No function in your program is allowed to be longer than 10 statements. • Be sure to include the entire program, including your call to the main function, the answer box. For example: Test Input Result # This test simply runs the program, Angus Enter student's name or 'quit' if finished: Angus # so make sure you include the call to main() Bella Enter student's name or 'quit' if finished: Bella Enter student's name or 'quit' if finished: Cam Enter student's name or 'quit' if finished: Dani Enter student's name or 'quit' if finished: quit Cam Dani quit 51 Enter Angus's mark: 51 67 Enter Bella's mark: 67 78 Enter Cam's mark: 78 94 Enter Dani's mark: 94 CLASS RESULTS Angus: C- (51%) Bella: B- (67%) Cam: B+ (78%) Dani: A+ (94%)
No function in your program is allowed to be longer than 10 statements. • Be sure to include the entire program, including your call to the main function, the answer box. For example: Test Input Result # This test simply runs the program, Angus Enter student's name or 'quit' if finished: Angus # so make sure you include the call to main() Bella Enter student's name or 'quit' if finished: Bella Enter student's name or 'quit' if finished: Cam Enter student's name or 'quit' if finished: Dani Enter student's name or 'quit' if finished: quit Cam Dani quit 51 Enter Angus's mark: 51 67 Enter Bella's mark: 67 78 Enter Cam's mark: 78 94 Enter Dani's mark: 94 CLASS RESULTS Angus: C- (51%) Bella: B- (67%) Cam: B+ (78%) Dani: A+ (94%)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
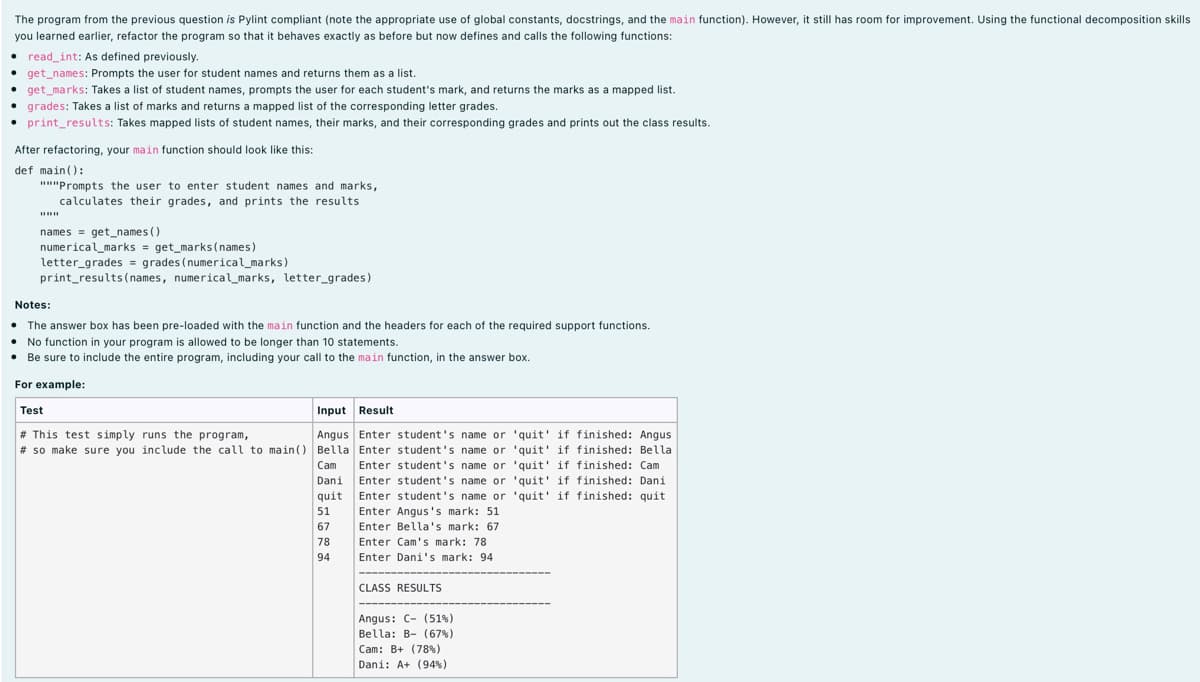
Transcribed Image Text:The program from the previous question is Pylint compliant (note the appropriate use of global constants, docstrings, and the main function). However, it still has room for improvement. Using the functional decomposition skills
you learned earlier, refactor the program so that it behaves exactly as before but now defines and calls the following functions:
read_int: As defined previously.
get_names: Prompts the user for student names and returns them as a list.
get_marks: Takes a list of student names, prompts the user for each student's mark, and returns the marks as a mapped list.
• grades: Takes a list of marks and returns a mapped list of the corresponding letter grades.
• print_results: Takes mapped lists of student names, their marks, and their corresponding grades and prints out the class results.
After refactoring, your main function should look like this:
def main():
""Prompts the user to enter student names and marks,
calculates their grades, and prints the results
names = get_names ()
numerical_marks = get_marks(names)
letter_grades = grades (numerical_marks)
print_results ( names, numerical_marks, letter_grades)
Notes:
• The answer box has been pre-loaded with the main function and the headers for each of the required support functions.
No function in your program is allowed to be longer than 10 statements.
• Be sure to include the entire program, including your call to the main function, in the answer box.
