
Branch 7 $46777 $31706 $19771 $32610
Branch 8 $34077 $30228 $32118 $18654
Branch 9 $40335 $25232 $42942 $48181
Branch 10 $18642 $14075 $45192 $42640
Menu
Choose 1 to see a branch's sales for a particular quarter
Choose 2 to see the total sales for a branch.
Choose 3 to see the total branch sales for a particular quarter
the following is in the picture
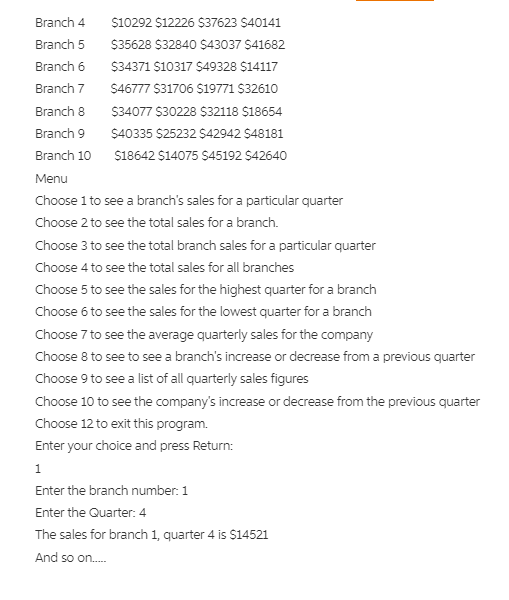

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

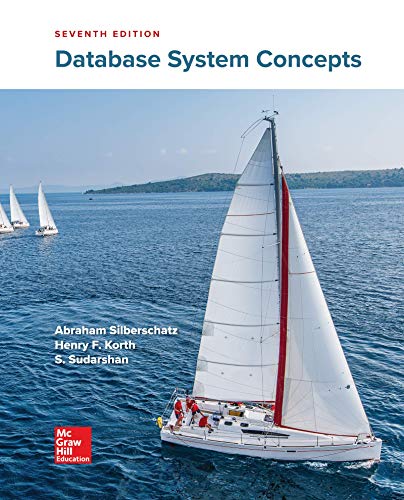
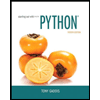
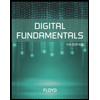
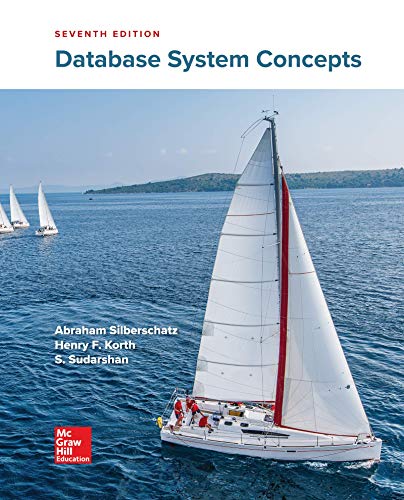
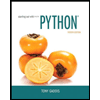
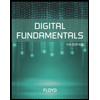
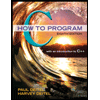
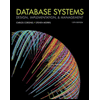
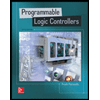