For example:
Test
Input Result
# This test simply runs the program,
# so make sure you include the call to main() Bella Enter student's name or 'quit' if finished: Bella
Angus Enter student's name or 'quit' if finished: Angus
Enter student's name or 'quit' if finished: Cam
Enter student's name or 'quit' if finished: Dani
Enter student's name or 'quit' if finished: quit
Enter Angus's mark: 51
Enter Bella's mark: 67
Cam
Dani
quit
51
67
78
Enter Cam's mark: 78
94
Enter Dani's mark: 94
CLASS RESULTS
Angus: C- (51%)
Bella: B- (67%)
Cam: B+ (78%)
Dani: A+ (94%)
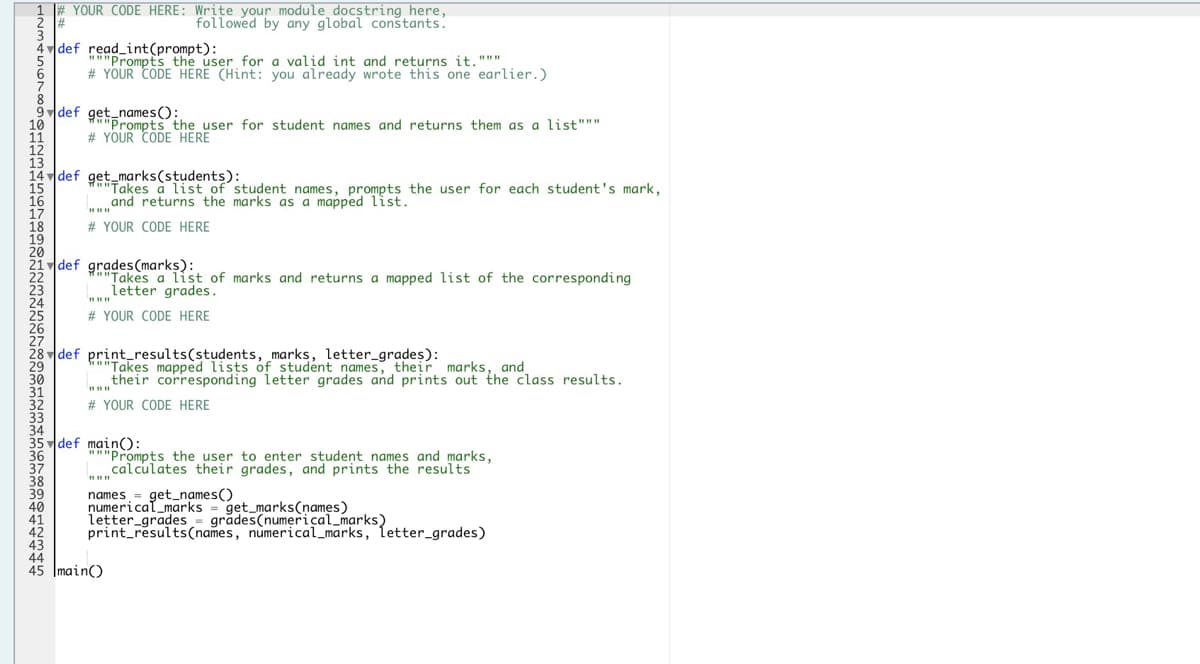
Transcribed Image Text:1 # YOUR CODE HERE: Write your module docstring here,
followed by any global constants.
4 def read_int(prompt):
*i"Prompts the user for a valid int and returns it."""
# YOUR CODE HERE CHint: you already wrote this one earlier.)
7
9 def get_names():
10
11
12
13
"Prompts the user for student names and returns them as a list"""
# YOUR CODE HERE
14 def get_marks(students):
15
16
17
18
19
"Takes a list of student names, prompts the user for each student's mark,
and returns the marks as a mapped list.
# YOUR CODE HERE
def grades(marks):
"Takes a list of marks and returns a mapped list of the corresponding
letter grades.
# YOUR CODE HERE
28 v def print_results(students, marks, letter_grades):
*"Takes mapped lists of studént names, their marks, and
their corresponding letter grades and prints out the class results.
# YOUR CODE HERE
def main():
"""Prompts the user to enter student names and marks,
calculates their grades, and prints the results
38
names = get_names()
numerical_marks = get_marks(names)
letter_grades
print_results(names, numerical_marks, letter_grades)
40
41
grădes(numerical_marks)
43
45 Imain()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
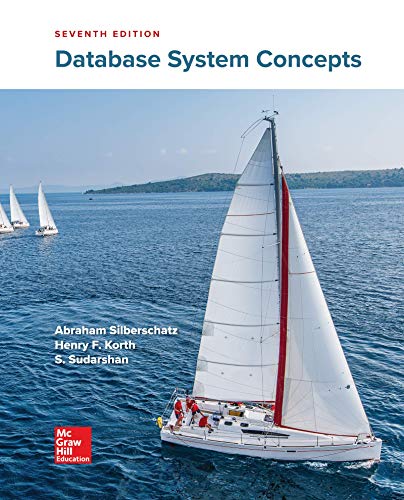
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
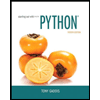
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
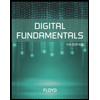
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
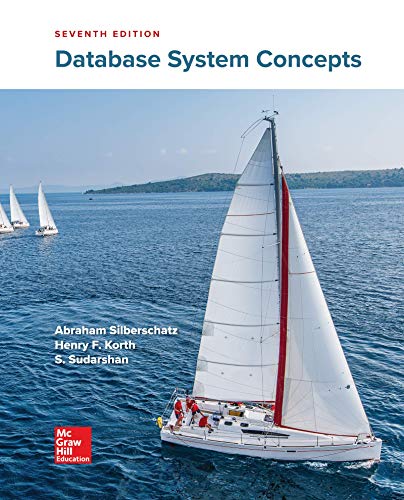
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
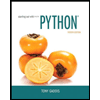
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
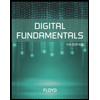
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
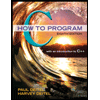
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
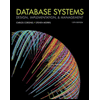
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
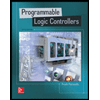
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education